9.7 (The Account class) Design a clasS named Account that contains: I A private int data field named id for the account (default 0). I A private double data field named balance for the account (default 0). IA private double data field named annualInterestRate that stores the current interest rate (default 0). Assume all accounts have the same interest rate. A private Date data field named dateCreated that stores the date when the account was created. A no-arg constructor that creates a default account. I A constructor that creates an account with the specified id and initial balance. 1 The accessorandmutatormethodsfor id,balance, andannualInterestRate. 1 The accessor method for dateCreated. I A method named getMonthlyInterestRate() that returns the monthly interest rate. I A method named getMonthlyInterest () that returns the monthly interest. IA method named withdraw that withdraws a specified amount from the account. IA method named deposit that denosits a specified amount to the account
9.7 (The Account class) Design a clasS named Account that contains: I A private int data field named id for the account (default 0). I A private double data field named balance for the account (default 0). IA private double data field named annualInterestRate that stores the current interest rate (default 0). Assume all accounts have the same interest rate. A private Date data field named dateCreated that stores the date when the account was created. A no-arg constructor that creates a default account. I A constructor that creates an account with the specified id and initial balance. 1 The accessorandmutatormethodsfor id,balance, andannualInterestRate. 1 The accessor method for dateCreated. I A method named getMonthlyInterestRate() that returns the monthly interest rate. I A method named getMonthlyInterest () that returns the monthly interest. IA method named withdraw that withdraws a specified amount from the account. IA method named deposit that denosits a specified amount to the account
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter9: Records (struct)
Section: Chapter Questions
Problem 12SA
Related questions
Question
![9.7
(The Account class) Design a class named Account that contains:
A private int data field named id for the account (default 0).
I A private double data field named balance for the account (default 0).
A private double data field named annua]InterestRate that stores the
current interest rate (default 0). Assume all accounts have the same interest
rate.
A private Date data field named dateCreated that stores the date when the
account was created.
A no-arg constructor that creates a default account.
A constructor that creates an account with the specified id and initial balance.
1 Theaccessorandmutatormethodsfor id,balance, andannualInterestRate.
The accessor method for dateCreated.
I A method named getMonthlyInterestRate() that returns the monthly
interest rate.
A method named getMonthlyInterest() that returns the monthly interest.
A method named withdraw that withdraws a specified amount from the
account.
I A method named deposit that deposits a specified amount to the account.
Draw the UML diagram for the class and then implement the class. (Hint: The
method getMonthlyInterest() is to return monthly interest, not the interest rate.
Monthly interest is balance * monthlyInterestRate. monthlyInterestRate
is annualInterestRate / 12. Note that annualInterestRate is a percentage,
e.g., like 4.5%. You need to divide it by 100.)
Write a test program that creates an Account object with an account ID of 1122,
a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw
method to withdraw $2,500, use the deposit method to deposit $3,000, and print
the balance, the monthly interest, and the date when this account was created.
VideoNote
The Fan class](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8dbcbf0b-9123-4a1c-9796-89fa34227664%2Fece99dfe-8295-47fb-b30f-2d2ffcd96215%2Fofrdygn_processed.jpeg&w=3840&q=75)
Transcribed Image Text:9.7
(The Account class) Design a class named Account that contains:
A private int data field named id for the account (default 0).
I A private double data field named balance for the account (default 0).
A private double data field named annua]InterestRate that stores the
current interest rate (default 0). Assume all accounts have the same interest
rate.
A private Date data field named dateCreated that stores the date when the
account was created.
A no-arg constructor that creates a default account.
A constructor that creates an account with the specified id and initial balance.
1 Theaccessorandmutatormethodsfor id,balance, andannualInterestRate.
The accessor method for dateCreated.
I A method named getMonthlyInterestRate() that returns the monthly
interest rate.
A method named getMonthlyInterest() that returns the monthly interest.
A method named withdraw that withdraws a specified amount from the
account.
I A method named deposit that deposits a specified amount to the account.
Draw the UML diagram for the class and then implement the class. (Hint: The
method getMonthlyInterest() is to return monthly interest, not the interest rate.
Monthly interest is balance * monthlyInterestRate. monthlyInterestRate
is annualInterestRate / 12. Note that annualInterestRate is a percentage,
e.g., like 4.5%. You need to divide it by 100.)
Write a test program that creates an Account object with an account ID of 1122,
a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw
method to withdraw $2,500, use the deposit method to deposit $3,000, and print
the balance, the monthly interest, and the date when this account was created.
VideoNote
The Fan class
Expert Solution

Step 1
import java.util.Date; | |
public class Account { | |
// Data fields | |
private int id; | |
private double balance; | |
private static double annualInterestRate; | |
private Date dateCreated; | |
// Constructors | |
/** Creates a default account */ | |
Account() { | |
this(0, 0); | |
} | |
/** Creates an account with the specified id and initial balance */ | |
Account(int id, double balance) { | |
this.id = id; | |
this.balance = balance; | |
annualInterestRate = 0; | |
dateCreated = new Date(); | |
} | |
// Mutator methods | |
/** Set id */ | |
public void setId(int id) { | |
this.id = id; | |
} | |
/** Set balance */ | |
public void setBalance(double balance) { | |
this.balance = balance; | |
} | |
/** Set annualInterestRate */ | |
public void setAnnualInterestRate(double annualInterestRate) { | |
this.annualInterestRate = annualInterestRate; | |
} | |
// Accessor methods | |
/** Return id */ | |
public int getId() { | |
return id; | |
} | |
/** Return balance */ | |
public double getBalance() { | |
return balance; | |
} | |
/** Return annualInterestRate */ | |
public double getAnnualInterestRate() { | |
return annualInterestRate; | |
} | |
/** Return dateCreated */ | |
public String getDateCreated() { | |
return dateCreated.toString(); | |
} | |
/** Return monthly interest rate */ | |
public double getMonthlyInterestRate() { | |
return annualInterestRate / 12; | |
} | |
// Methods | |
/** Return monthly interest */ | |
public double getMonthlyInterest() { | |
return balance * (getMonthlyInterestRate() / 100); | |
} | |
/** Decrease balance by amount */ | |
public void withdraw(double amount) { | |
balance -= amount; | |
} | |
/** Increase balance by amount */ | |
public void deposit(double amount) { | |
balance += amount; | |
} | |
/** Return a String decription of Account class */ | |
public String toString() { | |
return "\nAccount ID: " + id + "\nDate created: " + getDateCreated() | |
+ "\nBalance: $" + String.format("%.2f", balance) + | |
"\nMonthly interest: $" + String.format("%.2f", getMonthlyInterest()); | |
} | |
} |
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
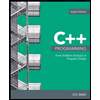
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
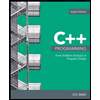
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning