C++ Assignment---This is an almost finished code, please complete it. Make changes if necessary. Objectives • To apply knowledge of classes and objects Instructions Implement all of the following, each in a separate Visual Studio project (and/or solution). 1. Define a class called CounterType to implement a simple counter. The class must have a private field (data member) counter of type int. You must also provide methods (member functions), including the following: a. A constructor to set the counter to the value specified by the user b. A constructor to set the counter to 0 c. A method to allow the user to set the counter to a value they specify d. A method to increment the counter by 1 e. A method to decrement the counter by 1 Your code must ensure the value of counter is never negative. If the user calls a method to attempt to make the counter negative, set the counter to 0 and print out an error to the user (e.g., “The counter must not be negative.”) Do not take any user input from inside this class. You should provide a separate main.cpp file, housing main, in which you may test the CounterType class. You don’t need to ask for user input. Just hardcode values for a couple different instances of CounterType to test it out. 2. Create a program to allow the user to enter a value as a Roman numeral, and store the value as a Roman numeral (string) in a class called RomanNumeralType. You may do this through a constructor requiring a Roman numeral to be entered a. Provide a method to return the value as the original roman numeral b. Provide a method to return the value as a positive integer In your main file, in the main function, test out the following values: MCXIV, CCCLIX, and MDCLXVI You must allow for the following symbols: M 1000 D 500 C 100 L 50 X 10 V 5 I 1 Notes and Help: • Our own number system (called the Hindu-Arabic number system), is a positional number system (1s place, 10s place, 100s place, etc.) – the number positions always have meaning regardless of what the other numbers are that surround them • Roman numerals are an additive number system o This means that the positions of the values depend on the values around them • You must allow for value input of 1 to 4999 in Roman numerals o That is, from I to MMMMCMXCIX Counting in Roman numerals would go something like this: I, II, III, IV, V, VI, VII, VIII, IX, X (1 – 10) XI, XII, XIII, XIV, XV, XVI, XVII, XVIII, XIX, XX (11 – 20) XXI, XXII, XXIII, XXIV, XXV, XXVI, XXVII, XXVIII, XXIX, XXX (21 – 30) Pay close attention to the value 4, and the value 9 (In Roman numerals, instead of IIII, it’s “1 before 5”, which is IV, and instead of VIIII it’s IX, which is “1 before 10”) Other useful values that might help you: C = 100, CC = 200, CCC = 300, CD = 400, D = 400, DC = 600, DCC = 700, DCCC = 800, CM = 900, M = 1000 Note the 400 is “100 before 500, which is 400”, and 900 is represented as “100 before 1000, which is 900”. M = 1000, MM = 2000, MMM = 3000, MMMM = 4000 MMMMC = 4100, MMMMCC = 4200, MMMMCCC = 4300, MMMMCD = 4400, MMMMD = 4500, MMMMDC = 4600, MMMMDCC = 4700, MMMMDCCC = 4800, MMMMCM = 4900 Note the 4900 is “4 thousands, and one hundred before the next thousand, which is 900, so it’s 4900”. Deliverables Please create a folder named proj2-cis2252. Place copies of each of the separate Visual Studio solutions in this top level folder. Alternatively, you may copy the source files (e.g., .cpp and .h) in the appropriate folder corresponding to the problem number. (E.g., proj2-1, proj2-2) and then place those folders inside the proj2- cis2252 folder. Then, zip the folder and then upload that zipped file to D2L under the appropriate Assignment directory. #include #include #include using namespace std; class counterType { int counter; public: counterType(int count) { counter = count; } counterType() { counter=0; } void setCounter(int c) { counter = c; } int getCounter() { return counter; } int increment() { return ++counter; } int decrement() { if(counter < 0) { cout<0) : "<>c; obj1.setCounter(c); if(c<0) { counterType obj; cout<<"Value of counter is negative "<
C++ Assignment---This is an almost finished code, please complete it. Make changes if necessary.
Objectives
• To apply knowledge of classes and objects
Instructions
Implement all of the following, each in a separate Visual Studio project (and/or solution).
1. Define a class called CounterType to implement a simple counter. The class must have a private field
(data member) counter of type int. You must also provide methods (member functions), including the
following:
a. A constructor to set the counter to the value specified by the user
b. A constructor to set the counter to 0
c. A method to allow the user to set the counter to a value they specify
d. A method to increment the counter by 1
e. A method to decrement the counter by 1
Your code must ensure the value of counter is never negative. If the user calls a method to
attempt to make the counter negative, set the counter to 0 and print out an error to the user
(e.g., “The counter must not be negative.”)
Do not take any user input from inside this class. You should provide a separate main.cpp file,
housing main, in which you may test the CounterType class. You don’t need to ask for user input.
Just hardcode values for a couple different instances of CounterType to test it out.
2. Create a program to allow the user to enter a value as a Roman numeral, and store the value as a
Roman numeral (string) in a class called RomanNumeralType. You may do this through a constructor
requiring a Roman numeral to be entered
a. Provide a method to return the value as the original roman numeral
b. Provide a method to return the value as a positive integer
In your main file, in the main function, test out the following values: MCXIV, CCCLIX, and MDCLXVI
You must allow for the following symbols:
M 1000
D 500
C 100
L 50
X 10
V 5
I 1
Notes and Help:
• Our own number system (called the Hindu-Arabic number system), is a positional number system (1s
place, 10s place, 100s place, etc.) – the number positions always have meaning regardless of what the
other numbers are that surround them
• Roman numerals are an additive number system
o This means that the positions of the values depend on the values around them
• You must allow for value input of 1 to 4999 in Roman numerals
o That is, from I to MMMMCMXCIX
Counting in Roman numerals would go something like this:
I, II, III, IV, V, VI, VII, VIII, IX, X (1 – 10)
XI, XII, XIII, XIV, XV, XVI, XVII, XVIII, XIX, XX (11 – 20)
XXI, XXII, XXIII, XXIV, XXV, XXVI, XXVII, XXVIII, XXIX, XXX (21 – 30)
Pay close attention to the value 4, and the value 9 (In Roman numerals, instead of IIII, it’s “1 before 5”, which is
IV, and instead of VIIII it’s IX, which is “1 before 10”)
Other useful values that might help you:
C = 100, CC = 200, CCC = 300, CD = 400, D = 400, DC = 600, DCC = 700, DCCC = 800, CM = 900, M = 1000
Note the 400 is “100 before 500, which is 400”, and 900 is represented as “100 before 1000, which is 900”.
M = 1000, MM = 2000, MMM = 3000, MMMM = 4000
MMMMC = 4100, MMMMCC = 4200, MMMMCCC = 4300, MMMMCD = 4400, MMMMD = 4500,
MMMMDC = 4600, MMMMDCC = 4700, MMMMDCCC = 4800, MMMMCM = 4900
Note the 4900 is “4 thousands, and one hundred before the next thousand, which is 900, so it’s 4900”.
Deliverables
Please create a folder named proj2-cis2252. Place copies of each of the separate Visual Studio solutions in this
top level folder. Alternatively, you may copy the source files (e.g., .cpp and .h) in the appropriate folder
corresponding to the problem number. (E.g., proj2-1, proj2-2) and then place those folders inside the proj2-
cis2252 folder. Then, zip the folder and then upload that zipped file to D2L under the appropriate Assignment
directory.
#include<iostream>
#include <stdio.h>
#include <string>
using namespace std;
class counterType
{
int counter;
public:
counterType(int count)
{
counter = count;
}
counterType()
{
counter=0;
}
void setCounter(int c)
{
counter = c;
}
int getCounter()
{
return counter;
}
int increment()
{
return ++counter;
}
int decrement()
{
if(counter < 0)
{
cout<<endl;
cout<<"Value of counter is negative "<<endl;
return counter;
}
return --counter;
}
void print()
{
cout<<"Final value of Counter : "<<counter<<endl;
}
};
int main()
{
counterType obj1(10);
int c;
cout<<"Enter the value of counter (>0) : "<<endl;
cin>>c;
obj1.setCounter(c);
if(c<0)
{
counterType obj;
cout<<"Value of counter is negative "<<endl;
cout<<" Value of counter initially : "<<obj.getCounter()<<endl;
cout<<"Value of counter after Incrementing : "<<obj.increment()<<endl;
cout<<"Value of counter after Decrementing : "<<obj.decrement()<<endl;
}
else
{
cout<<"Value of counter initially : "<<obj1.getCounter()<<endl;
cout<<"Value of counter after Incrementing : "<<obj1.increment()<<endl;
cout<<"Value of counter after Decrementing : "<<obj1.decrement()<<endl;
cout<<endl; obj1.print();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

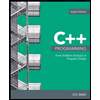
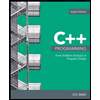