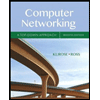
NEED HELP PYTHON PROGRAMMING ONLY SORTING AND SEARCHES (CODE GIVEN AS WELL)
Start with the code given to you below. This code will show you how to generate a list
of 1000 unique random numbers between 1 and 32000. The list will not be in any
specific order (i.e., not sorted). You only need to generate this list ONCE at the
beginning of your code. You can work on this same list for all the following steps.
2. Linear Search
a. Implement a simple linear search
do not remember what a linear search is) to look for a certain number. Use the
code in the example below (the line that assigns a number to value) to
determine what number you are trying to find. The code will guarantee that
number is in your list.
b. Make a loop to repeat the process of picking a search value (using the code
below) and searching for it. Repeat the pick-a-number/linear-search 10 times.
Time each iteration of your loop and calculate an average time for each search
after it has been done ten times. Print the average time for linear searches.
3. Index Method
a. Pick a new search value and use the list .index() method to locate the index of
the value.
b. Make another loop to repeat the process of picking a search value (using the
code below) and searching for it. Repeat the pick-a-number/index()-search 10
times. Time each iteration of your loop and calculate an average time for each
search after it has been done ten times. Print the average time for index()
searches.
4. Binary Search
a. Create the code to do a binary search algorithm. This code is a little tricker, so
you can either try to do this on your own to challenge yourself or find some
code on the Internet to do a binary search (there are a number of Python
binary search code examples out there). For the binary search code, this is one
time there is no problem copying a function directly from the internet directly.
This does not mean that posting this entire assignment to a homework and
programming site and getting the answer is OK, just getting the binary search
code!
b. If you create your own code for a binary search, you MUST put a comment in
to indicate that you are not using anyone else’s code. If you are trying to do the
code on your own, this will be considered when the assignment is graded. If
you use code from the Internet (which is OK for the binary search code), you
MUST put a comment in the code to indicate that you are using code from the
Internet and where you obtained the code.
c. Make another loop to repeat the process of picking a search value (using the
code below) and searching for it. Repeat the pick-a-number/binary search 10
times. Time each iteration of your loop and calculate an average time for each
search after it has been done ten times. Print the average time for binary
searches.
5. Finally, put comments in your code to discuss the results you get. Things like:
a. Which is the fastest?
b. Which is the slowest?
c. If you run your program multiple times, are the numbers consistent?
CODE BELOW:
##
## Import libraries – This needs to be at the top of your code
##
import random
import time
##
## The next thing you need to do is to create a list that contains
## 1000 randomly generated numbers between 1 and 32,000. The numbers
## are in a random order (not sorted).
##
data_set = random.sample(range(1, 32_001), k=1000)
##
## This bit of code will pick a random value from the list of numbers.
## Use this line of code to pick a search target EACH time before
## you start a new timed search. Please note that the search target
## is not an index into the data_set list, but one of the values
## contained in the data_set list.
##
value = data_set[random.randint(1,1000)-1]
##
## Here is some example code to time something in Python. This just
## uses a simple for loop to add some numbers, but observe how the
## time commands are used to get a starting time before the loop
## and an ending time when it is done. These two times are subtracted
## to get the time it takes to run the code in between.
##
start = time.time()
num = 0
for x in range(1,10000):
num += x
end = time.time()
print("Elapsed time",end-start,"seconds")

Step by stepSolved in 3 steps with 2 images

- python: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter, Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "Luna Lovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger, Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "James Potter, Gryffindor"])['Hermione Granger, Gryffindor',…arrow_forwardIn C ++ You will need to sort whitespace-separated integers using three different sort algorithms (described here: http://theoryapp.com/selection-insertion-and-bubble-sort/ and https://en.wikipedia.org/wiki/Insertion_sort). For each of the three sorting algorithms (selection, insertion, and bubble), your program should output the initial list of numbers as well as after each swap. Note: You should use the Wikipedia entry for insertion sort. Do not perform swaps if they don't change the vector. Example Input: 1 1 6 3 8 4 2 3 9 2 4 Output: 1 Selection Sort 2 1 6 3 8 4 2 3 9 2 4 3 1 2 3 8 4 6 3 9 2 4 4 1 2 2 8 4 6 3 9 3 4 5 1 2 2 3 4 6 8 9 3 4 6 1 2 2 3 3 6 8 9 4 4 7 1 2 2 3 3 4 8 9 6 4 8 1 2 2 3 3 4 4 9 6 8 9 1 2 2 3 3 4 4 6 9 8 10 1 2 2 3 3 4 4 6 8 9 11 Insertion Sort 12 1 6 3 8 4 2 3 9 2 4 13 1 3 6 8 4 2 3 9 2 4 14 1 3 6 4 8 2 3 9 2 4 15 1 3 4 6 8 2 3 9 2 4 16 1 3 4 6 2 8 3 9 2 4 17 1 3 4 2 6 8 3 9 2 4 18 1 3 2 4 6 8 3 9 2 4 19 1 2 3 4 6 8 3…arrow_forwardIn python don't import librariesarrow_forward
- Can you use Python programming language to to this question? Thanksarrow_forwardIn python, Problem Description:You are hosting a party and do not have room to invite all of your friends. You use the following unemotional mathematical method to determine which friends to invite. Number your friends 1, 2, . . . , K and place them in a list in this order. Then perform m rounds. In each round, use a number to determine which friends to remove from the ordered list. The rounds will use numbers r1, r2, . . . , rm. In round i remove all the remaining people in positions that are multiples of ri (that is, ri, 2ri, 3ri, . . .) The beginning of the list is position 1. Output the numbers of the friends that remain after this removal process. Input Specification:The first line of input contains the integer K (1 ≤ K ≤ 100). The second line of input contains the integer m (1 ≤ m ≤ 10), which is the number of rounds of removal. The next m lines each contain one integer. The ith of these lines (1 ≤ i ≤ m) contains ri ( 2 ≤ ri ≤ 100) indicating that every person at a position…arrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forward
- Question in image Please explain the algorithm with the answer. Python programmingarrow_forwardPrompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()arrow_forwardin python pleasearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
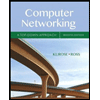
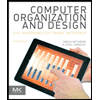
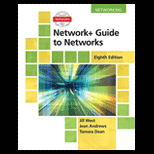
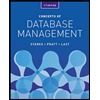
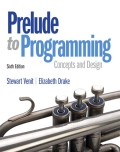
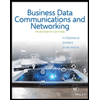