Each function (function name plotNegXSquaredPlus25) should accept two integer parameters indicating the minimum and maximum X value to be plotted. The functions should have no return values. Create class-level constants for things we might wish to change later, e.g., a char called PLOT_CHAR that holds an “x” (per samples below), another char called FILL_CHAR that holds an unobtrusive character like ASCII 183, generated with a cast, e.g., (char)183. JAVA PLEASE THOSE ARE NOT PERIODS OR DOTS. USE (System.out.print((Char)183); for (.) Please DON'T USE Loops(while);, if, Arrays, lists, or other data structures, objects, libraries, or methods. Sideways Plot y = -(x*x)+25 where -5<=x<=5 x ·········x ················x ·····················x ························x ·························x ························x ·····················x ················x ·········x x The area under the plot is 165
Each function (function name plotNegXSquaredPlus25) should accept two integer parameters indicating the minimum and maximum X value to be plotted. The functions should have no return values. Create class-level constants for things we might wish to change later, e.g., a char called PLOT_CHAR that holds an “x” (per samples below), another char called FILL_CHAR that holds an unobtrusive character like ASCII 183, generated with a cast, e.g., (char)183.
JAVA PLEASE
THOSE ARE NOT PERIODS OR DOTS.
USE (System.out.print((Char)183); for (.)
Please DON'T USE Loops(while);, if, Arrays, lists, or other data structures, objects, libraries, or methods.
Sideways Plot
y = -(x*x)+25 where -5<=x<=5
x
·········x
················x
·····················x
························x
·························x
························x
·····················x
················x
·········x
x
The area under the plot is 165

Step by step
Solved in 4 steps with 2 images

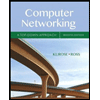
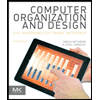
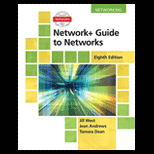
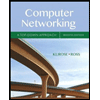
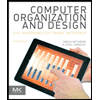
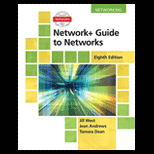
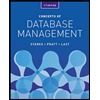
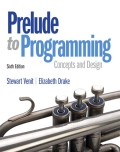
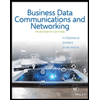