7. Dynamic MathStack The MathStack class shown in this chapter has only two member functions: add and sub. Write the following additional member functions: Function Description mult Pops the top two values off the stack, multiplies them, and pushes their product onto the stack. div Pops the top two values off the stack, divides the second value by the first, and pushes the quotient onto the stack. addAll Pops all values off the stack, adds them, and pushes their sum onto the stack. multA11 Pops all values off the stack, multiplies them, and pushes their prod- uct onto the stack. Demonstrate the class with a driver program. here is the extention file please use it. // Specification file for the IntStack class #ifndef INTSTACK_H #define INTSTACK_H class IntStack { private: int *stackArray; // Pointer to the stack array int stackSize; // The stack size int top; // Indicates the top of the stack public: // Constructor IntStack(int); // Copy constructor IntStack(const IntStack &); // Destructor ~IntStack(); // Stack operations void push(int); void pop(int &); bool isFull() const; bool isEmpty() const; }; #endif the second file // Specification file for the MathStack class #ifndef MATHSTACK_H #define MATHSTACK_H #include "IntStack.h" class MathStack : public IntStack { public: // Constructor MathStack(int s) : IntStack(s) {} // MathStack operations void add(); void sub(); }; #endif
7. Dynamic MathStack The MathStack class shown in this chapter has only two member functions: add and sub. Write the following additional member functions: Function Description mult Pops the top two values off the stack, multiplies them, and pushes their product onto the stack. div Pops the top two values off the stack, divides the second value by the first, and pushes the quotient onto the stack. addAll Pops all values off the stack, adds them, and pushes their sum onto the stack. multA11 Pops all values off the stack, multiplies them, and pushes their prod- uct onto the stack. Demonstrate the class with a driver program.
here is the extention file please use it.
// Specification file for the IntStack class
#ifndef INTSTACK_H
#define INTSTACK_H
class IntStack
{
private:
int *stackArray; // Pointer to the stack array
int stackSize; // The stack size
int top; // Indicates the top of the stack
public:
// Constructor
IntStack(int);
// Copy constructor
IntStack(const IntStack &);
// Destructor
~IntStack();
// Stack operations
void push(int);
void pop(int &);
bool isFull() const;
bool isEmpty() const;
};
#endif
the second file
// Specification file for the MathStack class
#ifndef MATHSTACK_H
#define MATHSTACK_H
#include "IntStack.h"
class MathStack : public IntStack
{
public:
// Constructor
MathStack(int s) : IntStack(s) {}
// MathStack operations
void add();
void sub();
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

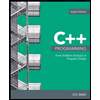
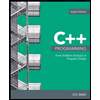