Assignment:Write a C++ program that computes a student’s grade for an assignment as a percentage given the student’s score and total points. The final score must be rounded up to the nearest whole value using the ceil function in the header file. You must also display the floating-point result up to 5 decimal places. The input to the program must come from a file containing a single line with the score and total separated by a space. In addition, you must print to the console “Excellent” if the grade is greater than or equal to 90, “Well Done” if the grade is less than 90 and greater than or equal to 80, “Good” if the grade is less than 80 and greater than or equal to 70, “Need Improvement” if the grade is less than 70 and greater than or equal to 60, and “Fail” if the grade is less than 60.
Question: I screenshot my work, but do not if i put extra in that does not need to be there or if i am missing something to not be able to get rid of the errors listed at the bottom. theyre both in relation to line 1 and i dont even see a probelm with the line.
Assignment:Write a C++ program that computes a student’s grade for an assignment as a percentage given the student’s score and total points. The final score must be rounded up to the nearest whole value using the ceil function in the header file. You must also display the floating-point result up to 5 decimal places. The input to the program must come from a file containing a single line with the score and total separated by a space. In addition, you must print to the console “Excellent” if the grade is greater than or equal to 90, “Well Done” if the grade is less than 90 and greater than or equal to 80, “Good” if the grade is less than 80 and greater than or equal to 70, “Need Improvement” if the grade is less than 70 and greater than or equal to 60, and “Fail” if the grade is less than 60.
![30
total[totalValue]
value;
=
31
//increment the counter
32
totalValue++;
33
34
35
36
for (int i = 0; i < totalValue; i++)
37
38
//calculate percentage
39
grade = score[i]/total[i];
//display percentage in floating-point with the five decimal places
cout <« fixed <« ceil(grade * 100) « "%" << fixed << grade;
40
41
//when percentage is greater than or equal to 90
if (ceil(grade * 100) >= 90)
{
//display grade
42
43
44
45
46
cout << "Excellent" << endl;
}
//when percentage is less than 90 and greater than or equal to 80
else if (cei1(grade * 100) >= 80)
{
//display grade
cout <« "Well-Done" << endl;
47
48
49
50
51
52
}
//when percentage is less than 80 and greater than or equal to 70
else if (ceil(grade * 100) >= 70)
{
//display grade
53
54
55
56
57
58
cout <« "Good" << endl;
}
//when percentage is less than 70 and greater than or equal to 60
else if (cei1(grade * 100) >= 60)
{
//display grade
cout <« "Need Improvement" << endl;
}
//when the percentage is less than 60
59
60
61
62
63
64
65
66
67
else
{
//display grade
cout << "Fail" << endl;
68
69
70
71
}
72
73
//Program logic
//Closing program statements
system("pause");
74
75
76
return 0;
77
78
//Function definitions
0-](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdc07602e-78f4-4480-b951-83c1bebc7673%2F760d046f-01be-4442-aaf2-5b784981e281%2Fvq90dt_processed.png&w=3840&q=75)
![D//<Headache Creator>
-- if/else assignment
//CSIS 111-<D01> ADD YOUR SECTION NUMBER
//<Sources if necessary>
| //Include statements
E#include <iostream>
#include <string>
#include <cmath>
8
#include <fstream>
using namespace std;
B//Global declarations: Constants and type definitions only -- no variables
| //Function prototypes
Bint main()
{
//In cout statement below SUBSTITUTE your name and lab number
10
11
12
13
14
15
cout << "Julianna Brown
Lab Number 3" <« endl « endl;
16
//Variable declarations
double score[20], total[20], value, grade;
int totalValue = 0;
17
18
//create an object of the input stream
ifstream fromFile;
19
20
//opening the file
fromFile.open("input.txt");
21
22
//get student score from the file
fromFile >> value;
23
24
25
//add student score in the array
score[totalValue] = value;
//get the total from the file
fromFile >> value;
26
27
28
//add total in the array
total[totalValue] = value;
29
30
31
//increment the counter
32
totalValue++;
33
34
35
36
for (int i = 0; i < totalValue; i++)
1 2 3 4 5 6 7co 0으-의의의밀 음답](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdc07602e-78f4-4480-b951-83c1bebc7673%2F760d046f-01be-4442-aaf2-5b784981e281%2F6edfuff_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

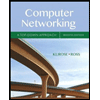
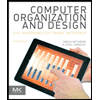
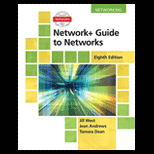
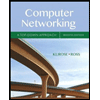
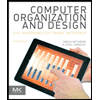
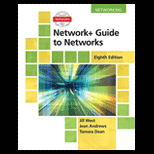
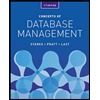
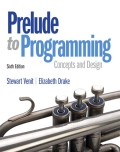
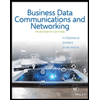