3. lookup_friends_heights This function takes a friends database (a list of dictionaries, like the previous functions) and a list of friends names, and it returns a list of heights for the specified friends. If a given friend's name is not present in the friends database, we put a None in the corresponding slot. We assume that there will be at most one friend with a given name in the list. You may want to use nested for-loops for this function. Sample calls should look like this. (»» lookup_friends_heights([{"name":"todd","height":170},{"name":"sarah","height":165}, {"name":"dweezil","height":175}], ["dweezil", "sarah"]) [175, 165] >» lookup_friends_heights([{"name":"todd","height":170},{"name":"sarah","height":165}, {"name":"dweezil","height":175}], ["sarah", "eliza", "dweezil"]) [165, None, 175] 4. class definition for Friend For the next version of the friends database (coming soon!), we want to use classes rather than dictionaries. Define a Friend class , where each Friend object will store that friend's name, height and favorite foods. The class should have an _init_ method that takes in that friend's name and height. It should have an add_favorite_food method that takes a string and adds that string to a set of favorite foods, and a number_of_favorite_foods method that returns the number of favorite foods for that friend. Sample runs should look like: >>> friend1 = Friend("dweezil", 175) >>> friend1.add_favorite_food("borscht") >>> friend1.add_favorite_food("borscht")
3. lookup_friends_heights This function takes a friends database (a list of dictionaries, like the previous functions) and a list of friends names, and it returns a list of heights for the specified friends. If a given friend's name is not present in the friends database, we put a None in the corresponding slot. We assume that there will be at most one friend with a given name in the list. You may want to use nested for-loops for this function. Sample calls should look like this. (»» lookup_friends_heights([{"name":"todd","height":170},{"name":"sarah","height":165}, {"name":"dweezil","height":175}], ["dweezil", "sarah"]) [175, 165] >» lookup_friends_heights([{"name":"todd","height":170},{"name":"sarah","height":165}, {"name":"dweezil","height":175}], ["sarah", "eliza", "dweezil"]) [165, None, 175] 4. class definition for Friend For the next version of the friends database (coming soon!), we want to use classes rather than dictionaries. Define a Friend class , where each Friend object will store that friend's name, height and favorite foods. The class should have an _init_ method that takes in that friend's name and height. It should have an add_favorite_food method that takes a string and adds that string to a set of favorite foods, and a number_of_favorite_foods method that returns the number of favorite foods for that friend. Sample runs should look like: >>> friend1 = Friend("dweezil", 175) >>> friend1.add_favorite_food("borscht") >>> friend1.add_favorite_food("borscht")
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter13: Structures
Section13.5: Dynamic Data Structure Allocation
Problem 3E
Related questions
Question
![3. lookup_friends_heights
This function takes a friends database (a list of dictionaries, like the previous functions) and a list of friends names, and
it returns a list of heights for the specified friends. If a given friend's name is not present in the friends database, we put
a None in the corresponding slot. We assume that there will be at most one friend with a given name in the list. You
may want to use nested for-loops for this function.
Sample calls should look like this.
>>» lookup_friends_heights([{"name":"todd", "height":170},{"name":"sarah","height":165},
{"name":"dweezil","height":175}], ["dweezil", "sarah"])
[175, 165]
>>> lookup_friends_heightsC[{"name":"todd","height":170},{"name":"sarah","height":165},
{"name":"dweezil","height":175}], ["sarah", "eliza", "dweezil"])
[165, None, 175]
4. class definition for Friend
For the next version of the friends database (coming soon!), we want to use classes rather than dictionaries. Define a
Friend class , where each Friend object will store that friend's name, height and favorite foods. The class should have an
_init_ method that takes in that friend's name and height. It should have an add_favorite_food method that takes a
string and adds that string to a set of favorite foods, and a number_of_favorite_foods method that returns the number
of favorite foods for that friend.
Sample runs should look like:
>>> friend1 = Friend("dweezil", 175)
>>> friend1.add_favorite_food("borscht")
>>> friend1.add_favorite_food("borscht")
>>> friend1.add_favorite_food("bagel")
>>> friend1.add_favorite_food("banana")
>>> friend1.number_of_favorite_foods()
3
>>> friend1.name
'dweezil'
>>> friend1.height
175](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc06466e0-b6af-451e-ad3a-a7add978ace4%2F8876d91c-eb7a-4292-affd-99de214a2562%2F9fgkgbi_processed.png&w=3840&q=75)
Transcribed Image Text:3. lookup_friends_heights
This function takes a friends database (a list of dictionaries, like the previous functions) and a list of friends names, and
it returns a list of heights for the specified friends. If a given friend's name is not present in the friends database, we put
a None in the corresponding slot. We assume that there will be at most one friend with a given name in the list. You
may want to use nested for-loops for this function.
Sample calls should look like this.
>>» lookup_friends_heights([{"name":"todd", "height":170},{"name":"sarah","height":165},
{"name":"dweezil","height":175}], ["dweezil", "sarah"])
[175, 165]
>>> lookup_friends_heightsC[{"name":"todd","height":170},{"name":"sarah","height":165},
{"name":"dweezil","height":175}], ["sarah", "eliza", "dweezil"])
[165, None, 175]
4. class definition for Friend
For the next version of the friends database (coming soon!), we want to use classes rather than dictionaries. Define a
Friend class , where each Friend object will store that friend's name, height and favorite foods. The class should have an
_init_ method that takes in that friend's name and height. It should have an add_favorite_food method that takes a
string and adds that string to a set of favorite foods, and a number_of_favorite_foods method that returns the number
of favorite foods for that friend.
Sample runs should look like:
>>> friend1 = Friend("dweezil", 175)
>>> friend1.add_favorite_food("borscht")
>>> friend1.add_favorite_food("borscht")
>>> friend1.add_favorite_food("bagel")
>>> friend1.add_favorite_food("banana")
>>> friend1.number_of_favorite_foods()
3
>>> friend1.name
'dweezil'
>>> friend1.height
175
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
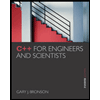
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
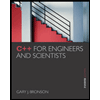
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage