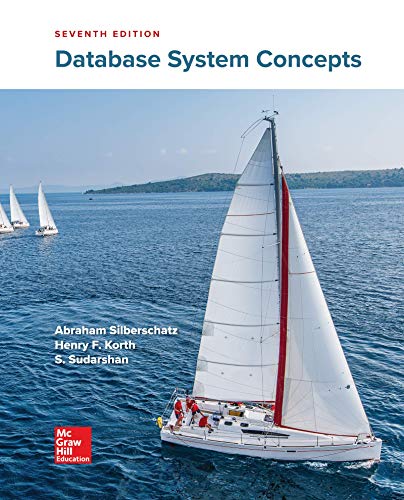
Concept explainers
C++ Need help with part 8
This is what i have so far
#include <iostream>
#include <string>
using namespace std;
int GetNumOfNonWSCharacters(const string text);
int GetNumOfWords(const string text);
int FindText(string text, string sample_text);
string ReplaceExclamation(string text);
string ShortenSpace(string text);
char PrintMenu(string sample_text){
char option;
cout << "\nMENU"<<endl;
cout << "c - Number of non-whitespace characters"<<endl;
cout << "w - Number of words"<<endl;
cout << "f - Find text"<<endl;
cout << "r - Replace all !'s"<<endl;
cout << "s - Shorten spaces"<<endl;
cout << "q - Quit"<<endl;
cout<<endl;
cout << "Choose an option:"<<endl;
cin >> option;
if(option == 'c'){
int nonWSCharacters = GetNumOfNonWSCharacters(sample_text);
cout << "Number of non-whitespace characters: "<<nonWSCharacters<<endl;
}else if(option == 'w'){
int numOfWords = GetNumOfWords(sample_text);
cout << "Number of words: "<<numOfWords<<endl;
}else if(option == 'f'){
cout<<"Enter a word or phrase to be found:"<<endl;
string phrase;
cin.ignore();
getline(cin, phrase, '\n');
int instances = FindText(phrase,sample_text);
cout << "\""<< phrase << "\" instances: "<<instances<<endl;
}else if(option == 'r'){
string edited_text = ReplaceExclamation(sample_text);
cout << "Edited text: "<<edited_text<<endl;
}else if(option == 's'){
string edited_text = ShortenSpace(sample_text);
cout << "Edited text: "<<edited_text;
}
return option;
}
int GetNumOfNonWSCharacters(const string text){
int len = text.length(), nonWSCharacters = 0;
for(int i = 0; i < len; i++){
if(text[i] != ' ' && text[i] != '\n' && text[i] != '\t')
nonWSCharacters++;
}
return nonWSCharacters;
}
int GetNumOfWords(const string text){
int len = text.length(), numOfWords = 0;
for(int i = 0; i < len; i++){
if(text[i] == ' ' || text[i] == '\n' || text[i] == '\t'){
numOfWords++;
++i;
while(i < len && (text[i] == ' ' || text[i] == '\n' || text[i] == '\t'))
++i;
--i;
}
}
if(text[len-1] != ' ' || text[len-1] != '\n' || text[len-1] != '\t')
numOfWords++;
return numOfWords;
}
int FindText(string text, string sample_text){
int sample_length = sample_text.length();
int text_length = text.length();
int instances = 0;
for(int i=0;i<=sample_length-text_length; i++){
int j = 0;
for(; j<text_length; j++){
if(text[j]!=sample_text[i+j])
break;
}
if(j==text_length){
instances++;
}
}
return instances;
}
string ReplaceExclamation(string sample_text){
int len = sample_text.length();
int i = 0;
string edited_text = "";
while(i < len)
{
if(sample_text[i] == '!'){
edited_text += '.';
}else{
edited_text += sample_text[i];
}
i++;
}
return edited_text;
} string ShortenSpace(string sample_text){
int len = sample_text.length();
int i = 0;
string edited_text = "";
while(i < len-1)
{
if(sample_text[i] == ' ' && sample_text[i+1] == ' ')
{
i++;
continue;
}else{
edited_text += sample_text[i];
}
i++;
}
if(sample_text[len-1] != ' '){
edited_text += sample_text[len-1];
}
return edited_text;
}
int main(){
string sample_text;
cout << "Enter a sample text:"<<endl;
getline(cin, sample_text);
cout<<endl;
cout << "You entered: ";
cout<<sample_text<<endl;
while(true){
char option = PrintMenu(sample_text);
if(option=='q')
break;
}
return 0;
}
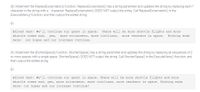

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- C++ cout << "Homework 5 Part 2"; cout << "Enter the name of the user "; getline(cin, name); string name, phoneNumber, address, city; outfile.open("list.txt"); outFile << "Name: " << name << endl; outfile.close();arrow_forwardc++problemarrow_forward#include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forward
- #include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forwardIn C++ Assume that a 5 element array of type string named boroughs has been declared and initialized. Write the code necessary to switch (exchange) the values of the first and last elements of the array.arrow_forwardIN C++ Write code that: creates 3 integer values - one can be set to 0, the other two should NOT multiples of one another (for example 3 and 6, or 2 and 8) take you largest value and perform a modulus operation using the smaller (non zero) value as the divisor print out the result. create an alias for the string type call the alias "name" then create an instance of the "name" class and assign it a value using an appropriate cout statement - print out the value Please screenshot your input and output, as the format tends to get messed up. Thank you!arrow_forward
- python programing: You are going to create a race class, and inside that class it has a list of entrants to the race. You are going to create the daily schedule of three or so races and populate the races with the jockey/horse entries and then print out the schedule. Create a race class that has The name of the race as a character string, ie “RACE 1” or “RACE 2”, The time of the race A list of entries for that race Create a list of of 3 (or 4) of those race objects(races) for the day For the list of entries for a particular race, make a entrant class (the building block of your entrants list) you should have The horse name The jockey name Now you need to Populate the structure (ie set up three races on the schedule, add 2-3 horses entries to each race. You can hardcode the entries if you want to, rather than getting them from the user, just to save the time of making a menu interface and whatnot. Be able to print the days schedule So example output would be…arrow_forwardPlease code this in C++. Do not use namespace std. Please use basic code. Thank you for your time. Common Elements Write a program that prompts the user to enter two arrays of 10 integers and displays the common elements that appear in both arrays. Here is a sample run. Enter list1: 8 5 10 1 6 16 61 9 11 2 Enter list2: 4 2 3 10 3 34 35 67 3 1 The common elements are 10 1 2arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
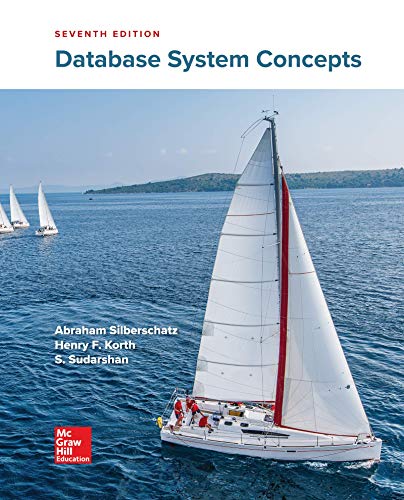
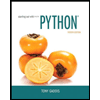
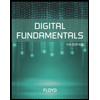
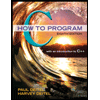
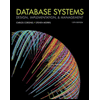
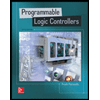