1. In this lab, you will read two files. Firstly, the ‘AccountData.csv’ file has the name and account number. Use each account number to scan the ‘BankData.csv’ file for a matching account number to get a ‘balance’ from the account transactions. You must repeatedly open, read the ‘BankData.csv’ file and close it for each account number in the ‘AccountData.csv’ file unless you wish to store its entire contents in a static array. (More code and may not be enough time to complete.) 2. So, for this lab, read my pseudocode in the given java files FIRST!! 3. ANALYZE my code carefully. 4. Then start coding the solution. I have given the solution to each lab instructor to help you during the time for your specific lab. 5. You will submit in iCollege, ONLY one file: BankDataProcessing.java 6. No need to submit the data files or the BankAccount ‘object’ file. 7. Do NOT MODIFY the ‘BankAccount.java’ file. PLEASE COMPLETE THE JAVA CODE BELOW AND MAKE SURE ITS LiKE THE OUTPUT import java.io.*; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.ArrayList; import java.time.format.DateTimeFormatter; public class BankDataProcessing { static DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MM-dd-yyyy HH:mm"); static ArrayList Accounts = new ArrayList<>(); public static void main(String[] args) { try { Path path = Paths.get("src/AccountData.csv"); readAccounts(path, true); path = Paths.get("src/BankData.csv"); readTransactions(path, true, Accounts); } catch (Exception e) { e.printStackTrace(); } } private static void readAccounts(Path Xpath, boolean bHead) throws IOException { BufferedReader bufferedReader = Files.newBufferedReader(Xpath); try{ // code to process a String 'line' // test for header //process remainder of 'AccountData.csv' //split each line into a string array String[]=line.split.... //name,acctnum //object in the ArrayList of 'Accounts' of objec datatype 'BankAccount' } catch (IOException ioe) { bufferedReader.close(); ioe.printStackTrace(); } finally { bufferedReader.close(); } } private static void readTransactions(Path Xpath, boolean bHead, ArrayList Xaccounts) throws IOException { try{ // one big 'for each' loop to process each 'BankAccount' in ArrayList 'Xaccounts' // test for header // open the file using 'Xpath' and BufferedReader object // split each line into a string array String[]=line.split.... // acctnum,date,transtype,amount // print the "account name and number" // while loop // if "c" then Sting transtype is "Credit" else, "Debit" // total all transactions for balance when current account number (from the 'for each loop') // equals 'Xaccounts.getAcctnum' hint: use Integer.parseInt() // print each transaction with proper "Credit" or "Debit" shown in sample output //end while loop // set balance in the specifice 'Xaccounts' // print the specific 'Xaccounts' element/object (uses the @Override in 'BankAcccount') // CLOSE THE BufferedReader object // end the for loop } } catch (IOException ioe) { ioe.printStackTrace(); } } } public class BankAccount { private String name; private double balance; private int acctnum; public BankAccount(String Xname, int Xacct) { this.name = Xname; this.acctnum = Xacct; } public void setBalance(double Xbalance) { this.balance = Xbalance; } public String getAcctname() { return this.name; } public int getAcctnum(){ return this.acctnum; } @Override public String toString() { return this.name+", has balance: $"+this.balance; } } SAMPLE OUTPUT FROM GIVEN DATA FILE: Account for Snoopy, 45655 Transaction date: 10-01-2021, Credit= 533 Transaction date: 10-03-2021, Debit= 10 Transaction date: 10-12-2021, Credit= 33 Transaction date: 10-01-2021, Debit= 53 Transaction date: 10-03-2021, Credit= 100 Transaction date: 10-12-2021, Debit= 133 Snoopy, has balance: $470.0 ------------------------------------------------------------ Account for Charlie Brown, 45622 Transaction date: 10-12-2021, Credit= 330 Transaction date: 10-04-2021, Debit= 100 Transaction date: 10-12-2021, Debit= 30 Transaction date: 10-12-2021, Debit= 130 Transaction date: 10-04-2021, Credit= 100 Transaction date: 10-12-2021, Credit= 30 Charlie Brown, has balance: $200.0 ------------------------------------------------------------ Account for Linus, 45611 Transaction date: 10-01-2021, Credit= 255 Transaction date: 10-03-2021, Debit= 25 Transaction date: 10-10-2021, Debit= 25 Transaction date: 10-01-2021, Debit= 155 Transaction date: 10-03-2021, Credit= 55 Transaction date: 10-10-2021, Credit= 95 Linus, has balance: $200.0 ------------------------------------------------------------ Account for Lucy, 45600 Transaction date: 10-01-2021, Credit= 405 Transaction date: 10-02-2021, Debit= 280 Transaction date: 10-11-2021, Credit= 92 Transaction date: 10-01-2021, Debit= 105 Transaction date: 10-02-2021, Credit= 20 Transaction date: 10-11-2021, Debit= 212 Lucy, has balance: $-80.0 ------------------------------------------------------------
1. In this lab, you will read two files. Firstly, the ‘AccountData.csv’ file has the name and account number.
Use each account number to scan the ‘BankData.csv’ file for a matching account number to get a ‘balance’
from the account transactions. You must repeatedly open, read the ‘BankData.csv’ file and close it for each
account number in the ‘AccountData.csv’ file unless you wish to store its entire contents in a static array.
(More code and may not be enough time to complete.)
2. So, for this lab, read my pseudocode in the given java files FIRST!!
3. ANALYZE my code carefully.
4. Then start coding the solution. I have given the solution to each lab instructor to help you during the time
for your specific lab.
5. You will submit in iCollege, ONLY one file: BankDataProcessing.java
6. No need to submit the data files or the BankAccount ‘object’ file.
7. Do NOT MODIFY the ‘BankAccount.java’ file.
PLEASE COMPLETE THE JAVA CODE BELOW AND MAKE SURE ITS LiKE THE OUTPUT
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.time.format.DateTimeFormatter;
public class BankDataProcessing {
static DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MM-dd-yyyy HH:mm");
static ArrayList<BankAccount> Accounts = new ArrayList<>();
public static void main(String[] args) {
try {
Path path = Paths.get("src/AccountData.csv");
readAccounts(path, true);
path = Paths.get("src/BankData.csv");
readTransactions(path, true, Accounts);
}
catch (Exception e) {
e.printStackTrace();
}
}
private static void readAccounts(Path Xpath, boolean bHead) throws IOException {
BufferedReader bufferedReader = Files.newBufferedReader(Xpath);
try{
// code to process a String 'line'
// test for header
//process remainder of 'AccountData.csv'
//split each line into a string array String[]=line.split.... //name,acctnum
//object in the ArrayList of 'Accounts' of objec datatype 'BankAccount'
}
catch (IOException ioe) {
bufferedReader.close();
ioe.printStackTrace();
}
finally {
bufferedReader.close();
}
}
private static void readTransactions(Path Xpath, boolean bHead, ArrayList<BankAccount> Xaccounts) throws IOException {
try{
// one big 'for each' loop to process each 'BankAccount' in ArrayList 'Xaccounts'
// test for header
// open the file using 'Xpath' and BufferedReader object
// split each line into a string array String[]=line.split.... // acctnum,date,transtype,amount
// print the "account name and number"
// while loop
// if "c" then Sting transtype is "Credit" else, "Debit"
// total all transactions for balance when current account number (from the 'for each loop')
// equals 'Xaccounts.getAcctnum' hint: use Integer.parseInt()
// print each transaction with proper "Credit" or "Debit" shown in sample output
//end while loop
// set balance in the specifice 'Xaccounts'
// print the specific 'Xaccounts' element/object (uses the @Override in 'BankAcccount')
// CLOSE THE BufferedReader object
// end the for loop
}
}
catch (IOException ioe) {
ioe.printStackTrace();
}
}
}
public class BankAccount {
private String name;
private double balance;
private int acctnum;
public BankAccount(String Xname, int Xacct) {
this.name = Xname;
this.acctnum = Xacct;
}
public void setBalance(double Xbalance) {
this.balance = Xbalance;
}
public String getAcctname() {
return this.name;
}
public int getAcctnum(){
return this.acctnum;
}
@Override
public String toString() {
return this.name+", has balance: $"+this.balance;
}
}
SAMPLE OUTPUT FROM GIVEN DATA FILE:
Account for Snoopy, 45655
Transaction date: 10-01-2021, Credit= 533
Transaction date: 10-03-2021, Debit= 10
Transaction date: 10-12-2021, Credit= 33
Transaction date: 10-01-2021, Debit= 53
Transaction date: 10-03-2021, Credit= 100
Transaction date: 10-12-2021, Debit= 133
Snoopy, has balance: $470.0
------------------------------------------------------------
Account for Charlie Brown, 45622
Transaction date: 10-12-2021, Credit= 330
Transaction date: 10-04-2021, Debit= 100
Transaction date: 10-12-2021, Debit= 30
Transaction date: 10-12-2021, Debit= 130
Transaction date: 10-04-2021, Credit= 100
Transaction date: 10-12-2021, Credit= 30
Charlie Brown, has balance: $200.0
------------------------------------------------------------
Account for Linus, 45611
Transaction date: 10-01-2021, Credit= 255
Transaction date: 10-03-2021, Debit= 25
Transaction date: 10-10-2021, Debit= 25
Transaction date: 10-01-2021, Debit= 155
Transaction date: 10-03-2021, Credit= 55
Transaction date: 10-10-2021, Credit= 95
Linus, has balance: $200.0
------------------------------------------------------------
Account for Lucy, 45600
Transaction date: 10-01-2021, Credit= 405
Transaction date: 10-02-2021, Debit= 280
Transaction date: 10-11-2021, Credit= 92
Transaction date: 10-01-2021, Debit= 105
Transaction date: 10-02-2021, Credit= 20
Transaction date: 10-11-2021, Debit= 212
Lucy, has balance: $-80.0
------------------------------------------------------------

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

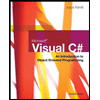
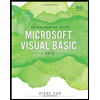
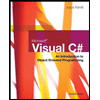
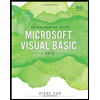