You’ve been given a list of books to read over the summer, but you only want to read the books by a certain author. Write a method public List filterBooks(List readingList, String author) That takes a List of Books as a parameter, removes all Books from the readingList that are not written by the given author, then returns the resulting list. You can access the author of a Book by calling book.getAuthor(). The Book class is provided for reference. public List filterBooks(List readingList, String author) { // Remove all Books from the readingList that are not // written by the given author. Return the resulting List for(int i = 0; i < readingList.size(); i++) { //does current author == author? String currentBook = readingList.get(i); if(!currentBook.getAuthor().equals(author)) { readingList.remove(i); i--; } } return readingList; } Book.java public class Book { private String title; private String author; public Book(String theTitle, String theAuthor) { title = theTitle; author = theAuthor; } public String getTitle() { return title; } public String getAuthor() { return author; } public String toString() { return title + " by " + author; } public boolean equals(Book other) { return title.equals(other.title) && author.equals(other.author); }
You’ve been given a list of books to read over the summer, but you only want to read the books by a certain author.
Write a method
public List<Book> filterBooks(List<Book> readingList, String author)
That takes a List of Books as a parameter, removes all Books from the readingList that are not written by the given author, then returns the resulting list.
You can access the author of a Book by calling book.getAuthor(). The Book class is provided for reference.
public List<Book> filterBooks(List<Book> readingList, String author)
{
// Remove all Books from the readingList that are not
// written by the given author. Return the resulting List
for(int i = 0; i < readingList.size(); i++)
{
//does current author == author?
String currentBook = readingList.get(i);
if(!currentBook.getAuthor().equals(author))
{
readingList.remove(i);
i--;
}
}
return readingList;
}
Book.java
public class Book
{
private String title;
private String author;
public Book(String theTitle, String theAuthor)
{
title = theTitle;
author = theAuthor;
}
public String getTitle()
{
return title;
}
public String getAuthor()
{
return author;
}
public String toString()
{
return title + " by " + author;
}
public boolean equals(Book other)
{
return title.equals(other.title) && author.equals(other.author);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

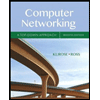
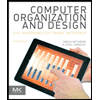
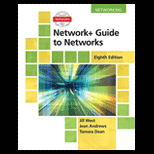
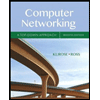
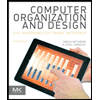
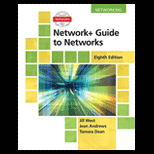
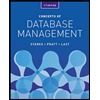
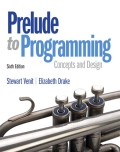
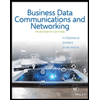