c++ The Lock class of the preceding problem has a small problem. It does not support combinations that start with one or more zeroes. For example, a combination of 0042 can be opened by entering just 42. Solve this problem by choosing a different representation. Instead of an integer, use a string to hold the input. Once again, the combination is hardwired. You will see in Section 9.6 how to change it. Complete the following file:
c++ The Lock class of the preceding problem has a small problem. It does not support combinations that start with one or more zeroes. For example, a combination of 0042 can be opened by entering just 42. Solve this problem by choosing a different representation. Instead of an integer, use a string to hold the input. Once again, the combination is hardwired. You will see in Section 9.6 how to change it. Complete the following file:
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section12.3: Class Scope And Duration Categories
Problem 3E
Related questions
Question
c++
The Lock class of the preceding problem has a small problem. It does not support combinations that start with one or more zeroes. For example, a combination of 0042 can be opened by entering just 42.
Solve this problem by choosing a different representation. Instead of an integer, use a string to hold the input.
Once again, the combination is hardwired. You will see in Section 9.6 how to change it.
Complete the following file:
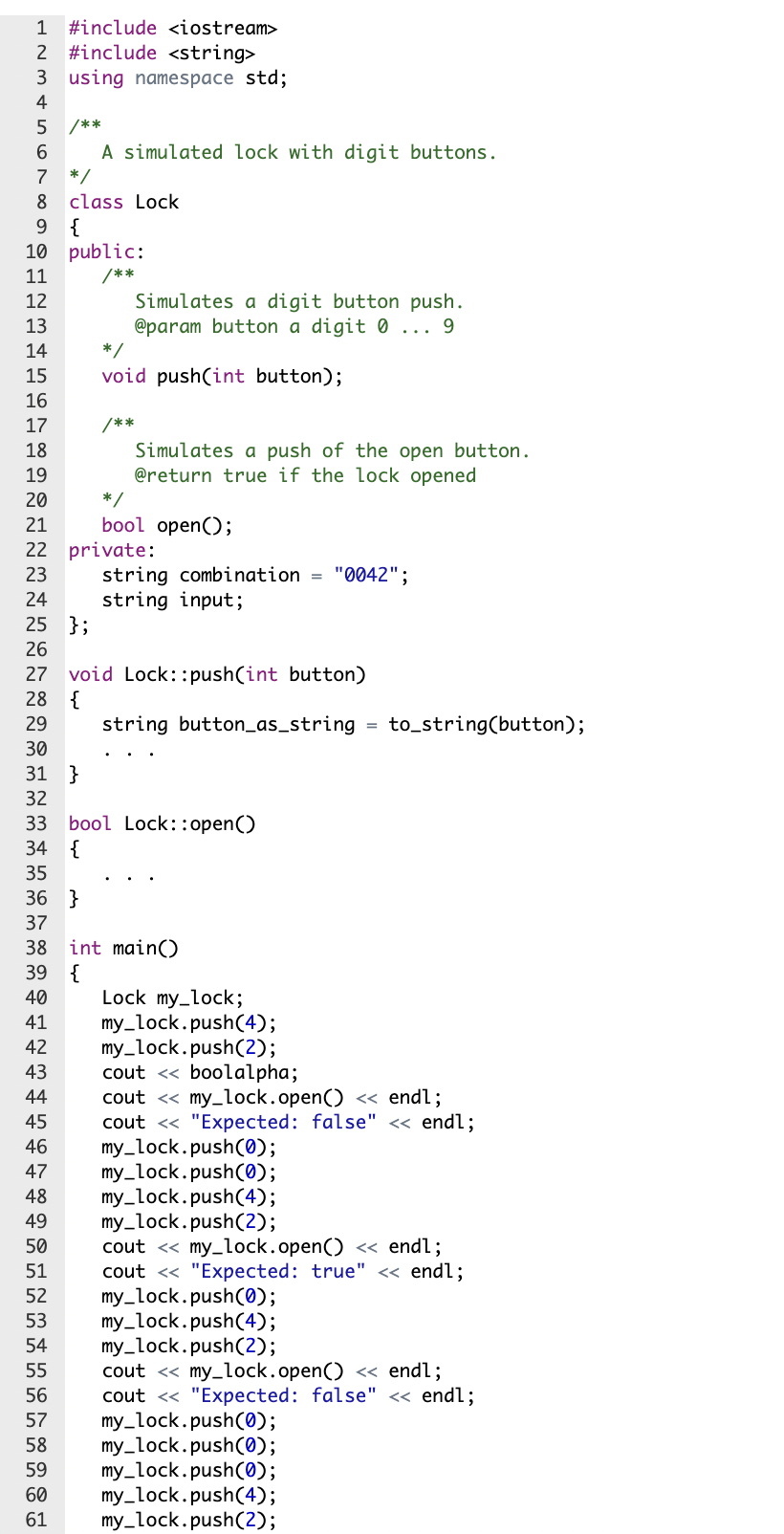
Transcribed Image Text:1
#include <iostream>
2 #include <string>
3 using namespace std;
4
5 /**
6.
A simulated lock with digit buttons.
7
*/
8.
class Lock
9 {
10 public:
11
/**
Simulates a digit button push.
@param button a digit 0
12
13
9.
14
*/
15
void push(int button);
16
17
/**
Simulates a push of the open button.
@return true if the lock opened
18
19
20
*/
21
bool open();
22 private:
"0042";
string combination =
string input;
23
24
25 };
26
void Lock::push(int button)
28 {
27
29
string button_as_string
to_string(button);
%3D
30
31 }
32
33 bool Lock::open()
{
34
35
36 }
37
38
int main()
39 {
40
Lock my_lock;
my_lock.push(4);
my_lock.push(2);
cout <« boolalpha;
cout <« my_lock.open() << endl;
cout <« "Expected: false" « endl;
my_lock.push(0);
my_lock.push(0);
my_lock.push(4);
my_lock.push(2);
cout <« my_lock.open() << endl;
cout <« "Expected: true" « endl;
my_lock.push(0);
my_lock.push(4);
my_lock.push(2);
cout <« my_lock.open() << endl;
cout <« "Expected: false" « endl;
my_lock.push(0);
my_lock.push(0);
my_lock.push(0);
my_lock.push(4);
my_lock.push(2);
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
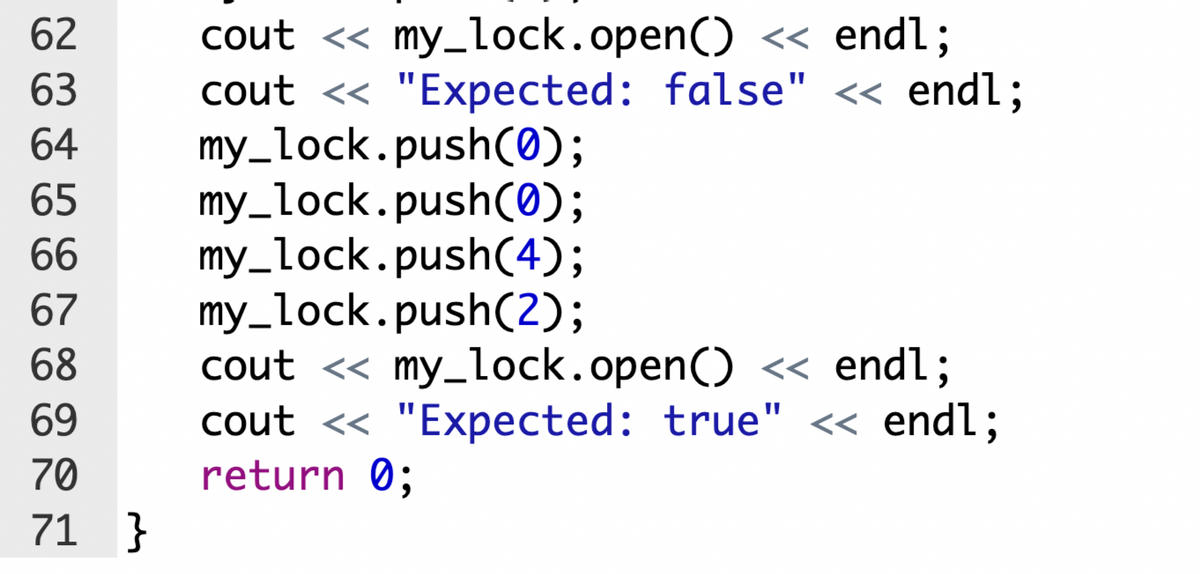
Transcribed Image Text:cout « my_lock.open() « endl;
cout <« "Expected: false" « endl;
my_lock.push(0);
my_lock.push(0);
my_lock.push(4);
my_lock.push(2);
cout <« my_lock.open() << endl;
cout <« "Expected: true" <« endl;
return 0;
62
63
64
65
66
67
68
69
70
71 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
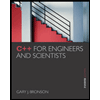
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
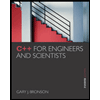
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr