You should not make any changes to DPQueue.h. ■ You should not remove any functions from DPQueue.cpp, and you need to: ● Fill in the implementation of all the "stub" functions in the file (those with output statements containing the message "??? not implemented yet"), and ● Provide any additional documentation where appropriate. The file SuccessfulAutoTest.txt shows how the output for a test with complete success should look like. ► Referring to it should give you a purview of the number/kind of tests involved.
You should not make any changes to DPQueue.h. |
■ | You should not remove any functions from DPQueue.cpp, and you need to: |
● | Fill in the implementation of all the "stub" functions in the file (those with output statements containing the message "??? not implemented yet"), and |
● | Provide any additional documentation where appropriate. |
The file SuccessfulAutoTest.txt shows how the output for a test with complete success should look like. |
► | Referring to it should give you a purview of the number/kind of tests involved. |
#endifusing namespace std;
namespace CS3358_FA2022_A7
{
// CONSTRUCTORS AND DESTRUCTOR
p_queue::p_queue(size_type initial_capacity)
{
cerr << "p_queue() not implemented yet" << endl;
}
p_queue::p_queue(const p_queue& src)
{
cerr << "p_queue(const p_queue&) not implemented yet" << endl;
}
p_queue::~p_queue()
{
cerr << "~p_queue() not implemented yet" << endl;
}
// MODIFICATION MEMBER FUNCTIONS
p_queue& p_queue::operator=(const p_queue& rhs)
{
cerr << "operator=(const p_queue&) not implemented yet" << endl;
return *this;
}
void p_queue::push(const value_type& entry, size_type priority)
{
cerr << "push(const value_type&, size_type) not implemented yet" << endl;
}
void p_queue::pop()
{
cerr << "pop() not implemented yet" << endl;
}
// CONSTANT MEMBER FUNCTIONS
p_queue::size_type p_queue::size() const
{
cerr << "size() not implemented yet" << endl;
return 0; // dummy return value
}
bool p_queue::empty() const
{
cerr << "empty() not implemented yet" << endl;
return false; // dummy return value
}
p_queue::value_type p_queue::front() const
{
cerr << "front() not implemented yet" << endl;
return value_type(); // dummy return value
}
// PRIVATE HELPER FUNCTIONS
void p_queue::resize(size_type new_capacity)
// Pre: (none)
// Post: The size of the dynamic array pointed to by heap (thus
// the capacity of the p_queue) has been resized up or down
// to new_capacity, but never less than used (to prevent
// loss of existing data).
// NOTE: All existing items in the p_queue are preserved and
// used remains unchanged.
{
cerr << "resize(size_type) not implemented yet" << endl;
}
bool p_queue::is_leaf(size_type i) const
// Pre: (i < used)
// Post: If the item at heap[i] has no children, true has been
// returned, otherwise false has been returned.
{
cerr << "is_leaf(size_type) not implemented yet" << endl;
return false; // dummy return value
}
p_queue::size_type
p_queue::parent_index(size_type i) const
// Pre: (i > 0) && (i < used)
// Post: The index of "the parent of the item at heap[i]" has
// been returned.
{
cerr << "parent_index(size_type) not implemented yet" << endl;
return 0; // dummy return value
}
p_queue::size_type
p_queue::parent_priority(size_type i) const
// Pre: (i > 0) && (i < used)
// Post: The priority of "the parent of the item at heap[i]" has
// been returned.
{
cerr << "parent_priority(size_type) not implemented yet" << endl;
return 0; // dummy return value
}
p_queue::size_type
p_queue::big_child_index(size_type i) const
// Pre: is_leaf(i) returns false
// Post: The index of "the bigger child of the item at heap[i]"
// has been returned.
// (The bigger child is the one whose priority is no smaller
// than that of the other child, if there is one.)
{
cerr << "big_child_index(size_type) not implemented yet" << endl;
return 0; // dummy return value
}
p_queue::size_type
p_queue::big_child_priority(size_type i) const
// Pre: is_leaf(i) returns false
// Post: The priority of "the bigger child of the item at heap[i]"
// has been returned.
// (The bigger child is the one whose priority is no smaller
// than that of the other child, if there is one.)
{
cerr << "big_child_priority(size_type) not implemented yet" << endl;
return 0; // dummy return value
}
void p_queue::swap_with_parent(size_type i)
// Pre: (i > 0) && (i < used)
// Post: The item at heap[i] has been swapped with its parent.
{
cerr << "swap_with_parent(size_type) not implemented yet" << endl;
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

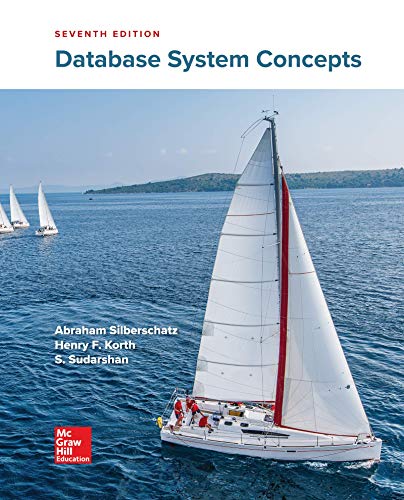
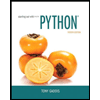
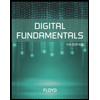
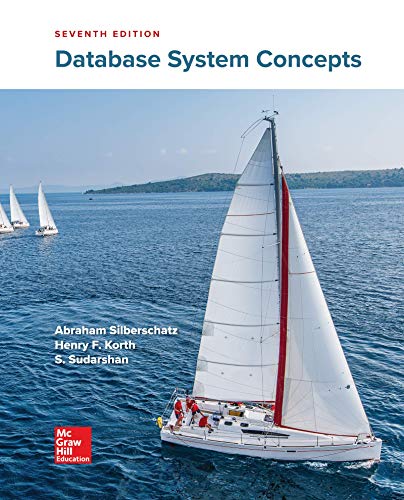
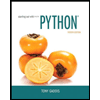
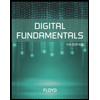
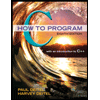
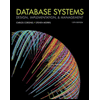
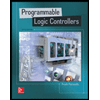