Alter the attached code so that the bar chart can be up to 25 categories, and can be sorted ascending or descending. In this assignment an array of Category structs are used to store both the category name (label) and the category value. The Category struct is provided below along with changes to the prototypes from assignment 3. The get_longest_category_name function has been removed because after sorting, the last or first element in the cats array will be the longest length label. Use this information in create_bar_chart and the asc Boolean value where true is sort ascending and false is sort descending.
Alter the attached code so that the bar chart can be up to 25 categories, and can be sorted ascending or descending. In this assignment an array of Category structs are used to store both the category name (label) and the category value. The Category struct is provided below along with changes to the prototypes from assignment 3. The get_longest_category_name function has been removed because after sorting, the last or first element in the cats array will be the longest length label. Use this information in create_bar_chart and the asc Boolean value where true is sort ascending and false is sort descending.
struct Category
{
std::string label;
double value;
};
- Update the attached code to include the following in global scope:
#define CATEGORIES 25
int num_categories = 5;
- Change the attached code's functions to the following:
//Ask user how many categories, up to a max of 25
int how_many_categories();
void get_category(Category cats[CATEGORIES]);
void get_values(Category cats[CATEGORIES]);
double compute_total(Category cats[CATEGORIES]);
//
// sort the cats array of Category structs
// do not use selection sort or STL's sort
// use: bubblesort, insertion-sort, etc.
void sort(Category cats[CATEGORIES], bool asc=true);
// can get the longest_category_length from the sorted cats array
void create_bar_chart(Category categories[CATEGORIES], double total, bool asc=true);
- Sample main for new code
int main()
{
double total{ 0.0 };
bool asc = true;
while (true) {
std::cout << "Enter 1 or 0 for ascending (1) or descending order (0)?";
std::cin >> asc;
while (std::cin.fail())
{
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'\n');
std::cout << "Enter 1 or 0 for ascending (1) or descending order (0)?";
std::cin >> asc;
}
num_categories = how_many_categories();
get_category(cats);
get_values(cats);
total = compute_total(cats);
//sort categories, if ascending last element for longest, first for descending
sort(cats, num_categories, asc);
std::cout << "\nCategories as a Percentage of the Total (category_amount/" << total << ")\n";
std::cout << "~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~\n";
create_bar_chart(cats, total, asc);
std::cout << "\nEnter y to create another bar chart, or any other key to exit : ";
std::string response; std::cin >> response; std::cout << "\n";
if (response != "y")
break;
total = 0.0;
}
}
![for (int i = 0; i<5; i++) {
}
}
if (cat[i].length() > longest) {
longest = cat[i].length();
}
void create_bar_chart(std::string cat [CATEGORIES], double values [CATEGORIES], int
lcl, double total) {
for (int i = 0; i<5; i++) {
std::cout << cat[i] << "\t\t";
lcl = (values[i]/total)*100;
for(int j = 0; j<lcl; j++) {
std::cout << "*";
}
std::cout << lcl << "\n";](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e826cb-c133-47e8-aeaa-858e7b3a09da%2Fa9a40315-cbf0-428b-ac5c-08fac65adb50%2F7u9ce8r_processed.png&w=3840&q=75)
![#include <iostream>
#include <string>
#define CATEGORIES 5
void get_category(std::string cat [CATEGORIES]);
void get values (double values [CATEGORIES], std::string cat [CATEGORIES]);
void compute_total(double& total, double values [CATEGORIES]);
void get_longest_category_name
(int& longest, std::string cat [CATEGORIES]);
void create_bar_chart(std::string cat [CATEGORIES], double values [CATEGORIES], int
lcl, double total);
int main() {
std::string categories [CATEGORIES];
double values [CATEGORIES]; double total; int lcl;
while(true) {
get_longest_category_name(lcl, categories);
std::cout << "\nCategories as a percentage of the total
(category_amount/" << total <<")\n";
std::cout <<
to exit: ";
}
void
}
}
void
get_category(categories);
get_values (values, categories);
compute_total(total, values);
}
create_bar_chart (categories, values, lcl, total);
std::cout << "\nEnter y to create another bar chart, or any other key
char response;
std::cin>> response;
std::cout << "\n";
if (response != 'y')
break;
lcl = 0; total = 0.0;
get_category(std::string cat [CATEGORIES]) {
std::cout<<"Enter in the category names for your five-bar bar chart: \n";
for (int i = 0; i<5; i++) {
std::cin>> cat[i];
}
void get_values (double values [CATEGORIES], std::string cat [CATEGORIES]) {
for (int i = 0; i<5; i++) {
std::cout << "How many in the " << cat[i] << "category? ";
std::cin>> values[i];
-\n";
void compute_total (double& total, double values [CATEGORIES]) {
total = 0;
for (int i = 0; i<5; i++) {
total values[1];
}
get_longest_category_name (int& longest, std::string cat [CATEGORIES]) {
longest = 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e826cb-c133-47e8-aeaa-858e7b3a09da%2Fa9a40315-cbf0-428b-ac5c-08fac65adb50%2Fs8pdxy5_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

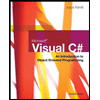
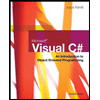