You will analyze three algorithms to solve the maximum contiguous subsequence sum problem, and then evaluate the performance of instructor-supplied implementations of those three algorithms. You will compare your theoretical results to your actual results in a written report. What is the maximum contiguous subsequence sum problem? Given a sequence of integers A1, A2, ..., An (where the integers may be positive or negative), find a subsequence Aj, ... , Ak that has the maximum value of all possible subsequences. The maximum contiguous subsequence sum is defined to be zero if all of the integers in the sequence are negative. Consider the sequence shown below. A1: -2 A2: 11 A3: -4 A4: 13
You will analyze three algorithms to solve the maximum contiguous subsequence sum problem, and then evaluate the performance of instructor-supplied implementations of those three algorithms. You will compare your theoretical results to your actual results in a written report.
What is the maximum contiguous subsequence sum problem?
Given a sequence of integers A1, A2, ..., An (where the integers may be positive or negative), find a subsequence Aj, ... , Ak that has the maximum value of all possible subsequences.
The maximum contiguous subsequence sum is defined to be zero if all of the integers in the sequence are negative.
Consider the sequence shown below.
A1: -2
A2: 11
A3: -4
A4: 13
A5: -5
A6: 2
The maximum contiguous subsequence sum is 20, representing the contiguous subsequence in positions 2, 3, and 4 (i.e. 11 + (-4) + 13 = 20). The sum of the values in all other contiguous subsequences is less than or equal to 20.
Consider a second sequence, shown below.
A1: 1
A2: -3
A3: 4
A4: -2
A5: -1
A6: 6
The maximum contiguous subsequence sum is 7, representing the contiguous subsequence in positions 3, 4, 5, and 6 (i.e. 4 + (-2) + (-1) + 6 = 7).
Four different algorithms have been developed to solve this problem, you can download them below.
You will:
1. Analyze each of the three algorithms in source code form.
To analyze an
2. Compile and run the program to evaluate the performance of the three functions.
To evaluate the performance of an algorithm on a computer, you will call the functions in a driver (such as the one we supplied: proj9driver.cpp ) and execute the program. The driver can call these three functions with different prototypes shown below.
To evaluate a function, you will execute the program which uses that function for each of the following input sequence sizes:
N = 64, 128, 256, 512, 1024, 2048
3. Write a report comparing your theoretical and actual results from 1. and 2.
In your report, include the times of all test cases for each combination of N and function (COLOR).
Your report will contain the following sections (in the order stated):
a) analysis of Algorithm #1 (contained in "proj9algorithm1.cpp download"-- this is the Blue function)
b) analysis of Algorithm #2 (contained in "proj9algorithm2.cpp download"-- this is the Green function)
c) analysis of Algorithm #3 (contained in "proj9algorithm3.cpp download"-- this is the Red function)
d) the name and specification of the computer such as the
e) actual running times for function BLUE
f) actual running times for function GREEN
g) actual running times for function RED
h) a statement about which algorithms from a) through c) are implemented by which functions (e) through (g) and conclusions about your theoretical and actual results
In sections (e) through (g), be sure to include the maximum contiguous subsequence sum for each array size to demonstrate that the computations are correct.
proj9algorithm1.cpp
// Algorithm #1
int Max_Subsequence_Sum( const int A[], const int N ) { int This_Sum = 0, Max_Sum = 0; for (int i = 0; i < N; i++) { This_Sum = 0; for (int j = i; j < N; j++) { This_Sum += A[j]; if (This_Sum > Max_Sum) { Max_Sum = This_Sum; } } } return Max_Sum;}
proj9algorithm2.cpp
// Algorithm #2int Max_Subsequence_Sum( const int A[], const int N ){ int This_Sum = 0, Max_Sum = 0; for (int i = 0; i < N; i++) { for (int j = i; j < N; j++) { This_Sum = 0; for (int k = i; k <= j; k++) { This_Sum += A[k]; } if (This_Sum > Max_Sum) { Max_Sum = This_Sum; } } } return Max_Sum;}
proj9algorithm3.cpp
// Algorithm #3int Max_Subsequence_Sum( const int A[], const int N ){ int This_Sum = 0, Max_Sum = 0; for (int Seq_End= 0; Seq_End < N; Seq_End++) { This_Sum += A[Seq_End]; if (This_Sum > Max_Sum) { Max_Sum = This_Sum; } else if (This_Sum < 0) { This_Sum = 0; } } return Max_Sum;}
![include <iostream>
include <lomanip>
linclude <cstring>
linclude <cstdlib>
linclude "timer.h"
using namespace std;
int Max Subsequence_Sum_BLUE( const int A[J, const int N)
int This Sum = 0, Max Sum = 0;
for (int i = 0; i < N; i++)
This Sum
for (int j = i; j < N; j++)
This Sum +- A[j];
if (This Sum > Max Sum)
Max Sum=
This Sum;
return Max_Sum;
int main( )
int Size
int *Vec, Result[6];
char Answer;
Timer T[6];
64;
for (int i - 0; i < 6; i++)
Vec
= new Int [5ize];
srand( time(6) );
for (int j = e; j < Size; j++)
Vec[j] = rand() X 100 - 5e;
rand() X 100 - 50;
cout << "Do you wish Lo view the array contents? (Y or N): ";
cin >> Answer;
If (Answer
== Y | Answer ==
'y')
for (Int J = 0; j < Size; J++)
cout << Vec[] << "\n";
cout << endl;
T[1].start();
Result[1]
T[1].stop();
Max Subsequence Sum BLUE( Vec, Size );
Size = 2 Size;
for ( int 1 = 0; 1 < 6; 1++)
cout << "Maximum contiguous subsequence sum: " << Result [i] << "\n":
T[1].show();
cout << endl;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda975a4b-126b-4e9c-824d-c5ef9f01d28b%2Ff43b2ff6-be52-4432-ae8b-98b5644382d1%2Fejrsyq_processed.jpeg&w=3840&q=75)
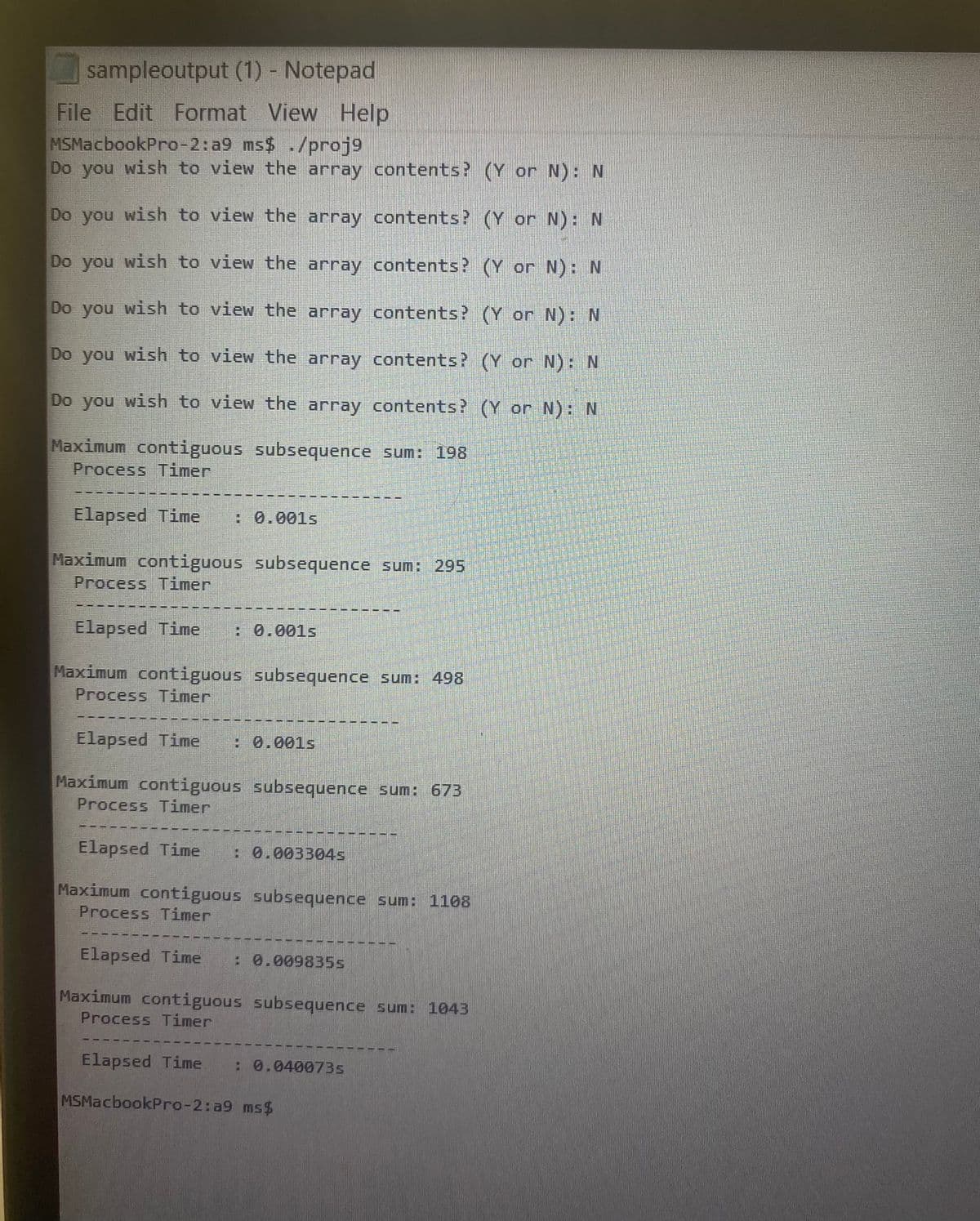

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

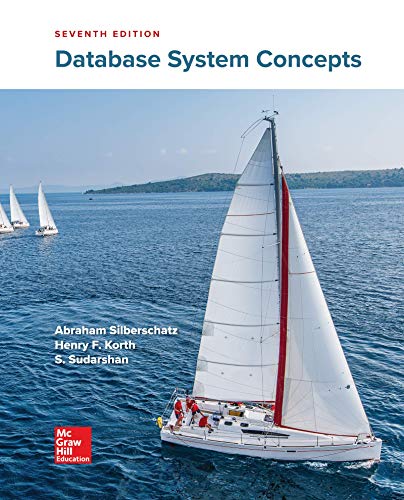
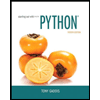
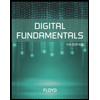
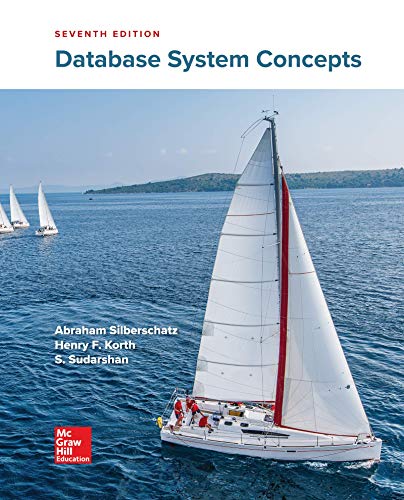
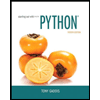
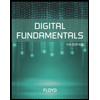
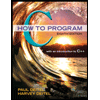
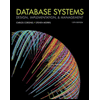
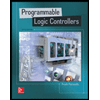