1. Again start a new program. This time, you need to #include . 2. Next, write code to prompt the user to enter a string, and use getline() to obtain the string from the user 3. Now, create an istringstream object on the string. This is easily done using the istringstream constructor. For instance, if your string is held in the variable str, and you want to create a stringstream object named sin, you can create the object using istringstream sin(str); Here, I've named the object sin (string input"), but you can name it whatever you want. 4. Remember how we used the eof) method to detect when we hit the end of a file? The same method is used for istringstreams to detect when you reach the end of the input string. So you can now write a loop that uses the >>operator on your new istringstream object to get the individual strings from the string passed in by the user 5. For bonus points (well, not really as this part isn't graded), try using the >operator, the good method, and the clear) method to take in an arbitrary string and parse it into doubles (when possible) and strings for anything not matching a double. (It would be nice to further distinguish between integers and doubles, but that takes a bit more work.) Your program should output what kind of value you read, and the value itself. For instance, if the input is "The fourth digit of 3.14159265 is 1", your output might look like string: The string: fourth string: digit string: of number: 3.14159265 string: is number: 1 Task 4: Put it all together For the final task, you are provided with a text file, "lines.txt", and asked to discover two facts from the file. The file contains a large number of English words (from a Scrabble dictionary); one of these is larger than al of the others. Your job is to a) find that word and tell us what it is and b) tell us what line number the word is on. The file is laid out as follows: The first line has three integers. The first integer is the number of lines after the first line. The second and third integers are the minimum and maximum number of words on each line, respectively Each subsequent line contains words separated by spaces Assume that the first line of text (not the first line of the file) is line number 1 for lie numbering purposes. To find the information we're asking for, you'll want to do something like the following: 1. Read in the first line of the file to get the number of lines of text. 2. Read in the lines of text one by one using getline). 3. Read in each word from the line using an istringstream object 4. Keep track of the longest word you've found, and what line you found it on.
1. Again start a new program. This time, you need to #include . 2. Next, write code to prompt the user to enter a string, and use getline() to obtain the string from the user 3. Now, create an istringstream object on the string. This is easily done using the istringstream constructor. For instance, if your string is held in the variable str, and you want to create a stringstream object named sin, you can create the object using istringstream sin(str); Here, I've named the object sin (string input"), but you can name it whatever you want. 4. Remember how we used the eof) method to detect when we hit the end of a file? The same method is used for istringstreams to detect when you reach the end of the input string. So you can now write a loop that uses the >>operator on your new istringstream object to get the individual strings from the string passed in by the user 5. For bonus points (well, not really as this part isn't graded), try using the >operator, the good method, and the clear) method to take in an arbitrary string and parse it into doubles (when possible) and strings for anything not matching a double. (It would be nice to further distinguish between integers and doubles, but that takes a bit more work.) Your program should output what kind of value you read, and the value itself. For instance, if the input is "The fourth digit of 3.14159265 is 1", your output might look like string: The string: fourth string: digit string: of number: 3.14159265 string: is number: 1 Task 4: Put it all together For the final task, you are provided with a text file, "lines.txt", and asked to discover two facts from the file. The file contains a large number of English words (from a Scrabble dictionary); one of these is larger than al of the others. Your job is to a) find that word and tell us what it is and b) tell us what line number the word is on. The file is laid out as follows: The first line has three integers. The first integer is the number of lines after the first line. The second and third integers are the minimum and maximum number of words on each line, respectively Each subsequent line contains words separated by spaces Assume that the first line of text (not the first line of the file) is line number 1 for lie numbering purposes. To find the information we're asking for, you'll want to do something like the following: 1. Read in the first line of the file to get the number of lines of text. 2. Read in the lines of text one by one using getline). 3. Read in each word from the line using an istringstream object 4. Keep track of the longest word you've found, and what line you found it on.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I am trying to read in a text file called "lines.txt" that holds an entire dictionary of words with multiple words on each line. I am supposed to find the longest word in the file and the line number it is on. So far I know that I am supposed to have a line counter when initializing the first get line() to 1 but I am confused on how to parse through it.
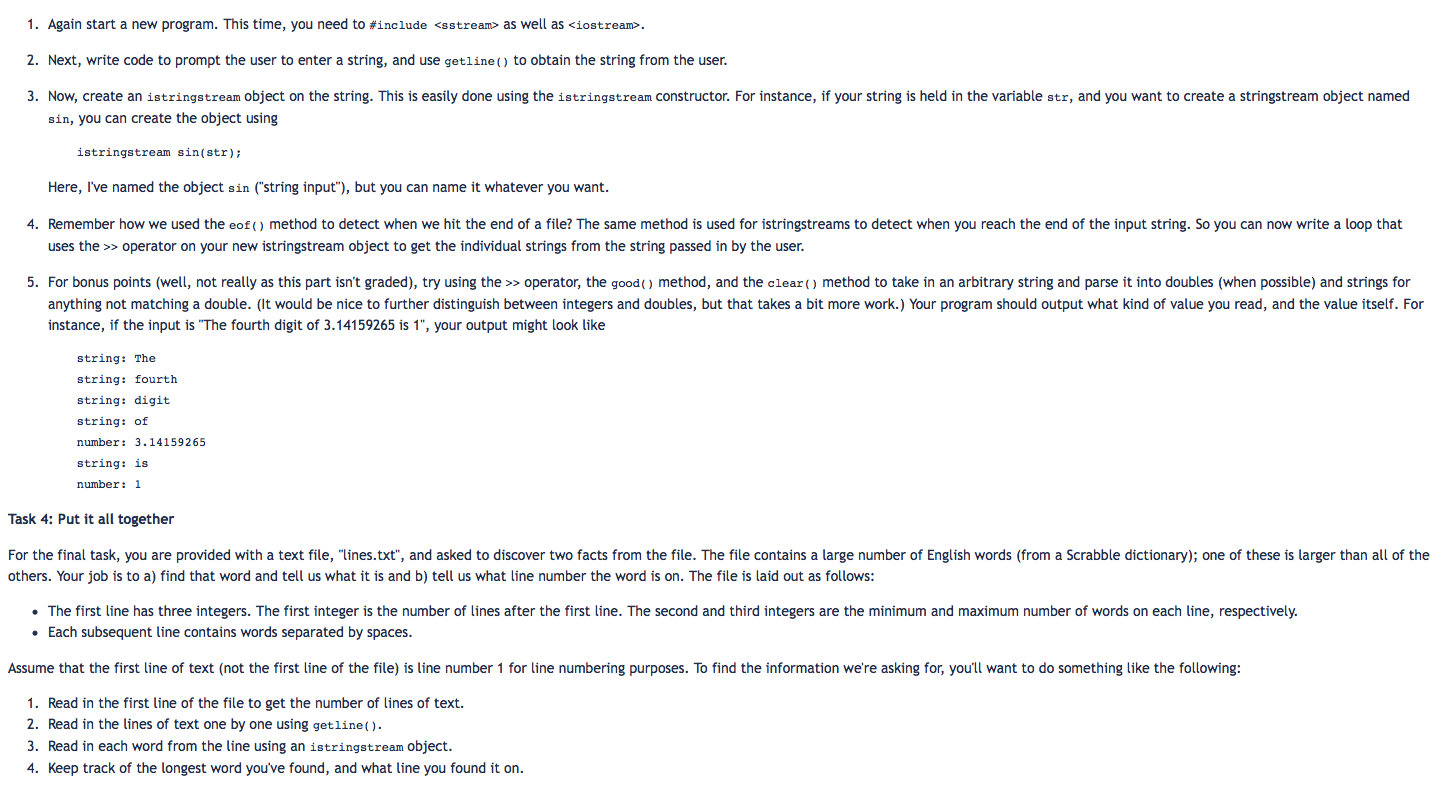
Transcribed Image Text:1. Again start a new program. This time, you need to #include <s stream» as well as <iostream>.
2. Next, write code to prompt the user to enter a string, and use getline() to obtain the string from the user
3. Now, create an istringstream object on the string. This is easily done using the istringstream constructor. For instance, if your string is held in the variable str, and you want to create a stringstream object named
sin, you can create the object using
istringstream sin(str);
Here, I've named the object sin (string input"), but you can name it whatever you want.
4. Remember how we used the eof) method to detect when we hit the end of a file? The same method is used for istringstreams to detect when you reach the end of the input string. So you can now write a loop that
uses the >>operator on your new istringstream object to get the individual strings from the string passed in by the user
5. For bonus points (well, not really as this part isn't graded), try using the >operator, the good
method, and the clear) method to take in an arbitrary string and parse it into doubles (when possible) and strings for
anything not matching a double. (It would be nice to further distinguish between integers and doubles, but that takes a bit more work.) Your program should output what kind of value you read, and the value itself. For
instance, if the input is "The fourth digit of 3.14159265 is 1", your output might look like
string: The
string: fourth
string: digit
string: of
number: 3.14159265
string: is
number: 1
Task 4: Put it all together
For the final task, you are provided with a text file, "lines.txt", and asked to discover two facts from the file. The file contains a large number of English words (from a Scrabble dictionary); one of these is larger than al of the
others. Your job is to a) find that word and tell us what it is and b) tell us what line number the word is on. The file is laid out as follows:
The first line has three integers. The first integer is the number of lines after the first line. The second and third integers are the minimum and maximum number of words on each line, respectively
Each subsequent line contains words separated by spaces
Assume that the first line of text (not the first line of the file) is line number 1 for lie numbering purposes. To find the information we're asking for, you'll want to do something like the following:
1. Read in the first line of the file to get the number of lines of text.
2. Read in the lines of text one by one using getline).
3. Read in each word from the line using an istringstream object
4. Keep track of the longest word you've found, and what line you found it on.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
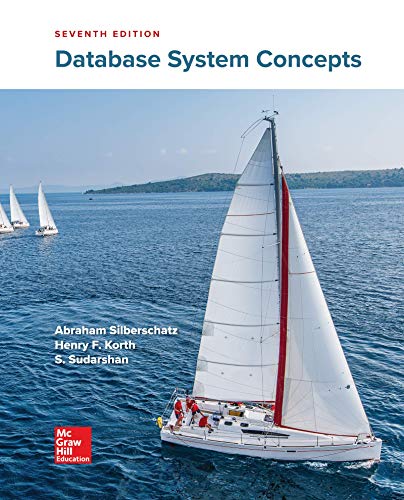
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
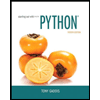
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
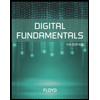
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
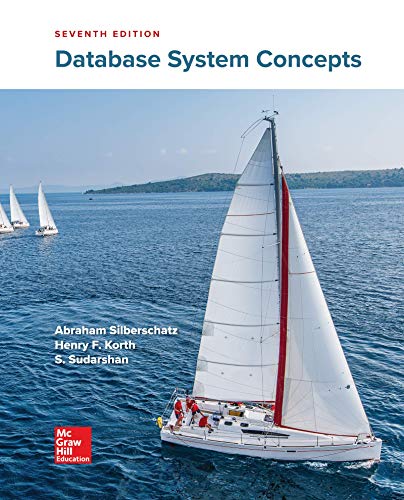
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
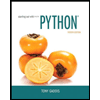
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
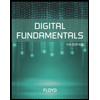
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
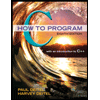
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
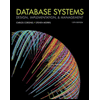
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
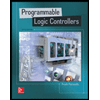
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education