1. In the file script2.js, first change the line in the handleSubmit function which calls createCookies to instead call a function called saveSignUp. 2. Now remove or comment out the function createCookies. 3. At the top of the file, create a new global object variable “signUp” to store the sign-up information.
1. In the file script2.js, first change the line in the handleSubmit function which calls createCookies to instead call a
function called saveSignUp.
2. Now remove or comment out the function createCookies.
3. At the top of the file, create a new global object variable “signUp” to store the sign-up information.
4. Add the following properties to the object: name, email, phone, address, city, state, zip, foodAllergies,
frequency, size and initialize all to a blank string (HINT: review chapter 6)
5. Remove or comment out the following line in the populateInfo() function:
var hiddenInputs = document.querySelectorAll("input[type=hidden]");
6. Define a new local array variable called “fields” in the populateInfo function.
7. Inside the for loop in the same function, replace “hiddenInputs[i].value” with this new array variable.
8. Below the line you just modified, add the following lines to remove and modify encoded characters from the url
string:
var curValue = decodeURIComponent(fields[i]);
curValue = curValue.replace(/\+/g, " ");
fields[i] = curValue;
9. Immediately after the for loop, assign the properties of the signUp object to the values of the fields array you
just captured from the URL string.
10. Create a new function called saveSignUp().
11. Write a line of code to save the value of the textarea in the signUp object field for allergies.
12. Write a line of code to assign the form radio buttons using querySelectorAll to a variable called radioButtons.
13. The following loop should be inserted just after this line. The loop will process each of the 6 radio buttons and
determine whether they are checked, then copy the value of the button into the registration if it is checked.
for(var i = 0; i < radioButtons.length; i++) {
if(i < 3 && radioButtons[i].checked){
signUp.frequency = radioButtons[i].value;
} else if (i > 2 && radioButtons[i].checked) {
signUp.size = radioButtons[i].value;
}
}
14. Add the following lines at the end of the functions to retrieve any existing data in localStorage, then add the new
registration, then save all of the data in localStorage again.
var reg = []; //array to hold signUp objects
if(localStorage.getItem('registrations')) { //registrations array already exists
reg = JSON.parse(localStorage.getItem('registrations')); //get existing registrations
}
reg.push(signUp); //add new registration to end of array
localStorage.setItem('registrations', JSON.stringify(reg)); //store all items again.
Javascript code
"use strict";
var queryArray = [];
function populateInfo() {
if (location.search) {
var queryData = location.search;
var hiddenInputs = document.querySelectorAll("input[type=hidden]");
queryData = queryData.substring(1, queryData.length);
queryArray = queryData.split("&");
for (var i = 0; i < queryArray.length; i++) {
hiddenInputs[i].value = queryArray[i].substring(queryArray[i].lastIndexOf("=") + 1);
}
}
}
function createCookies() {
var formFields = document.querySelectorAll("input[type=hidden],input[type=radio], textarea");
var expireDate = new Date();
expiresDate.setDate(expiresDate.getDate() + 7);
for (var i = 0; i < formFields.length; i++) {
var currentValue = decodeURIComponent(formFields[i].value);
currentValue = currentValue.replace(/\+/g, " ");
document.cookie = formFields[i].name + "=" + currentValue + "; expires=" + expiresDate.toUTCString();
}
}
function handleSubmit(evt) {
if (evt.preventDefault) {
evt.preventDefault();
} else {
evt.returnValue = false;
}
createCookies();
document.getElementsByTagName("form")[0].submit();
}
function createEventListeners() {
var form = document.getElementsByTagName("form")[0];
if (form.addEventListener) {
form.addEventListener("submit", handleSubmit, false);
} else if (form.attachEvent) {
form.attachEvent("onsubmit", handleSubmit);
}
}
function setupPage() {
createEventListeners();
populateInfo();
}
if (window.addEventListener) {
window.addEventListener("load", setupPage, false);
} else if (window.attachEvent) {
window.attachEvent("onload", setupPage);
}

Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 3 images

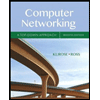
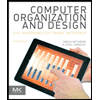
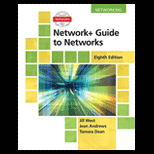
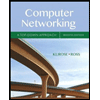
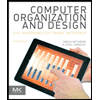
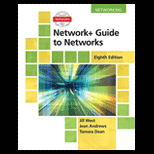
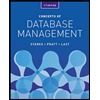
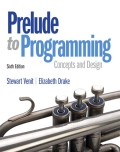
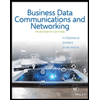