Your Tasks: 1. Item class encapsulating a shopping item. An item has the following attributes: a. Name(String), the length of name is limited to [1, 50], use the first 50 characters if its length is greater than 50. For null or an empty String, throw RuntimeException with appropriate messages-either null or an empty String is not allowed. b. Category(String), the length of name is limited to [1, 50], use the first 50 characters if its lengt is greater than 50. For null or an empty String, throw RuntimeException with appropriate messages-either null or an empty String is not allowed. c. Quantity(int), its value is limited to [1, 100], for all invalid values, set default to 1. d. unitPrice(double). Its value is limited to [0, 1000], for all invalid values, set to 0.99. 2. The Item class implements Comparable that compares two items based on category, then item name, ignoring cases when comparing. 3. Item class should also include: a. Constructor that takes name, category, quantity, and unitPrice as parameters. b. All accessor and mutator methods c. Override toString() method to return item information as follows: (please note: without category, we will handle category in our ShoppingList ADT) Subtotal, for Item Name Quantity example: Eggs 2 $5.99 d. Subtotal() returns quantity"unitPrice 4. ShoppingList class. This class has the following methods: a. isEmpty() b. isFull() c. size()-return the number of unique items on the shopping list d. totalltems()-return the total number of items on the shopping list. For example, after adding two meats and 3 boxes of eggs, calling size() should return 2, while calling totalltems() should return 2+3= 5. e. grandTotal()-returns ground total of items on the shopping list. f. printNames()-print unique item names on the shopping list. g. print()-item name, quantity, subtotal, and grand total. Display categories for all items in the same category. Please refer to sample output at the end of this document. h. insert(Item item) with the following requirements: • if null item is not allowed: do nothing if null • No duplicated items-all items' category and name must be unique. If an item with the sam name and category already exists in the list, simply add quantity to the existing item in the list After inserting an item, the list should maintain sorted-based on category and name i. remove(Item item) with the following requirements: • Ifitem not found(item category and name), display the item does not exist in the list, otherwise, remove the item from the list.
Your Tasks: 1. Item class encapsulating a shopping item. An item has the following attributes: a. Name(String), the length of name is limited to [1, 50], use the first 50 characters if its length is greater than 50. For null or an empty String, throw RuntimeException with appropriate messages-either null or an empty String is not allowed. b. Category(String), the length of name is limited to [1, 50], use the first 50 characters if its lengt is greater than 50. For null or an empty String, throw RuntimeException with appropriate messages-either null or an empty String is not allowed. c. Quantity(int), its value is limited to [1, 100], for all invalid values, set default to 1. d. unitPrice(double). Its value is limited to [0, 1000], for all invalid values, set to 0.99. 2. The Item class implements Comparable that compares two items based on category, then item name, ignoring cases when comparing. 3. Item class should also include: a. Constructor that takes name, category, quantity, and unitPrice as parameters. b. All accessor and mutator methods c. Override toString() method to return item information as follows: (please note: without category, we will handle category in our ShoppingList ADT) Subtotal, for Item Name Quantity example: Eggs 2 $5.99 d. Subtotal() returns quantity"unitPrice 4. ShoppingList class. This class has the following methods: a. isEmpty() b. isFull() c. size()-return the number of unique items on the shopping list d. totalltems()-return the total number of items on the shopping list. For example, after adding two meats and 3 boxes of eggs, calling size() should return 2, while calling totalltems() should return 2+3= 5. e. grandTotal()-returns ground total of items on the shopping list. f. printNames()-print unique item names on the shopping list. g. print()-item name, quantity, subtotal, and grand total. Display categories for all items in the same category. Please refer to sample output at the end of this document. h. insert(Item item) with the following requirements: • if null item is not allowed: do nothing if null • No duplicated items-all items' category and name must be unique. If an item with the sam name and category already exists in the list, simply add quantity to the existing item in the list After inserting an item, the list should maintain sorted-based on category and name i. remove(Item item) with the following requirements: • Ifitem not found(item category and name), display the item does not exist in the list, otherwise, remove the item from the list.
