The program will READ in data from a text file named StudyHours.txt. The user corrects any bad data. The program updates the information in StudyHours.txt file. For example if the file contains a letter grade of K which is not a possible letter grade. I'm not sure if I can use another method and call it in the hours method or if I need to add the code directly to the Hour method I've already done. An example of the StudyHours.txt file is: Aaron RODgers 12 A Tom brady 9 K philip Rivers apple c Joe Theismann 15 B
In Java - I'm having a hard time with this part of my project. Under menu option A and B I need to do the following:
- The program will READ in data from a text file named StudyHours.txt. The user corrects any bad data. The program updates the information in StudyHours.txt file. For example if the file contains a letter grade of K which is not a possible letter grade.
I'm not sure if I can use another method and call it in the hours method or if I need to add the code directly to the Hour method I've already done.
An example of the StudyHours.txt file is:
Aaron RODgers
12
A
Tom brady
9
K
philip Rivers
apple
c
Joe Theismann
15
B
So basically I need a user to be able to read the file, and correct the information they want, such as looking for apple and changing it to 9.
import java.util.Scanner;
import javax.swing.JOptionPane;
import java.io.*;
import java.util.ArrayList;
public class studyTime {
public static void main(String[] args) {
JOptionPane.showMessageDialog(null, "Welcome to the Study Time Calculator by Bob Smith.\nThis program calculates the number of hours needed\n"
+ "to study to get the letter grade desired.\nThe user can also see the averages and total of all users. ");
showMenu ();
}//end of main
public static void showMenu () {
String input;
char option;
input = JOptionPane.showInputDialog("A: How many hours do you need to study?\nB: What grade will you earn if you study this many hours?\n"
+ "C: Total number of students and average hours: \n D: Exit Program");
option = input.charAt(0);
if(option=='A' || option == 'a') {
Hours();
}
else if (option =='B' || option== 'b') {
Grades();
}
else if (option == 'C' || option== 'c') {
Display();
}
else if (option == 'D' || option == 'd') {
Goodbye();
}
else JOptionPane.showMessageDialog(null, "Invalid menu option.");
}
public static String ProperCase (String input) {
StringBuilder titleCase = new StringBuilder(input.length());
boolean nextTitleCase = true;
for (char c : input.toCharArray()) {
if (Character.isSpaceChar(c)) {
nextTitleCase = true;
} else if (nextTitleCase) {
c = Character.toTitleCase(c);
nextTitleCase = false;
}
titleCase.append(c);
}
return titleCase.toString();
}// end of proper case
public static void Hours() {
int hourstostudy=0,credits=0;
String name, input;
char grade, option;
name = JOptionPane.showInputDialog("What is your name? Please enter your first and last name such as Casie West.");
input = JOptionPane.showInputDialog("How many credits are you taking this semester? Credits should be no more than 18 and divisible by three.");
credits = Integer.parseInt(input);
input = JOptionPane.showInputDialog("What grade would you like to earn?");
grade = input.charAt(0);
if(grade == 'A' || grade == 'a')
hourstostudy=15;
else if(grade == 'B' || grade == 'b')
hourstostudy = 12;
else if(grade == 'C' || grade == 'c')
hourstostudy = 9;
else if(grade == 'D' || grade == 'd')
hourstostudy = 6;
else if(grade == 'F' || grade == 'f')
hourstostudy = 0;
else
JOptionPane.showMessageDialog(null, "Invalid Grade");
hourstostudy *=(credits/3);
name = ProperCase(name);
try {
FileWriter fwriter = new FileWriter("StudentsHoursGrades.txt", true);
PrintWriter outputFile = new PrintWriter(fwriter);
outputFile.println(name);
outputFile.println(credits);
outputFile.println(hourstostudy);
outputFile.println(grade);
outputFile.close();
}
catch (Exception e) {
System.out.println(e);
}
showMenu();
System.out.println("\tStudent Name: "+name);
System.out.println("\tCredits: "+credits);
System.out.println("\tTotal number of weekly study hours: "+hourstostudy);
System.out.println("\tDersired Grade: "+grade);
showMenu();
}// end of Hours method
public static void Grades() {
int hourstostudy=0,credits=0;
String name, input;
char grade, option;
name = JOptionPane.showInputDialog("What is your name?");
input = JOptionPane.showInputDialog("How many credits are you taking this semester?");
credits = Integer.parseInt(input);
input = JOptionPane.showInputDialog("How many hours are you willing to study?");
hourstostudy = Integer.parseInt(input);
if(hourstostudy*(credits/3) > 24*7)
System.out.println("Invalid Hours to Study Value.");
if(hourstostudy >=15)
grade = 'A';
else if(hourstostudy >=12 && hourstostudy <15)
grade = 'B';
else if(hourstostudy >=9 && hourstostudy <12)
grade = 'B';
else if(hourstostudy >=6 && hourstostudy <9)
grade ='D';
else
grade ='F';
hourstostudy *=(credits/3);
name = ProperCase(name);
try {
FileWriter fwriter = new FileWriter("StudentsHoursGrades.txt", true);
PrintWriter outputFile = new PrintWriter(fwriter);
outputFile.println(name);
outputFile.println(credits);
outputFile.println(hourstostudy);
outputFile.println(grade);
outputFile.close();
}
catch (Exception e) {
System.out.println(e);
}
showMenu();
System.out.println("\tStudent Name: "+name);
System.out.println("\tCredits: "+credits);
System.out.println("\tTotal number of weekly study hours: "+hourstostudy);
System.out.println("\tDersired Grade: "+grade);
}//end of grades method

Actually, given information:
- The program will READ in data from a text file named StudyHours.txt. The user corrects any bad data. The program updates the information in StudyHours.txt file. For example if the file contains a letter grade of K which is not a possible letter grade.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

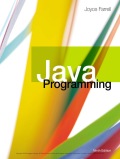
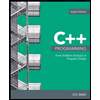
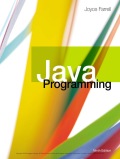
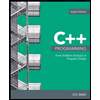