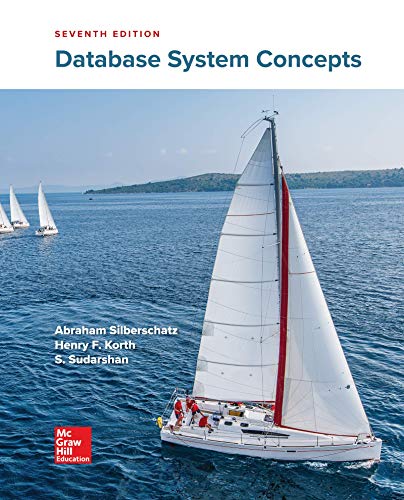
Concept explainers
C++
How would you write a function that takes a filename and an array of struct time, opens a file, reads each line in the file as the number of days, converts this to a struct time and stores this is in an array?
Here is my code so far (p.s. the function i need help with is called void readData):
#include<iostream>
#include<string>
#include<fstream>
#include<cstdlib>
#include<
using namespace std;
struct time{
// private:
int years, months, days;
//public:
time(){}
time(int days){
}
};
time getYearsMonthsDays(int days){
time x;
x.years = days/450;
days = days%450;
x.months = days/30;
x.days = days%30;
return x;
}
int getTotalDays(time x){
int totalDays;
totalDays = x.years*450 + x.months*30 + x.days;
return totalDays;
}
void openofile(string ofilename,ofstream &fout){
fout.open(ofilename.c_str());
if(!fout){
cout << "Error opening output file..." << endl;
exit(1);
}
}
void openifile(string ifilename,ifstream &fin){
fin.open(ifilename.c_str());
if(!fin){
cout << "Error opening input file..." << endl;
exit(1);
}
}
void readData(vector <time> &myTimeVector, ifstream &fin){
string ifilename; // takes a file name
int days;
time xArray[days]; // takes an array of struct time
fin.open(ifilename.c_str()); // opens the file
while (!fin.eof()){
fin >> days; // reads each line as the number of days
time x = time(days); // converts this to a struct time
myTimeVector.push_back(x); // and stores this in the array
}
fin.close();
}
bool compare(time a, time b){
if (a.days < b.days)
return true;
else
return false;
}
void swap(time &a, time &b){
time temp;
temp = a;
a = b;
b = temp;
}
void sort(vector <time> &myTimeVector){
for (int i = 0; i < myTimeVector.size(); i++)
for (int j = i+1; j < myTimeVector.size(); j++)
if (compare(myTimeVector[i], myTimeVector[j]))
swap (myTimeVector[i], myTimeVector[j]);
}
void printData(vector <time> myTimeVector, ostream &out){
time x;
for (int i = 0; i < myTimeVector.size(); i++){
out << x.years << "\t" << x.months << "\t" << x.days << endl;
}
}
int main(){
time x;
string ifilename1 = "/home/ec2-user/environment/timedata.txt";
string ofilename1 = "/home/ec2-user/environment/timeoutput.txt";
vector<time> myTimeVector;
ifstream fin;
ofstream fout;
openifile(ifilename1,fin);
openofile(ofilename1,fout);
readData(myTimeVector,fin);
fin.close();
printData(myTimeVector,cout);
printData(myTimeVector,fout);
cout << "========================"<<endl;
fout << "========================"<<endl;
sort(myTimeVector);
printData(myTimeVector,cout);
printData(myTimeVector,fout);
fout.close();
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Write a program in C++ that allows the user to create files of random data. They should be offered a menu of possibilities: 1) create random Whole number data file 2) create random Decimal number data file 3) create random Character data file 4) Quit programarrow_forwardWhich of the following are true? O The C-library has many functions that are useful in manipulating strings. V If a and b are two different C-string variables, the value in a can be assigned the value in b with the following C-statement: a = b; %3D O The length of the char array holding the string must be at least one character longer than the string itself. O An array identifier cannot be used as a pointer. V The identifier, name, of a C-string is also a pointer to a char data type. The ending character in a C-string must be the newline character, '\n'. O The name of a 1-D array is a pointer to the data type of the array, while the name of a 2-D array is a pointer to a 1-D array of the data type.arrow_forwardPlease complete the following functions: 1. int loadDictionary(istream& dictfile, vector<string>& dict) 2. int permute(string word, vector<string>& dict, vector<string>& results) 3. void display(vector<string>& results)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
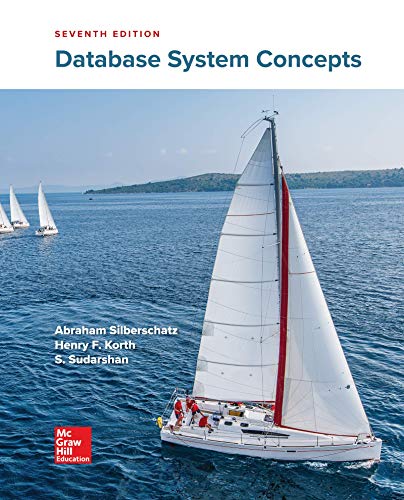
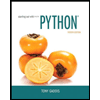
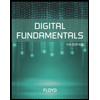
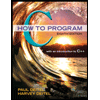
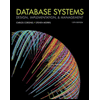
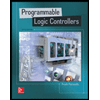