Chapter3: Using Methods, Classes, And Objects
Section: Chapter Questions
Problem 1GZ
Related questions
Question
public class ShoppingListDriver{
public static void main(String[] args){
ShoppingList sl=new ShoppingList(3);
sl.insert(null);
sl.insert(new Item("Bread", "Carb Food", 2, 2.99));
sl.insert(new Item("Seafood","Sea Food", -1, 10.99));
sl.insert(new Item("Rice", "Carb Food",2, 19.99));
sl.insert(new Item("Salad Dressings","Dessing", 2, 19.99));
sl.insert(new Item("Eggs", "Protein",2, 3.99));
sl.insert(new Item("Cheese","Protein", 2, 1.59));
sl.insert(new Item("Eggs", "Protein",3, 3.99));
sl.printNames();
sl.print();
System.out.println("After removing Eggs:");
sl.remove(new Item("Eggs","Protein",0,0));
sl.printNames();
sl.print();
}
}
![Your Tasks:
1. Item class encapsulating a shopping item. An item has the following attributes:
a. Name(String), the length of name is limited to [1, 50], use the first 50 characters if its length is
greater than 50. For null or an empty String, throw RuntimeException with appropriate
messages-either null or an empty String is not allowed.
b. Category(String), the length of name is limited to [1, 50], use the first 50 characters if its length
is greater than 50. For null or an empty String, throw RuntimeException with appropriate
messages-either null or an empty String is not allowed.
c. Quantity(int), its value is limited to [1, 100], for all invalid values, set default to 1.
d. unitPrice(double). Its value is limited to [0, 1000], for all invalid values, set to 0.99.
2. The Item class implements Comparable that compares two items based on category, then item
name, ignoring cases when comparing.
3. Item class should also include:
a. Constructor that takes name, category, quantity, and unitPrice as parameters.
b. All accessor and mutator methods
c. Override toString() method to return item information as follows: (please note: without
category, we will handle category in our ShoppingList ADT)
Subtotal, for
Item Name
Quantity
example:
Eggs
2
$5.99
d. Subtotall) returns quantity"unitPrice
4. ShoppingList class. This class has the following methods:
a. isEmpty()
b. isFull()
c. size()-return the number of unique items on the shopping list
d. totalltems()-return the total number of items on the shopping list. For example, after adding
two meats and 3 boxes of eggs, calling size() should return 2, while calling totalltems() should
return 2+3= 5.
e. grandTotal()-returns ground total of items on the shopping list.
f. printNames()-print unique item names on the shopping list.
g. print()-item name, quantity, subtotal, and grand total. Display categories for all items in the
same category. Please refer to sample output at the end of this document.
h. insert(Item item) with the following requirements:
• if null item is not allowed: do nothing if null
• No duplicated items-all items' category and name must be unique. If an item with the same
name and category already exists in the list, simply add quantity to the existing item in the
list
· After inserting an item, the list should maintain sorted-based on category and name
i. remove(ltem item) with the following requirements:
• If item not found(item category and name), display the item does not exist in the list,
otherwise, remove the item from the list.
5. Once completed, download and run the ShoppingListDriver class.
• ShoppingListDriver.java
6. If you implement all the required methods properly, the driver program should generate outputs
similar to the following:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1144a455-13cb-49b0-8cf0-5b36bab3656a%2F547f20b1-7c89-42b9-a995-06b635cf094c%2Fr8qbtls_processed.png&w=3840&q=75)
Transcribed Image Text:Your Tasks:
1. Item class encapsulating a shopping item. An item has the following attributes:
a. Name(String), the length of name is limited to [1, 50], use the first 50 characters if its length is
greater than 50. For null or an empty String, throw RuntimeException with appropriate
messages-either null or an empty String is not allowed.
b. Category(String), the length of name is limited to [1, 50], use the first 50 characters if its length
is greater than 50. For null or an empty String, throw RuntimeException with appropriate
messages-either null or an empty String is not allowed.
c. Quantity(int), its value is limited to [1, 100], for all invalid values, set default to 1.
d. unitPrice(double). Its value is limited to [0, 1000], for all invalid values, set to 0.99.
2. The Item class implements Comparable that compares two items based on category, then item
name, ignoring cases when comparing.
3. Item class should also include:
a. Constructor that takes name, category, quantity, and unitPrice as parameters.
b. All accessor and mutator methods
c. Override toString() method to return item information as follows: (please note: without
category, we will handle category in our ShoppingList ADT)
Subtotal, for
Item Name
Quantity
example:
Eggs
2
$5.99
d. Subtotall) returns quantity"unitPrice
4. ShoppingList class. This class has the following methods:
a. isEmpty()
b. isFull()
c. size()-return the number of unique items on the shopping list
d. totalltems()-return the total number of items on the shopping list. For example, after adding
two meats and 3 boxes of eggs, calling size() should return 2, while calling totalltems() should
return 2+3= 5.
e. grandTotal()-returns ground total of items on the shopping list.
f. printNames()-print unique item names on the shopping list.
g. print()-item name, quantity, subtotal, and grand total. Display categories for all items in the
same category. Please refer to sample output at the end of this document.
h. insert(Item item) with the following requirements:
• if null item is not allowed: do nothing if null
• No duplicated items-all items' category and name must be unique. If an item with the same
name and category already exists in the list, simply add quantity to the existing item in the
list
· After inserting an item, the list should maintain sorted-based on category and name
i. remove(ltem item) with the following requirements:
• If item not found(item category and name), display the item does not exist in the list,
otherwise, remove the item from the list.
5. Once completed, download and run the ShoppingListDriver class.
• ShoppingListDriver.java
6. If you implement all the required methods properly, the driver program should generate outputs
similar to the following:
![(Bread, Rice, Salad Dressings, Cheese, Eggs, Seafood]
Name
Qty $Subtotal
Carb Food:
1. Bread
2. Rice
2 $5.98
2 $39.98
Dessing
1. Salad Dressings
2 $39.98
Protein:
1. Cheese
2. Eggs
2 $3.18
5 $19.95
Sea Food:
1. Seafood
1 $10.99
Number of Items: 14
Grand Totalt : $120.06
After removing Eggs:
(Bread, Rice, Salad Dressings, Cheese, Seafood]
Name
Qty $Subtotal
Carb Food:
1. Bread
2. Rice
2 $5.98
2 $39.98
Dessing:
1. Salad Dressings
2 $39.98
Protein:
1. Cheese
2 $3.18
Sea Food
1. Seafood
1 $10.99
Number of Items:
9
Grand Total:: $100.11](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1144a455-13cb-49b0-8cf0-5b36bab3656a%2F547f20b1-7c89-42b9-a995-06b635cf094c%2F0ki8ric_processed.png&w=3840&q=75)
Transcribed Image Text:(Bread, Rice, Salad Dressings, Cheese, Eggs, Seafood]
Name
Qty $Subtotal
Carb Food:
1. Bread
2. Rice
2 $5.98
2 $39.98
Dessing
1. Salad Dressings
2 $39.98
Protein:
1. Cheese
2. Eggs
2 $3.18
5 $19.95
Sea Food:
1. Seafood
1 $10.99
Number of Items: 14
Grand Totalt : $120.06
After removing Eggs:
(Bread, Rice, Salad Dressings, Cheese, Seafood]
Name
Qty $Subtotal
Carb Food:
1. Bread
2. Rice
2 $5.98
2 $39.98
Dessing:
1. Salad Dressings
2 $39.98
Protein:
1. Cheese
2 $3.18
Sea Food
1. Seafood
1 $10.99
Number of Items:
9
Grand Total:: $100.11
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
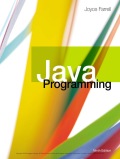
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
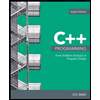
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
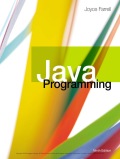
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
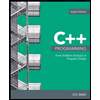
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning