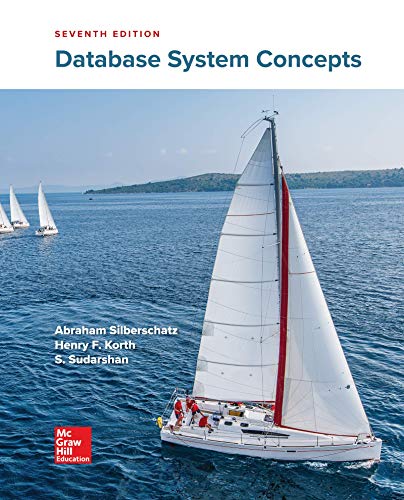
Concept explainers
Task 4 – Socket
Complete Assignment 3: Mail Client of the Socket Programming Assignments found on Page 180 of the prescribed text book. Name your file mailstudentnumber.py
The goal of this programming assignment is to create a simple mail client that sends email to any recipient. Your client will need to establish a TCP connection with a mail server (e.g., a Google mail server), dialogue with the mail server using the SMTP protocol, send an email message to a recipient (e.g., your friend) via the mail server, and finally close the TCP connection with the mail server.
Your job is to complete the code and test your client by sending email to different user accounts. You may also try sending through different servers (for example, through a Google mail server and through your university mailserver).
- Run this server and client on the mininet topology created in Task
- Run wireshark to capture all network traffic, when executing the server and client
- Capture wireshark screenshot and upload to

Step by stepSolved in 3 steps

- Modify the server code so that it prints the client’s IP and port number when it receive a new connection. from socket import * serverName = 'localhost' serverPort = 12000 clientSocket = socket(AF_INET, SOCK_STREAM) clientSocket.connect((serverName,serverPort)) sentence = input('Input lowercase sentence:') byteToSend = str.encode (sentence) clientSocket.send(byteToSend) modifiedSentence = clientSocket.recv(1024) print('From Server:', modifiedSentence) clientSocket.close() from socket import * serverPort = 12000 serverSocket = socket(AF_INET,SOCK_STREAM) serverSocket.bind(('',serverPort)) serverSocket.listen(1) print ('The server is ready to receive') while 1: connectionSocket, addr = serverSocket.accept() sentence = connectionSocket.recv(1024) capitalizedSentence = sentence.upper() connectionSocket.send(capitalizedSentence) connectionSocket.close() from socket import * serverName = 'localhost' serverPort = 12000 clientSocket = socket(AF_INET,…arrow_forwardCan anyone help me with basic client-server interaction using TCP sockets in network programming in c code that includes csapp.c and csapp.h. and a text file of the student record that include first name, last name, age, major, and graducation year. The ideas is have 4 choice: Add record, Search Age Range, Search graducation year, and Terminate. In opition 1, it should collect all the data to the text file, in opition 2, it should search for age range from the text file. in opition 3, it should search the graducation year from the text file and if the year is not there it should print record not found. And opition 4, the client should close the connection and terminate. studentRecord.txt: John,Doe,24,Computer Science,2023Jane,Doe,22,Mechanical Engineering,2020Charles,Babbage,21,Math,2022George,Bool,25,Math,2023Marie,Curie,26,Chemistry,2023 I know this isn't enough but I want to understand about the client-server interaction using socket interface. So please try to make it simple…arrow_forwardA browser sends an HTTP request to a web server on port 80. One line of the request is "GET / HTTP/1.1". Explain the purpose of the request,and describe the process that will be used to prepare the response. It is not necessary to show actual content of the HTTP response.arrow_forward
- I made a TCP server using socket python,basically my server can: - Upload (“put”) request: The client should, at the very least, open (in binary mode) the local file defined on the command line, read its data, send it to the server through the socket, and finally close the connection. - Download (“get”) request: The client should, at the very least, create the local file defined on the command line (in exclusive binary mode), read the data sent by the server, store it in the file, and finally close the connection. To avoid accidents, the client should deny overwriting existing files. - Listing (“list”) request: the client should, at the very least, send an appropriate request message, receive the listing from the server, print it on the screen one file per line, and finally close the connection. I want you to draw an ER diagram that can show the process above please.arrow_forwardwrite a custom Snort rule to handle Inbound and Outbound HTTP traffic on the Private (Host-Only) network. Upload a screen shot of the Snort console displaying the alerts. In this exercise, we are going to create two Snort monitoring rules that will be used to alert on HTTPnetwork traffic for both Inbound and Outbound traffic. Remember, Inbound rules are those rules whosedestination is to your internal network (HOME_NET), outbound rules are directed out of your internalnetwork (!HOME_NET). When you use “any” there is no distinction on whether a rule is Inbound orOutbound. When using Inbound/Outbound to describe local traffic, traffic generated on the samenetwork (as in this lab on VMnet-1), the Inbound reference is to your client system that is running snortthe Outbound reference is to the HTTP server.You should now understand a little bit about custom rules, so given the following rule:alert tcp any any -> any 80 (msg:"TCP HTTP Testing Rule"; sid:1000004;)You should be able to…arrow_forwardQuestion 23 A client's browser sends an HTTP request to a website. The website responds with a handshake and sets up a TCP connection. The connection setup takes 2.1 ms, including the RTT. The browser then sends the request for the website's index file. The index file references 8 additional images, which are to be requested/downloaded by the client's browser. Assuming all other conditions are equal, how much longer would non-persistent HTTP take than persistent HTTP? (Give answer in milliseconds, without units, rounded to one decimal place. For an answer of 0.01005 seconds, you would enter "10.1" without the quotes.)arrow_forward
- C Programming: Write a program that optionally accepts an address and a port from the command line. If there is no address and port on the command line, it should create a TCP socket and print the address (i.e. server mode). You can choose any port number for your server (>1024). If there is an address and port, it should connect to it (i.e., client mode). Once the connections are set up, each side should enter a loop of receive, print what it received, then send a message. The ping from the client should be sent before entering that loop to start the process. Otherwise both sides will sit and listen without getting anything. The message should be ping from the client and a pong from the server. In order to test this on one machine, you will need to run the same program twice (in two separate terminals). Run first in server mode, then run in client mode using the information printed from the server as your command line arguments. This is the output it must print out: Running…arrow_forwardimport socket def authenticate_user(tcp_socket): while True: response = tcp_socket.recv(1024).decode() print(response) username = input() tcp_socket.sendall(username.encode()) response = tcp_socket.recv(1024).decode() print(response) password = input() tcp_socket.sendall(password.encode()) auth_response = tcp_socket.recv(1024).decode() if "successful" in auth_response: print(auth_response) return True else: print(auth_response) def receive_items(tcp_socket): while True: response = tcp_socket.recv(1024).decode() if "Item List:" in response: print(response) while True: item = tcp_socket.recv(1024).decode() if not item.strip(): break print(item) break def select_items(tcp_socket): selected_items = [] while True: item_id = input("Select item by entering…arrow_forwardSuppose an HTTP client makes a first GET request to the gaia.cs.umass.edu web server for a base page that it has never before requested, which contains an embedded object, which causes the client to make a second GET request. A very short time later, the client then makes a third GET request - for the same base page, with that third GET request having an If-Modified-Since field (as does the 4th GET request that the client makes for the embedded object). Neither the base object nor the jpeg object has changed. How many round trip times (RTTs) are needed from when the client first makes the third GET request (i.e., when it requests the base object for the second time) to when the base page and the jpeg file are displayed a second time, assuming that: any time needed by the server to transmit the base file, or the jpeg file into the server's link is (each) equal to 1/2 RTT the time needed to transmit an HTTP GET into the client's link is zero? the time needed by the server to transmit…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
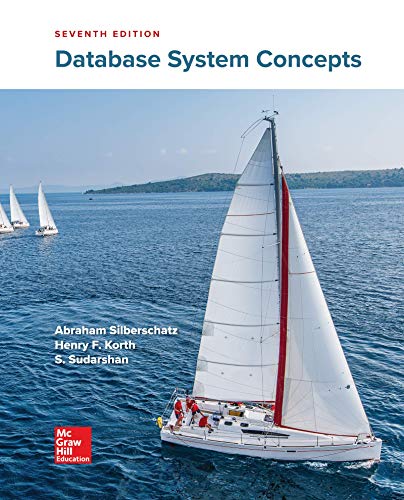
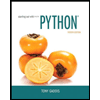
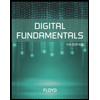
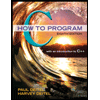
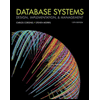
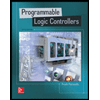