: You will build a simplified, one-player version of the classic board game Battleship! In this version of the game, there will be a single ship hidden in a random location on a 5 °ø 5 grid. The player will have 4 guesses at most to try to sink the ship. At each guess, the player names an attacking coordinate, that is (“guessrow”, “guess col”). The game ends in two conditions: (1) the player is out of guesses; (2) the player hits the ship. Examples are given in Figure 1. § The 5 X 5 board is shown every time the player inputs a guess entry. § The ship takes only one entry of the board, and it is randomly given before the player’s guesses. § The player inputs guessing entries in the Python console. § Entries that missed the ship are replaced by “X” on the board. § You must use a loop in your code. § Please submit your code and console screenshots to Blackboard. Code containing syntax error will be graded zero. Hints: 1. Create a variable board and set it equal to an empty list and create a 5 X 5 grid initialized to all ‘O’s and store it in the board. 2. Define two functions, random_row and random_col. Each takes the board as input. 3. Create new variables called guess_row and guess_col and set them to input("Guess Row:") and input("Guess Col:"), respectively. 4. If your guess misses the target, set the entry of the board at (guess_row, guess_col) to “X”. 5. The game should check if guess_row is not in range(5) or guess_col is not in range(5). If that is the case, print out "Oops, that’s not even in the ocean." 6. Thoroughly test your game. Make sure you try a variety of different guesses and look for any errors in the syntax or logic of your program. 7. Add a break under the win condition to end the loop after a win
: You will build a simplified, one-player version of the classic board game Battleship! In this version of the game, there will be a single ship hidden in a random location on a 5 °ø 5 grid. The player will have 4 guesses at most to try to sink the ship. At each guess, the player names an attacking coordinate, that is (“guessrow”, “guess col”). The game ends in two conditions: (1) the player is out of guesses; (2) the player hits the ship. Examples are given in Figure 1. § The 5 X 5 board is shown every time the player inputs a guess entry. § The ship takes only one entry of the board, and it is randomly given before the player’s guesses. § The player inputs guessing entries in the Python console. § Entries that missed the ship are replaced by “X” on the board. § You must use a loop in your code. § Please submit your code and console screenshots to Blackboard. Code containing syntax error will be graded zero. Hints: 1. Create a variable board and set it equal to an empty list and create a 5 X 5 grid initialized to all ‘O’s and store it in the board. 2. Define two functions, random_row and random_col. Each takes the board as input. 3. Create new variables called guess_row and guess_col and set them to input("Guess Row:") and input("Guess Col:"), respectively. 4. If your guess misses the target, set the entry of the board at (guess_row, guess_col) to “X”. 5. The game should check if guess_row is not in range(5) or guess_col is not in range(5). If that is the case, print out "Oops, that’s not even in the ocean." 6. Thoroughly test your game. Make sure you try a variety of different guesses and look for any errors in the syntax or logic of your program. 7. Add a break under the win condition to end the loop after a win

Algorithm:
The code implements the game as described in the prompt.
It starts by initializing an empty list board and then using a for loop to create a 5x5 grid initialized to all "O"s.
It defines two functions random_row and random_col that return a random row and column index respectively.
Then, it randomly selects the location of the ship on the board.
The main game logic is implemented in a for loop that goes through up to 4 turns.
In each turn, the player inputs their guess for the row and column, and the game checks if the guess matches the ship's location.
If the guess matches, the player wins and the game ends.
If the guess misses, the game replaces the missed location with an "X" and continues to the next turn.
If the player exhausts all their turns, the game ends with a "Game Over" message.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

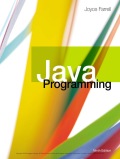
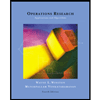
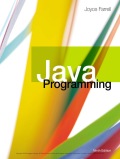
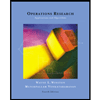