Add another public method dblValue() to your Fraction class which returns the double precision approximation value of the fraction. That is, the floating point result of actually dividing numerator by denominator. N.B. this method does not do any display itself, but can be called by a client program to be used in an output statement. Eg: if a client has a fraction frac that represents 1 / 2, then a method call to frac.dblValue() should return the double number 0.5. Use your client program to test this functionality; i.e. provide an output statement to display the double value of a fraction
Add another public method dblValue() to your Fraction class which returns the
double precision approximation value of the fraction. That is, the floating
point result of actually dividing numerator by denominator. N.B. this method
does not do any display itself, but can be called by a client program to be used
in an output statement. Eg: if a client has a fraction frac that represents 1 / 2,
then a method call to frac.dblValue() should return the double number 0.5.
Use your client program to test this functionality; i.e. provide an output
statement to display the double value of a fraction
here are my codes
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package lab4;
/**
*
* @author engko
*/
public class Fraction {
//Define the class Fraction
// declaring instance variables
String res;
private int num, denom;
// default constructor to initialize instance variables
public Fraction() {
this.num = 0;
this.denom = 1; // Initialize the values
}
// parameterized constructor to initialize instance variables
public Fraction(int n, int d) {
this.num = n; // Initialize the variables
this.denom = d;
}
// Getter method to get the numerator
public int getNum() {
// Returns numerator
return num;
}
// Getter method to get the denominator
public int getDen() {
// Returns denominatorinator
return denom;
}
// Method to check if the Fraction is zeroFraction or not
public boolean isZero() {
return (getNum() == 0 && getDen() != 0);
}
// Method to Check if two fractions are equal or not
public boolean isEquals(Object obj) {
Fraction q = (Fraction) obj;
if (num == num && denom == q.denom)
return true;
return false;
}
// method to add two fractions
public Fraction add(Fraction q) {
int numerator = num * q.getDen() + denom * q.getNum();
int denominator = denom * q.getDen();
return new Fraction(numerator, denominator);
}
// get the GCD of two numbers
public int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
// simplify the Fraction
void simplify() {
// get gcd of num and denom
int gcd = gcd(num, denom);
// check if gcd more than 1
if (gcd > 1) {
num /= gcd;
denom /= gcd;
}
}
// String representation of Fraction
public String toString() {
return num + "/" + denom;
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package lab4;
import java.util.Scanner;
/**
*
* @author engko
*/
public class TestFraction {
// Create the object for scanner class
static Scanner sc = new Scanner(System.in);
// Define the main method
public static void main(String[] args) {
// use the do-while loop to iterate test the conditions
do {
// Create the object of Fraction class
Fraction fraction = takeFractionValues();
// check if first Fraction is zeroFraction or not
if (!fraction.isZero()) {
// second fraction details
System.out.println("\nEnter Second Fraction Details: ");
Fraction fraction1 = takeFractionValues();
System.out.println(fraction + " AND " + fraction1);
// check if two fractions are equal or not
if (fraction1.isEquals(fraction)) {
System.out.println("\nTrue-> Both Fractions are Equal");
} else
System.out.println("\nFalse-> Both Fractions are not Equal");
// add two fractions fraction and fraction1
Fraction result = fraction.add(fraction1);
result.simplify();
System.out.println("Result of Both Fraction Addition: " + result);
} else // fraction1 is zerofraction so end the loop
break;
System.out.println("\nEnter First fraction as Zero Fraction to end the Loop");
} while (true);
//print on newline
System.out.println("\n\nFinished Working with Fractions...");
}
//take Fraction value as input from the user
public static Fraction takeFractionValues() {
int num, denom;
// Prompts the user to enter the numerator
System.out.print("Enter the num: ");
// Store the Integer part only
num = Integer.parseInt(sc.nextLine().trim());
// Prompts the user to enter the denominator
// validating Deonominator value
while (true) {
// to give modified message on invalid value
String append = "";
System.out.print("Enter the den "+append+": ");
// store the integer part only
denom = Integer.parseInt(sc.nextLine().trim());
// check if denom is 0
if (denom == 0) {
System.out.println("Denominator can't be zero");
append = "again";
} else
break;
}
//return the New Fraction
return new Fraction(num, denom);
}
}

Step by step
Solved in 4 steps with 2 images

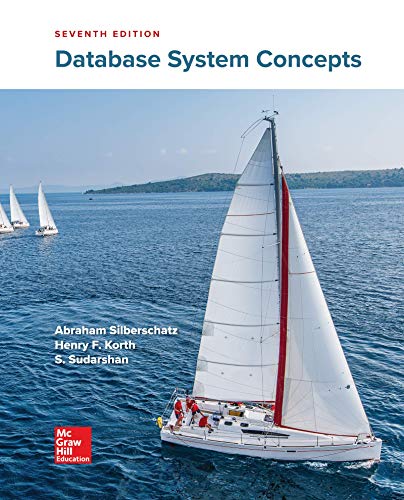
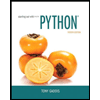
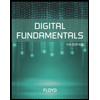
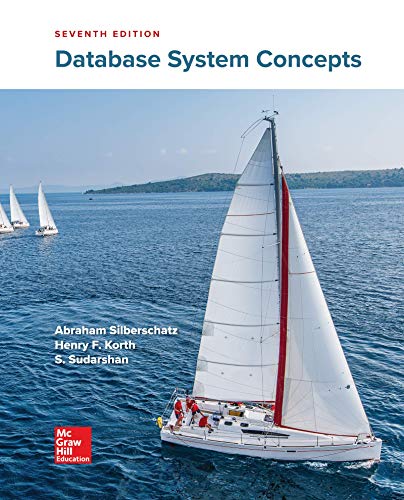
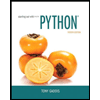
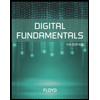
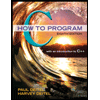
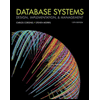
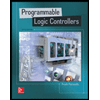