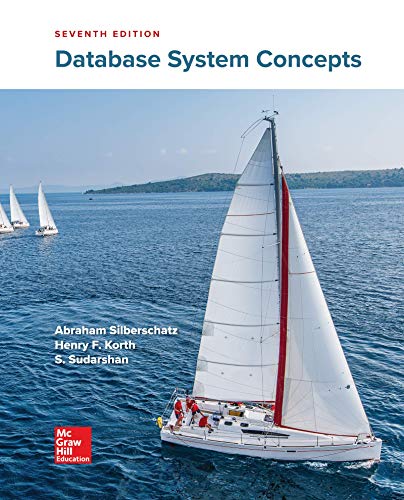
I need image part
in this program
// C++ program to create and implement FCFS Queue of customers
#include <iostream>
#include <cstring>
using namespace std;
class Customer
{
private:
char name[15];
int arrivalTime;
int serviceTime;
int finishTime;
public:
// function to set name, arrivalTime and serviceTime to specified values
void setCustomer(char *name, int arrivalTime, int serviceTime)
{
strcpy(this->name, name);
this->arrivalTime = arrivalTime;
this->serviceTime = serviceTime;
this->finishTime = arrivalTime + serviceTime; // set finishTime as sum of arrivalTime and serviceTime
}
// function to display the customer details
void displayCustomer()
{
cout<<"Name: "<<name<<", Arrival Time: "<<arrivalTime<<", Service Time: "<<serviceTime<<", Finish Time: "<<finishTime<<endl;
}
};
class FCFSQueue
{
private:
Customer customerList[100];
int length = 0;
public:
// function that returns true if queue is empty else return false
bool isEmpty()
{
return length == 0;
}
// function to return number of elements in queue
int GetLength()
{
return length;
}
// function to insert customer at end of queue
void Enqueue(Customer customer)
{
if(length < 100) // queue is not full, insert customer at index length
{
customerList[length] = customer;
length++; // increment length by 1
}
else // queue is full
cout<<"Queue is full"<<endl;
}
// function to remove the customer at the front
void Dequeue()
{
if(length > 0) // queue is not empty
{
Customer customer = customerList[0]; // get the customer at the front
// loop to shift the elements to left by 1 position from second element to end
for(int i=0;i<length-1;i++)
customerList[i] = customerList[i+1];
length--; // decrement length by 1
// display the customer removed
cout<<"Customer removed: "<<endl;
customer.displayCustomer();
}
else // queue is empty
cout<<"Queue is empty"<<endl;
}
};
int main()
{
FCFSQueue queue; // create an empty queue
Customer customer;
cout<<"Queue is empty: "<<boolalpha<<queue.isEmpty()<<endl;
cout<<"Number of customers in queue: "<<queue.GetLength()<<endl;
// add customers
cout<<"\nAdding customers"<<endl;
customer.setCustomer("Adam Hope", 5, 15);
queue.Enqueue(customer);
customer.setCustomer("Harry Johnson", 7, 18);
queue.Enqueue(customer);
customer.setCustomer("John Smith", 10, 12);
queue.Enqueue(customer);
cout<<"Queue is empty: "<<boolalpha<<queue.isEmpty()<<endl;
cout<<"Number of customers in queue: "<<queue.GetLength()<<endl;
cout<<"\nRemoving all customers:"<<endl;
// loop to remove all customers from queue
while(!queue.isEmpty())
{
queue.Dequeue();
}
cout<<"Queue is empty: "<<boolalpha<<queue.isEmpty()<<endl;
cout<<"Number of customers in queue: "<<queue.GetLength()<<endl;
return 0;
}
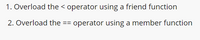

Step by stepSolved in 2 steps with 1 images

- Write a menu-driven C++ program to manage your college course history and plans, named as you wish. It should work something like this: Array size: 0, capacity: 2MENUA Add a courseL List all coursesC Arrange by courseY arrange by YearU arrange by UnitsG arrange by GradeQ Quit...your choice: a[ENTER]Enter a courses' name: Comsc-165[ENTER]Enter the year for Comsc-165 [like 2020]: 2016[ENTER]Enter the units for Comsc-165 [0, 1, 2,...]: 4[ENTER]Enter the grade for Comsc-165 [A, B,..., X if still in progress or planned]: x[ENTER]Array size: 1, capacity: 2MENUA Add a courseL List all coursesC Arrange by courseY arrange by YearU arrange by UnitsG arrange by GradeQ Quit...your choice: a[ENTER]Enter a courses' name: Comsc-110[ENTER]Enter the year for Comsc-110 [like 2020]: 2015[ENTER]Enter the units for Comsc-110 [0, 1, 2,...]: 4[ENTER]Enter the grade for Comsc-110 [A, B,..., X if still in progress or planned]: A[ENTER]Array size: 2, capacity: 2MENUA Add a courseL List all coursesC Arrange by…arrow_forwardConsider the following C++ code segment: int d = 20; int f(int b) { static int c = 0; c *= b; return c; } int main() { int a; cin >> a; cout << f(a) * d << endl; }For each variable a, b, c, d: identify all type bindings and storage bindings for each binding, determine when the binding occurs identify the scope and lifetimearrow_forwarduse replit please Thank youarrow_forward
- please help me to answerarrow_forwardneed help in C++ Problem: You are asked to create a program for storing the catalog of movies at a DVD store using functions, files, and user-defined structures. The program should let the user read the movie through the file, add, remove, and output movies to the file. For this assignment, you must store the information about the movies in the catalog using a single vector. The vector's data type is a user-defined structure that you must define on functions.h following these rules: Identifier for the user-define structure: movie. Member variables of the structure "movie": name (string), year (int), and genre (string). Note: you must use the identifiers presented before when defining the user-defined structure. Your solution will NOT pass the unit test cases if you do not follow the instructions presented above. The main function is provided (you need to modify the code of the main function to call the user-defined functions described below). The following user-defined functions are…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
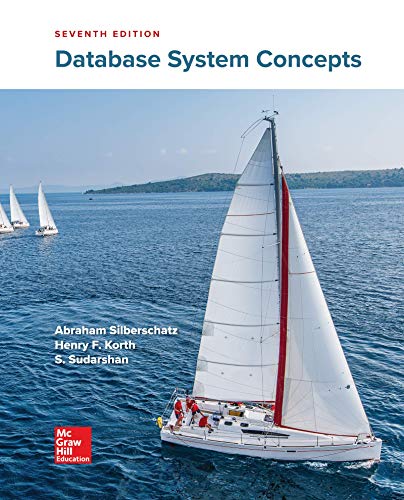
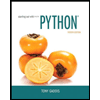
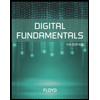
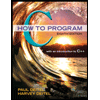
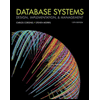
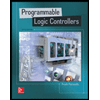