By the end of this lab, you will have acquired a better understanding of SMTP protocol. You will also gain experience in implementing a standard protocol using Python. Your task is to develop a simple mail client that sends email to any recipient. Your client will need to connect to a mail server, dialogue with the mail server using the SMTP protocol, and send an email message to the mail server. Python provides a module, called smtplib, which has built-in methods to send mail using SMTP protocol. However, you will not be using this module in this lab, because it hides the details of SMTP and socket programming. In order to limit spam, some mail servers do not accept TCP connections from arbitrary sources. For the experiment described below, you may want to try connecting both to your university mail server and to a popular Webmail server, such as an AOL mail server. To connect to the university mail server, you will need to use NYU’s VPN. You may also try making your connection both from your home and from your university campus. from socket import * def smtp_client(port=1025, mailserver='127.0.0.1'): msg = "\r\n My message" endmsg = "\r\n.\r\n"
By the end of this lab, you will have acquired a better understanding of SMTP protocol. You will also gain experience in implementing a standard protocol using Python.
Your task is to develop a simple mail client that sends email to any recipient. Your client will need to connect to a mail server, dialogue with the mail server using the SMTP protocol, and send an email message to the mail server. Python provides a module, called smtplib, which has built-in methods to send mail using SMTP protocol. However, you will not be using this module in this lab, because it hides the details of SMTP and socket programming.
In order to limit spam, some mail servers do not accept TCP connections from arbitrary sources. For the experiment described below, you may want to try connecting both to your university mail server and to a popular Webmail server, such as an AOL mail server. To connect to the university mail server, you will need to use NYU’s VPN. You may also try making your connection both from your home and from your university campus.
from socket import *
def smtp_client(port=1025, mailserver='127.0.0.1'):
msg = "\r\n My message"
endmsg = "\r\n.\r\n"
# Choose a mail server (e.g. Google mail server) if you want to verify the script beyond GradeScope
# Create socket called clientSocket and establish a TCP connection with mailserver and port
clientSocket = socket(AF_INET, SOCK_STREAM)
clientSocket.connect((mailserver, port))
# Fill in start
# Fill in end
recv = clientSocket.recv(1024).decode()
print(recv)
if recv[:3] != '220':
print('220 reply not received from server.')
# Send HELO command and print server response.
heloCommand = 'HELO Alice\r\n'
clientSocket.send(heloCommand.encode())
recv1 = clientSocket.recv(1024).decode()
# print(recv1)
if recv1[:3] != '250':
print('250 reply not received from server.')
# Send MAIL FROM command and print server response.
# Fill in start
mailFrom = 'Mail from<xugeegeegee@gmail.com>\r\n'
clientSocket.send(mailFrom.encode())
recv1 = clientSocket.recv(1024).decode()
# print(recv1)
if recv1[:3] != '250':
print('250 reply not received from server.')
# Fill in end
# Send RCPT TO command and print server response.
# Fill in start
rcptTo = 'RCPT TO: <smtp.nyu.edu> \r\n'
clientSocket.send(rcptTo.encode())
recv1 = clientSocket.recv(1024)
# print("After RCPT TO command: " + recv3)
if recv1[:3] != '250':
print('250 reply not received from server.')
# Fill in end
# Send DATA command and print server response.
# Fill in start
data = 'DATA\r\n'
clientSocket.send(data.encode())
recv1 = clientSocket.recv(1024)
# print("After DATA command: " + recv4)
if recv1[:3] != '250':
print('250 reply not received from server.')
# Fill in end
# Send message data.
# Fill in start
clientSocket.send('\r\n')
clientSocket.send(msg)
clientSocket.send(endmsg)
# Fill in end
# Message ends with a single period.
# Fill in start
clientSocket.send('.\r\n')
recv1 = clientSocket.recv(1024)
print(recv1)
if recv1[:3] != '250':
print('250 reply not received from server.')
# Fill in end
# Send QUIT command and get server response.
# Fill in start
quit = 'QUIT\r\n'
clientSocket.send(quit.encode())
recv1 = clientSocket.recv(1024)
# print(recv1)
if recv1[:3] != '250':
print('250 reply not received from server.')
clientSocket.close()
# Fill in end
if __name__ == '__main__':
smtp_client(1025, '127.0.0.1')
This is my code, can you help me find where did I do wrong?
![from socket import *
def smtp client (port=1025, mailserver='127.0.0.1'):
msg = "\r\n My message"
endmsg
"\r\n.\r\n"
# Choose a mail server (e.g. Google mail server)
if you want to verify the script beyond GradeScope
# Create socket called clientSocket and establish a TCP connection with mailserver and port
# Fill in start
# Fill in end
recv = clientSocket.recv(1024).decode ()
print (recv)
if recv[:3]
print ('220 reply not received from server.')
!= '220':
# Send HELO command and print server response.
heloCommand
'HELO Alice\r\n'
clientSocket.send (heloCommand.encode () )
recv1 = clientSocket.recv(1024).decode ()
print (recv1)
if recvl[:3] != '250':
print ('250 reply not received from server.')
# Send MAIL FROM command and print server response.
# Fill in start
# Fill in end
# Send RCPT TO command and print server response.
# Fill in start
# Fill in end
# Send DATA command and print server response.
# Fill in start
# Fill in end
# Send message data.
# Fill in start
# Fill in end
# Message ends with a single period.
# Fill in start
# Fill in end
# Send QUIT command and get server response.
# Fill in start
# Fill in end
if
name
main
smtp_client (1025, '127.0.0.1')](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd484a4b5-f85c-4659-9baa-8e6f09e92697%2F81a326f8-6e46-4030-ae3b-154726f71a60%2Fdquud7w_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

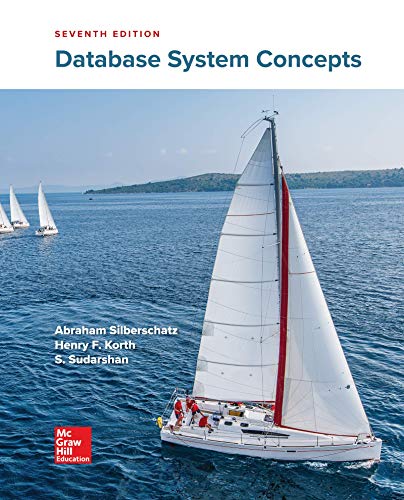
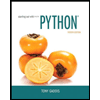
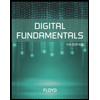
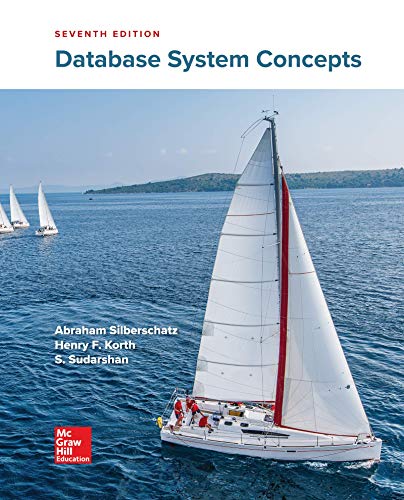
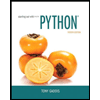
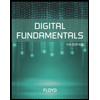
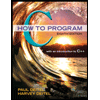
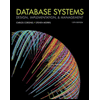
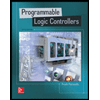