QUESTION IN ATTACHMENT! PLEASE CHECK ----AND ITS HUMBLE REQUEST TO USE ONLY BELOW TEMPLATES WHILE MAKING SOLUTION----- MAIN.CPP #include #include #include #include #include #include #include #include #include "UserBO.cpp" using namespace std; int main() { // fill your code here // declare variables string name; string username; string password; string contactnumber; // create a User class object User obj; // create a object of ofstream class ofstream outfile; outfile.open("example.txt");// open file example.txt UserBO userObj;// create a object of class UserBO userObj for write in file int choice; int i=1; // input from user do{ cout<<"Sample input and output : "<>name; cout<<"Enter the contact number "<>contactnumber; cout<<"Enter the username"<>username; cout<<"Enter the password "<>password; obj.setName(name); obj.setContactnumber(contactnumber); obj.setUsername(username); obj.setPassword(password); userObj.writeUserdetails( outfile ,obj); // wirte in file cout<<"Press 1-> countinue \nPress 0-> exit \nChoice : "; cin>>choice; }while(choice!=0); // close the opened file. outfile.close(); return 0; } UserBO.cpp #include #include #include #include #include #include #include #include"User.cpp" using namespace std; class UserBO{ public: // writeUserdetails() function write data into file void writeUserdetails(ofstream &file,User Obj){ // fill your code here; // write data into the file. file << Obj.getName() << ","<< Obj.getContactnumber() << ","<< Obj.getUsername() << ","<< Obj.getPassword() << endl; cout<<"Datas writtrn in successfully"< #include #include #include #include #include #include using namespace std; class User{ // private variable declare private: string name; string username; string password; string contactnumber; public: // set name void setName(string name){ this->name = name; } // get name string getName(){ return this->name ; } // set username void setUsername(string username){ this->username = username; } /// get username string getUsername(){ return this->username; } // set password void setPassword(string password){ this->password = password; } // get password string getPassword(){ return this->password; } // set contactnumber void setContactnumber(string contactnumber){ this->contactnumber = contactnumber; } // get contactnumber string getContactnumber(){ return this->contactnumber; } };
QUESTION IN ATTACHMENT! PLEASE CHECK
----AND ITS HUMBLE REQUEST TO USE ONLY BELOW TEMPLATES WHILE MAKING SOLUTION-----
MAIN.CPP
#include<iostream> using namespace std; int main() obj.setName(name); |
UserBO.cpp
#include<iostream> class UserBO{ |
User.cpp
#include<iostream> class User{ |
![Write a program to read the GPA of students from users and display the details using dynamic memory
allocation.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class
names, member variable names, and function names should be the same as specified in the problem
statement.
Include the following member function in the Main class
Member Function
void display(float arr[], int size)
Description
This method is used to display the student's GPA details.
In the main method, obtain input from the user in the console and call the display method to display the
GPA details.
Note:
Use a new keyword to create a dynamic array to store the GPA details.
Input Format:
Input consists of roll number and name.
Output Format:
The output displays the roll number and name
Refer sample input and output for formatting specifications.
[All text in bold corresponds to input and rest corresponds to output]
Sample Input and Output 1:
Enter total number of students:
2
Enter GPA of students
7.8
6.7
Students GPA Details
Student1:7.8
Student2:6.7](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8deb4dee-94e2-4016-aac5-3aeff4bdb06f%2F475d95ce-07c5-4939-a7bc-ce92152975f2%2Fpal45nk_processed.png&w=3840&q=75)

Step by step
Solved in 8 steps with 4 images

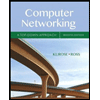
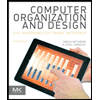
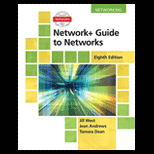
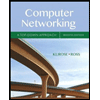
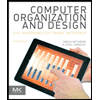
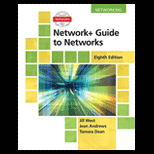
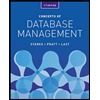
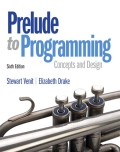
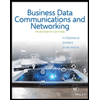