You work for an loan analytics organization have been tasked with writing a program that simulates an analysis of loan applications. Code a modular program that uses parallel arrays to store loan application credit scores (values generated between 300 and 900), score ratings (poor, fair, good, very good, or exceptional based on credit score) and loan status (approved or declined applications based on credit score). The program must do the following: The program must first ask the user for the number of loan applications that will be in the simulation. This must be done by calling the getNumLoanApplications() function (definition below). After the input of the number of accounts, the program must use loops and random numbers to generate the credit score, score rating, and application result for each loan application in parallel arrays. These arrays must not be declared globally (major error). The following arrays must be declared and populated: creditScores[] - an array of type int that holds the credit score for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in this array represent the credit scores for each loan application. Credit scores for loan applications range from 300 - 900. scoreRatings[] - an array of type string to hold the credit score rating for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the credit score rating for the loan application based on the credit score generated. In this simulation, credit scores can be one of the following: "poor" - Credit Score between 300 and 579 "fair" - Credit Score between 580 and 669 "good" - Credit Score between 670 and 739 "very good" - Credit Score between 740 and 799 "exceptional" - Credit Score between 800 and 900 loanStatuses[] - an array of type string to hold the status of each loan application. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the status of each loan application based on the credit score generated. In this simulation, a loan application is "Approved" if the credit score generated is 630 or higher, otherwise the loan application is "Declined". Populate each of these arrays by using a loop that will execute based on the number of loan applications the user entered in step 1. On each pass, call the generateCreditScore function (definition below) to generate a loan application credit score and store in the creditScores[] array. Then, call the generateScoreRating() function (definition below) using the credit score generated to generate a score rating and store in the scoreRatings[] array. Finally, call the generateStatus() function (definition below) using the credit score generated to generate a loan status and store in the loanStatuses[] array. Once all of the arrays are populated, the program must display the result statistics of the simulation. This includes displaying the status, credit score and the credit score rating in each loan application along with total counts of loans approved, declined, and credit score rating category counts. This must be done by calling the analysis() (defined below) function that accepts all of the arrays as well as the number of loan applications generated and evaluated in the simulation the user entered in step 1. main(). In this simulation, credit scores can be one of the following: "poor" - Credit Score between 300 and 579 "fair" - Credit Score between 580 and 669 "good" - Credit Score between 670 and 739 "very good" - Credit Score between 740 and 799 "exceptional" - Credit Score between 800 and 900 instructions for this in picture output be line Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 5000 Error : Invalid entry Enter the number of Loan Applications to be evaluated (min 50 - max 2000): -99 Error: Invalid entry Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 1500 Application Status: Approved Credit score: 745 Score rating: Very good Application Status: Approved Credit score: 639 Score rating: good Application Status: Declined Credit score: 200 Score rating: poor Application Status: Approved Credit score: 800 Score rating: exceptional Application Status: Declined Credit score: 544 Score rating: poor After 1500 rows --------------------------------------------- Stats:- --------------------------------- Total Applications: 1500 Loans Approved: 700 Loans Declined: 800 ------------------------------------------ Total Score by rating: # "poor" - Credit Score between (300 - 579 ) 2 # "fair" - Credit Score between ( 580 - 669) 399 # "good" - Credit Score between (670 - 739) 299 # "very good" - Credit Score between (740 - 799) 186 # "exceptional" - Credit Score between (800 - 900) 2 ----------
You work for an loan analytics organization have been tasked with writing a program that simulates an analysis of loan applications.
Code a modular program that uses parallel arrays to store loan application credit scores (values generated between 300 and 900), score ratings (poor, fair, good, very good, or exceptional based on credit score) and loan status (approved or declined applications based on credit score).
The program must do the following:
- The program must first ask the user for the number of loan applications that will be in the simulation. This must be done by calling the getNumLoanApplications() function (definition below).
- After the input of the number of accounts, the program must use loops and random numbers to generate the credit score, score rating, and application result for each loan application in parallel arrays. These arrays must not be declared globally (major error).
- The following arrays must be declared and populated:
-
- creditScores[] - an array of type int that holds the credit score for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in this array represent the credit scores for each loan application. Credit scores for loan applications range from 300 - 900.
- scoreRatings[] - an array of type string to hold the credit score rating for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the credit score rating for the loan application based on the credit score generated. In this simulation, credit scores can be one of the following:
- "poor" - Credit Score between 300 and 579
- "fair" - Credit Score between 580 and 669
- "good" - Credit Score between 670 and 739
- "very good" - Credit Score between 740 and 799
- "exceptional" - Credit Score between 800 and 900
- loanStatuses[] - an array of type string to hold the status of each loan application. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the status of each loan application based on the credit score generated. In this simulation, a loan application is "Approved" if the credit score generated is 630 or higher, otherwise the loan application is "Declined".
- Populate each of these arrays by using a loop that will execute based on the number of loan applications the user entered in step 1. On each pass, call the generateCreditScore function (definition below) to generate a loan application credit score and store in the creditScores[] array. Then, call the generateScoreRating() function (definition below) using the credit score generated to generate a score rating and store in the scoreRatings[] array. Finally, call the generateStatus() function (definition below) using the credit score generated to generate a loan status and store in the loanStatuses[] array.
-
- Once all of the arrays are populated, the program must display the result statistics of the simulation. This includes displaying the status, credit score and the credit score rating in each loan application along with total counts of loans approved, declined, and credit score rating category counts. This must be done by calling the analysis() (defined below) function that accepts all of the arrays as well as the number of loan applications generated and evaluated in the simulation the user entered in step 1.
main(). In this simulation, credit scores can be one of the following:
-
- "poor" - Credit Score between 300 and 579
- "fair" - Credit Score between 580 and 669
- "good" - Credit Score between 670 and 739
- "very good" - Credit Score between 740 and 799
- "exceptional" - Credit Score between 800 and 900
- instructions for this in picture
output be line
Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 5000
Error : Invalid entry
Enter the number of Loan Applications to be evaluated (min 50 - max 2000): -99
Error: Invalid entry
Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 1500
Application Status: Approved Credit score: 745 Score rating: Very good
Application Status: Approved Credit score: 639 Score rating: good
Application Status: Declined Credit score: 200 Score rating: poor
Application Status: Approved Credit score: 800 Score rating: exceptional
Application Status: Declined Credit score: 544 Score rating: poor
After 1500 rows
---------------------------------------------
Stats:-
---------------------------------
Total Applications: 1500
Loans Approved: 700
Loans Declined: 800
------------------------------------------
Total Score by rating:
# "poor" - Credit Score between (300 - 579 ) 2
# "fair" - Credit Score between ( 580 - 669) 399
# "good" - Credit Score between (670 - 739) 299
# "very good" - Credit Score between (740 - 799) 186
# "exceptional" - Credit Score between (800 - 900) 2
----------
![In addition to the main() function, declare prototypes, write function definitions and correctly call the following functions:
• getNumLoanApplications() - this is an integer-returning function that must allow the user to specify the number of loan applications that will be
evaluated in the simulation. This company can simulate between 50 and 2000 applications in the simulation. Validate the user input so that the user
must enter a value between 50 and 2000 loans to be generated. If the user enters an invalid number of loan applications, they must be given an
unlimited amount of chances to enter a valid number of loan applications. The function must return the validated number of loan applications.
generateCreditScore() - this is an int-returning function that generates a random loan application credit score between 300 and 900. Note: use
the int data type to return the transaction amount and store in the creditScores[] array in main().
• generateScoreRating() - this is a string-returning function that generates a score rating based on the credit score. The function must accept
the credit score generated for a loan application as a parameter. Based on the value of the credit score generate a string that represents the
value of the credit score rating. Return the appropriate credit rating string and store in the scoreRatings array in main(). In this simulation,
credit scores can be one of the following:
o "poor" - Credit Score between 300 and 579
o "fair" - Credit Score between 580 and 669
o "good" - Credit Score between 670 and 739
o "very good" - Credit Score between 740 and 799
o "exceptional" - Credit Score between 800 and 900
• generate Status() - this is a string-returning function that generates a status for the loan application based on the credit score. The function
must accept the credit score generated for a loan application as a parameter. In this simulation, a loan application is "Approved" if the credit
score generated is 630 or higher, otherwise the loan application is "Declined". Return the string and store in the loan Statuses[] array in
main();
• analysis () - Function must accept the parallel arrays that contain the credit scores, loan statuses, and credit score ratings, as well as the number of
loan applications in the simulation and display the following information for each loan: Application Status, Credit Score, and Score
Rating formatted with proper spacing on separate lines to match the sample output. Then calculate and display the following:
• The total number of loan applications (count)
o The total number of approved applications (count)
o The total number of declined applications (count)
o The count of poor credit scores
o The count of fair credit scores
• The count of good credit scores
o The count of very good credit scores
o The count of exceptional credit scores
Other Notes:
Program Output and Prompts MUST MATCH the formatting of the sample runs EXACTLY.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffb4fd0f6-7be1-48bb-9974-e6a33a794ca8%2F9bdc2576-63be-48b0-8a42-8abb8cc44ead%2Fx9ssasg_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 4 images

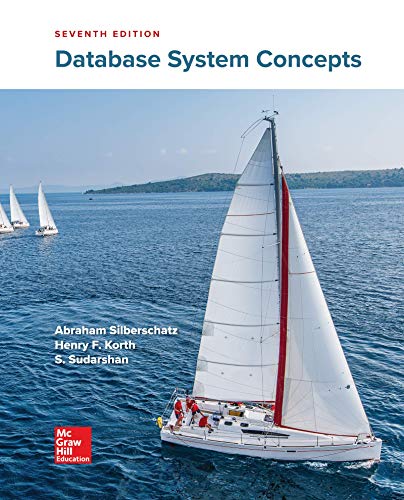
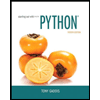
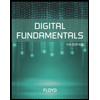
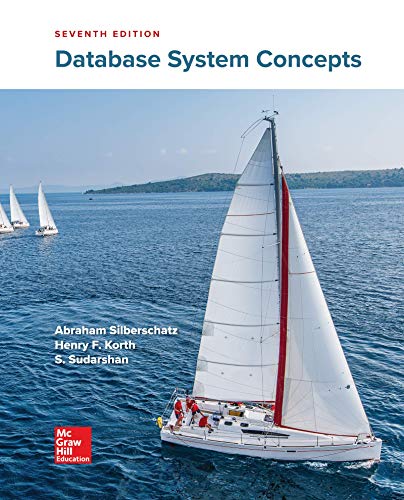
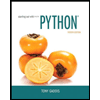
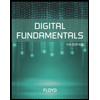
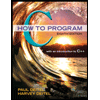
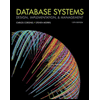
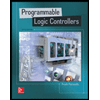