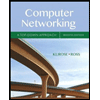
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Add 3 parameters to each function in the code below.
********************************************* Code starts here **************************************************
Module.py
#Defination to sort the list
def sort(listNum):
sortedList = []
#While loop will run until the listNum don't get null
while(len(listNum) != 0 ):
#Set the min as first element in list
min = listNum[0]
#iterate over the list to compare every element with num
for ele in listNum:
#If element is less than min
if ele < min:
#Then set min as element
min = ele
#append the sorted element in list
sortedList.append(min)
#Remove the sorted element from the list
listNum.remove(min)
return sortedList
#Function to find the sum of all elements in list
def SumOfList(listNum):
#Set the sum as zero
sum =0
#Iterate over the list to get every element
for ele in listNum:
#Keep adding the element to the sum variable
sum = sum + ele
#returnt the sum
return sum
#Function to find the max of the list
def listMax(listNum):
#To store the max
max =0
#Iterate over the list
for ele in listNum:
#If element is greater than max then max = element
if(ele>max):
max = ele
#Return the max
return max
***************************************************************************************
Main .py
#Import the module file having the 3 functions
import module
import random
def main():
#use the random module to create the list of random integers
#To store the random numbers in range 0 to 10
randomList = []
#Create the loop to create the list of 5 random integers
for i in range(0,5):
#Use randint to create the random integer
num = random.randint(0,10)
#Add the random integer into the list
randomList.append(num)
#Now create the menu to let user choose from what to perform on the random list
print("Random list created : ",randomList,"\nSpecify what operations you wanna perform on the list")
print("1:Sorting\n2:Sum of all elements \n3:Maximum\nChooce 1-3 : ",end = "")
#Now take input from the user
choice = int(input())
#Now use decision structures to decide which function to call from module.py
if(choice == 1 ):
#Print the sorted list
print("Sorted list as follows : " ,module.sort(randomList))
elif(choice == 2):
#Print the sum of all elements in list
print("The sum of all elements in list : ",module.SumOfList(randomList))
elif(choice == 3):
#print the maximum of list
print("The maximum of list is : ",module.listMax(randomList))
#When the __name__ will be ___main__ the main function will be called.
if __name__ == "__main__":
main()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- tutorial. B4 Amend your code to keep track of the total number of moves in the game, by writing a function PlayHanoi2 (pos, a, b, c,n, j) which has an additional input j (which will be zero on the initial input) to serve as a move-counter, and which outputs suitably modified (pos, j). Define a function WholeGame2 (n) which runs PlayHanoi2 on a suitable starting configuration. This might be tricky depending on how you coded B3: the danger is that the counting variable j will assume different val- ues in different subroutines. Make sure you keep careful track of the current game position and number of moves throughout your function.arrow_forwardWhat is a stub? Group of answer choices A stub is an inline function with a single line that simply sends a "Hello" message to others, indicating that it has been activated. A stub is a function that has an empty body. It will be developed later. A stub is an inline function with a single line that simply increases the pass in value by one. A stub is a function with a single line that simply prints the name of the function, indicating that it has been activated. A stub is a short function that will generate an error message.arrow_forwardDefine a function print_repeated() that takes two parameters and outputs the first parameter the second parameter number of times on one line separated and ending with a space. The function does not return any value.arrow_forward
- Call by value in programming means that a copy of variable value is pased from function calling part to function definition part. Call by reference means that an address of variables are passed from calling part to function definition. arrow_forward Step 2 Call by reference is used to avoid extra memory usage because in call by reference same variables addresses are passed. Any changes made will reflect back. So it doesn't require extra variable to hold data. Call by value in programming is used where memory storage is not concern. Scenario in which actual value must not be disturbed then in these type of cases, call by value is used. We can't use only one because user requirement changes. Sometimes user tella actual data should be preserved and he/she want any data processing, in such scenario programmers use call by value. Some users only want calculated result. In such cases, programmers use call by reference to preserve memory usage. So it is totally dependent on user…arrow_forwardList all cases when inline functions have the opposite effect.arrow_forwardExplain what a piecewise-defined function is and why it is used. Give an example.arrow_forward
- Python Suppose scDict maps names to test scores. Print each name/score on a separate line Write a function calcStats that calculates and RETURNS the average, highest, and lowest of the scores. The function should NOT print anything. Ex: scDict ={“Joe”:70, “Bob”:90, “Sue”: 95} Output: Joe 70Bob 90Sue 95Average: 85High Score: 95Low Score: 703. Ask the user to enter a name and a score, and put that entry into the scDict. Ask the user to enter a name to delete and remove that entry Check to see if the dictionary contains a key “Mary” and print “YES” if it does. 6. Print the dictionary, sorted by namearrow_forward. Initialize please.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
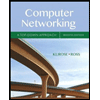
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
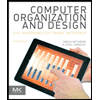
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
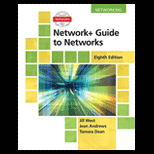
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
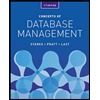
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
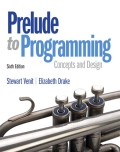
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
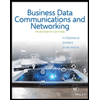
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY