1. With the partial implementation of the "Merge-Sort" algorithm (shown below), namely, merge( ) and mergeSort(), build a test program that sorts an unsorted array of the size of 20. You may need Queue, LinkedQueue or any other classes and interfaces to import. /** Merge contents of arrays S1 and S2 into properly sized array S. */ public static void merge(K[] s1, K[] S2, K[] s, Comparator comp) { int i = 0, j = 0; while (i + j < S.length) { if (j == S2.1ength || (i < s1. length && comp.compare(S1[i], S2[j]) < e)) S[i+j] = S1[i++]; else // copy ith element of S1 and increment i // copy ith element of S2 and increment j S[i+j] = S2[j++]; } } /** Merge-sort contents of array S. */ public static void mergeSort (K[] S, Comparator comp) { int n = S.length; if (n < 2) return; // divide int mid = n/2; K[] s1 = Arrays.copy0fRange (S, e, mid); K[] s2 = Arrays.copy0fRange (S, mid, n); // conquer (with recursion) mergeSort(S1, comp); mergeSort(S2, comp); // merge results merge (S1, S2, s, comp); // array is trivially sorted // copy of first half // copy of second half // sort copy of first half // sort copy of second half // merge sorted halves back into original
1. With the partial implementation of the "Merge-Sort" algorithm (shown below), namely, merge( ) and mergeSort(), build a test program that sorts an unsorted array of the size of 20. You may need Queue, LinkedQueue or any other classes and interfaces to import. /** Merge contents of arrays S1 and S2 into properly sized array S. */ public static void merge(K[] s1, K[] S2, K[] s, Comparator comp) { int i = 0, j = 0; while (i + j < S.length) { if (j == S2.1ength || (i < s1. length && comp.compare(S1[i], S2[j]) < e)) S[i+j] = S1[i++]; else // copy ith element of S1 and increment i // copy ith element of S2 and increment j S[i+j] = S2[j++]; } } /** Merge-sort contents of array S. */ public static void mergeSort (K[] S, Comparator comp) { int n = S.length; if (n < 2) return; // divide int mid = n/2; K[] s1 = Arrays.copy0fRange (S, e, mid); K[] s2 = Arrays.copy0fRange (S, mid, n); // conquer (with recursion) mergeSort(S1, comp); mergeSort(S2, comp); // merge results merge (S1, S2, s, comp); // array is trivially sorted // copy of first half // copy of second half // sort copy of first half // sort copy of second half // merge sorted halves back into original
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please answer the following question attached below(use java ) and also add necessary comments for understanding
![1. With the partial implementation of the "Merge-Sort" algorithm (shown below),
namely, merge() and mergeSort(), build a test program that sorts an unsorted array
of the size of 20. You may need Queue, LinkedQueue or any other classes and
interfaces to import.
/** Merge contents of arrays S1 and S2 into properly sized array S. */
public static <K> void merge (K[] S1, K[] s2, K[] S, comparator<K> comp) {
int i = 0, j = 0;
while (i + j < S.length) {
if (j == S2.1length || (i < S1.length && comp.compare(S1[i], S2[j]) < 0))
S[i+j] = S1[i++];
else
// copy ith element of S1 and increment i
S[i+j] = S2[j++];
}
}
/** Merge-sort contents of array S. */
public static <K> void mergeSort (K[] S, Comparator<K> comp) {
int n = S.length;
if (n < 2) return;
// divide
int mid = n/2;
// copy jth element of S2 and increment j
// array is trivially sorted
// copy of first half
// copy of second half
K[] s1 = Arrays.copy0fRange (S, 0, mid);
K[] s2 = Arrays.copy0fRange (S, mid, n);
// conquer (with recursion)
mergeSort(S1, comp);
mergeSort(S2, comp);
// merge results
merge(S1, S2, S, comp);
// sort copy of first half
// sort copy of second half
// merge sorted halves back into original](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F159e8188-60a2-4e95-8ad8-f5d0b7e7fd6d%2F8a4bff79-4c68-4785-a5ce-96ba9b2ac4b5%2Feooargs_processed.jpeg&w=3840&q=75)
Transcribed Image Text:1. With the partial implementation of the "Merge-Sort" algorithm (shown below),
namely, merge() and mergeSort(), build a test program that sorts an unsorted array
of the size of 20. You may need Queue, LinkedQueue or any other classes and
interfaces to import.
/** Merge contents of arrays S1 and S2 into properly sized array S. */
public static <K> void merge (K[] S1, K[] s2, K[] S, comparator<K> comp) {
int i = 0, j = 0;
while (i + j < S.length) {
if (j == S2.1length || (i < S1.length && comp.compare(S1[i], S2[j]) < 0))
S[i+j] = S1[i++];
else
// copy ith element of S1 and increment i
S[i+j] = S2[j++];
}
}
/** Merge-sort contents of array S. */
public static <K> void mergeSort (K[] S, Comparator<K> comp) {
int n = S.length;
if (n < 2) return;
// divide
int mid = n/2;
// copy jth element of S2 and increment j
// array is trivially sorted
// copy of first half
// copy of second half
K[] s1 = Arrays.copy0fRange (S, 0, mid);
K[] s2 = Arrays.copy0fRange (S, mid, n);
// conquer (with recursion)
mergeSort(S1, comp);
mergeSort(S2, comp);
// merge results
merge(S1, S2, S, comp);
// sort copy of first half
// sort copy of second half
// merge sorted halves back into original
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
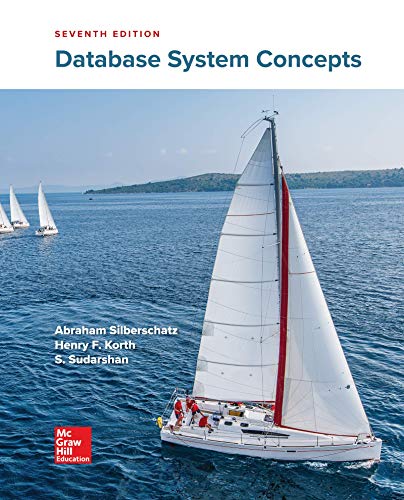
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
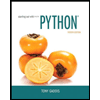
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
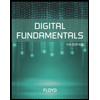
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
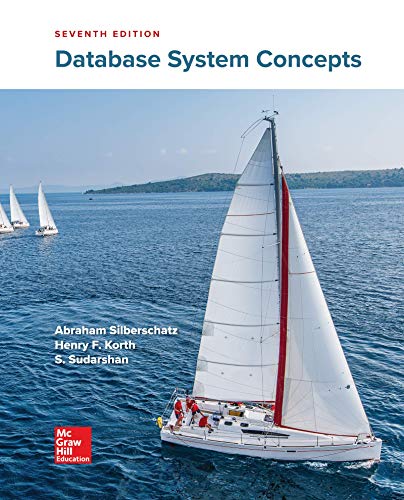
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
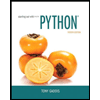
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
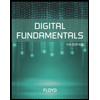
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
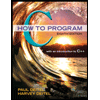
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
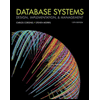
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
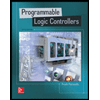
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education