A store owner keeps a record of daily cash transactions in a text file. Each line contains three items: The invoice number, the cash amount, and the letter P if the amount was paid or R if it was received. Items are separated by spaces. Assume all input files are in this valid format. Write a method balance which takes as parameters the amount of cash at the beginning and end of the day, and the name of the file, checks whether the actual amount of cash at the end of the day equals the expected value, and returns the result as a string. Use this template: BalanceTransactions.java You must catch any FileNotFoundException that is thrown and print out a message in the format: "File: [filename] not found", e.g., File: transactions1.txt not found Example: balance (0, 55.55, "res/transactions1.txt , "); // returns "End of day total is properly balanced." balance (25, 55.55, "res/transactions1.txt "); // returns "End of day total is not balanced." balance (0, 55.55, "res/doesnotexist.txt"); // prints out "File: res/doesnotexist.txt not found"
A store owner keeps a record of daily cash transactions in a text file. Each line contains three items: The invoice number, the cash amount, and the letter P if the amount was paid or R if it was received. Items are separated by spaces. Assume all input files are in this valid format. Write a method balance which takes as parameters the amount of cash at the beginning and end of the day, and the name of the file, checks whether the actual amount of cash at the end of the day equals the expected value, and returns the result as a string. Use this template: BalanceTransactions.java You must catch any FileNotFoundException that is thrown and print out a message in the format: "File: [filename] not found", e.g., File: transactions1.txt not found Example: balance (0, 55.55, "res/transactions1.txt , "); // returns "End of day total is properly balanced." balance (25, 55.55, "res/transactions1.txt "); // returns "End of day total is not balanced." balance (0, 55.55, "res/doesnotexist.txt"); // prints out "File: res/doesnotexist.txt not found"
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 6PE
Related questions
Question
Java. Please use the template in the picture, thank you!
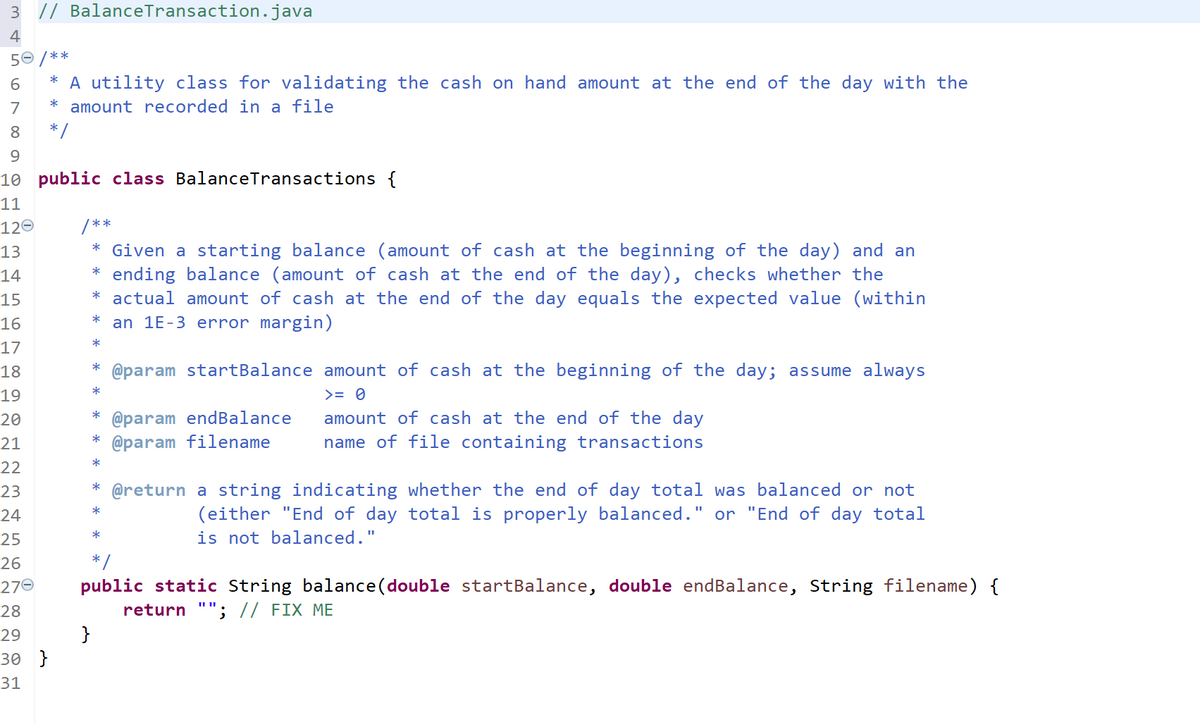
Transcribed Image Text:3 // BalanceTransaction.java
4
50 /**
* A utility class for validating the cash on hand amount at the end of the day with the
* amount recorded in a file
* /
6.
7
8
9.
10 public class BalanceTransactions {
11
120
/**
* Given a starting balance (amount of cash at the beginning of the day) and an
* ending balance (amount of cash at the end of the day), checks whether the
* actual amount of cash at the end of the day equals the expected value (within
an 1E-3 error margin)
13
14
15
*
16
*
17
18
* @param startBalance amount of cash at the beginning of the day; assume always
*
19
>= 0
* @param endBalance
* @param filename
20
amount of cash at the end of the day
21
name of file containing transactions
*
22
23
* @return a string indicating whether the end of day total was balanced or not
(either "End of day total is properly balanced." or "End of day total
is not balanced."
*
24
*
25
26
* /
public static String balance (double startBalance, double endBalance, String filename) {
return ""; // FIX ME
}
270
28
29
30 }
31
![A store owner keeps a record of daily cash transactions in a text file. Each line contains three items: The
invoice number, the cash amount, and the letter P if the amount was paid or R if it was received. Items are
separated by spaces. Assume all input files are in this valid format.
Write a method balance which takes as parameters the amount of cash at the beginning and end of the
day, and the name of the file, checks whether the actual amount of cash at the end of the day equals the
expected value, and returns the result as a string. Use this template: BalanceTransactions.java
You must catch any FileNotFoundException that is thrown and print out a message in the format:
"File: [filename] not found", e.g., File: transactions1.txt not found
Example:
balance (0, 55.55, "res/transactions1.txt , "); // returns "End of day total
is properly balanced."
balance (25, 55.55, "res/transactions1.txt ¿ "); // returns "End of day
total is not balanced."
balance (0, 55.55, "res/doesnotexist.txt"); // prints out "File:
res/doesnotexist.txt not found"](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe5f558a7-14fc-4024-84d6-4debb1adc6f6%2Ffeeaf24e-4510-49e5-873e-fcd4817380fc%2Fp3z9nf_processed.jpeg&w=3840&q=75)
Transcribed Image Text:A store owner keeps a record of daily cash transactions in a text file. Each line contains three items: The
invoice number, the cash amount, and the letter P if the amount was paid or R if it was received. Items are
separated by spaces. Assume all input files are in this valid format.
Write a method balance which takes as parameters the amount of cash at the beginning and end of the
day, and the name of the file, checks whether the actual amount of cash at the end of the day equals the
expected value, and returns the result as a string. Use this template: BalanceTransactions.java
You must catch any FileNotFoundException that is thrown and print out a message in the format:
"File: [filename] not found", e.g., File: transactions1.txt not found
Example:
balance (0, 55.55, "res/transactions1.txt , "); // returns "End of day total
is properly balanced."
balance (25, 55.55, "res/transactions1.txt ¿ "); // returns "End of day
total is not balanced."
balance (0, 55.55, "res/doesnotexist.txt"); // prints out "File:
res/doesnotexist.txt not found"
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
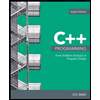
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
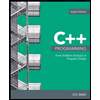
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning