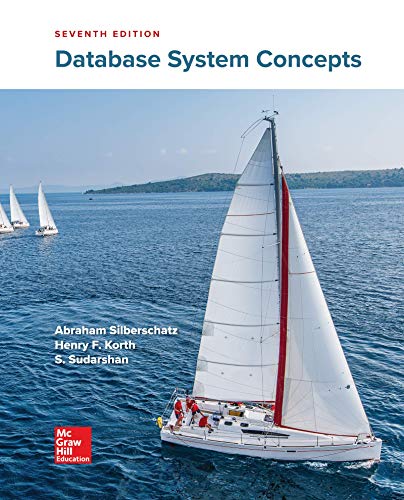
Concept explainers
#include <iostream> #include <string> using namespace std;
//define class & its public/private members
class bankAccount {
public:
setAccountNumber(int); setAccountBalance(double); putMoneyIn(double); takeMoneyOut(double);
const void
const void
const void
const void
void printAccountInfo();
private:
int accountNumber;
double accountBalance; int main()
//run all the functionas
bankAccount account1; account1.setAccountNumber(123456); account1.setAccountBalance(10000); account1.printAccountInfo(); account1.takeMoneyOut(200);
cout << "Your information after withdrawling $200 is as follows"; cout << endl;
account1.printAccountInfo();
account1.putMoneyIn(1293);
cout << "Your information after depositing $1293 is as follows"; cout << endl;
account1.printAccountInfo();
system("pause");
return 0; }
//set the account #
const void bankAccount::setAccountNumber(int num)
}; {
{
};
accountNumber = num;
//set a starting balance
const void bankAccount::setAccountBalance(double bal) {
accountBalance = bal;
};
//deposit money
void const bankAccount::putMoneyIn(double amount) {
accountBalance += amount;
};
//withdraw money
const void bankAccount::takeMoneyOut(double amount) {
if (amount > accountBalance) {
std::endl; }
{
} }
accountBalance -= amount;
std::cout << "You have insufficient funds for this withdrawl." << else
//show account # & balance
void bankAccount::printAccountInfo() {
};
cout << "Your account number is #" << accountNumber << endl; cout << "Your account balance is $" << accountBalance << endl;
Modify the above code in C++ to add a savings and a checkings account. I need help defining each function and each class.
Every bank offers a checking account. Derive the class checkingAccount from the class bankAccount (designed above). This class inherits members to store the account number and the balance from the base class. A customer with a checking account typically receives interest, maintains a minimum balance, and pays service charges if the balance falls below the minimum balance. Add member variables to store this additional information. In addition to the operations inherited from the base class, this class should provide the following operations: set interest rate, retrieve interest rate, set minimum balance, retrieve minimum balance, set service charges, retrieve service charges, post interest, verify if the balance is less than the minimum balance, write a check, withdraw (override the method of the base class), and print account information. Add appropriate constructors.
Every bank offers a savings account. Derive the class savingsAccount from the class bankAccount (designed above). This class inherits members to store the account number and the balance from the base class. A customer with a savings account typically receives interest, makes deposits, and withdraws money. In addition to the operations inherited from the base class, this class should provide the following operations: set interest rate, retrieve interest rate, post interest, withdraw (override the method of the base class), and print account information. Add appropriate constructors.

Step by stepSolved in 4 steps with 4 images

How would I add the minimun balance functions and service charges? Also include write. check? How would I add deposit money? Can you add it to your code so I can see where everything is supposed to go?
How would I add the minimun balance functions and service charges? Also include write. check? How would I add deposit money? Can you add it to your code so I can see where everything is supposed to go?
- class Airplane – highlights -- planeID : String -planeModel: String //”Boeing 757” etc. --seatCapacity : int //would vary by outfitting ………… Assume getters and setters, toString(), constructor with parameters and constructor ……………… Write lambdas using a standard functional interface to: a) Given a plane object and a required number of seats as an input, determine if the given plane meets or exceeds the required capacity b) Output the plane description if capacity is less than 80% of a specified number (We would use his for planning aircraft assigned to various routes. ) c) Adjust the seats in a plane by a specified positive or negative number [after modifications have been made to the interior.] …and write an example for each showing the usage, using an Airplane instance plane1arrow_forwardC# List the differences between CommissionEmployee class and BasePlusCommissionEmployee class public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; private decimal commissionRate; private decimal baseSalary; public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; CommissionRate = commissionRate; BaseSalary = baseSalary; } public decimal GrossSales { get { return grossSales; } set { if (value < 0) // validation { throw new ArgumentOutOfRangeException(nameof(value),…arrow_forwardProgram: File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File main.cpp: // include necessary header files #include <stdlib.h> #include "GamePurse.h" #include<iostream> #include<time.h> using namespace std; int main(){ // create the object of GamePurse class GamePurse dice(100); int amt=1; // seed the random generator srand(time(0)); // to play the…arrow_forward
- public class Book { protected String title; protected String author; protected String publisher; protected String publicationDate; public void setTitle(String userTitle) { title = userTitle; } public String getTitle() { return title; } public void setAuthor(String userAuthor) { author = userAuthor; } public String getAuthor(){ return author; } public void setPublisher(String userPublisher) { publisher = userPublisher; } public String getPublisher() { return publisher; } public void setPublicationDate(String userPublicationDate) { publicationDate = userPublicationDate; } public String getPublicationDate() { return publicationDate; } public void printInfo() { System.out.println("Book Information: "); System.out.println(" Book Title: " + title); System.out.println(" Author: " + author); System.out.println(" Publisher: " + publisher); System.out.println(" Publication…arrow_forwardC# Solve this error using System; namespace RahmanA3P1BasePlusCEmployee { public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; // gross weekly sales private decimal commissionRate; // commission percentage private decimal baseSalary; // base salary per week // six-parameter constructor public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { // implicit call to object constructor occurs here FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; // validates gross sales CommissionRate = commissionRate; // validates commission rate BaseSalary = baseSalary; // validates base…arrow_forwardC:/Users/r1821655/CLionProjects/untitled/sequence.cpp:48:5: error: return type specification for constructor invalidtemplate <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;};} 48 | void sequence<Item>::sequence() : used(0), current_index(0) { } | ^~~~ line 47 template<class Item> line 48 void sequence<Item>::sequence() : used(0), current_index(0) { }arrow_forward
- class implementation file -- Rectangle.cpp class Rectangle { #include #include "Rectangle.h" using namespace std; private: double width; double length; public: void setWidth (double); void setLength (double) ; double getWidth() const; double getLength() const; double getArea () const; } ; // set the width of the rectangle void Rectangle::setWidth (double w) { width = w; } // set the length of the rectangle void Rectangle::setLength (double l) { length l; //get the width of the rectangle double Rectangle::getWidth() const { return width; // more member functions herearrow_forward/* (name header)*/public class BatteryTester{ public static void main(String[] args) { //=========================battery1============================ //Create a Battery object called battery1 using the default constructor System.out.println("=====Battery1====="); //Print the max capacity and the current capacity of battery1 //Check if the battery is full and print the result. You need to call the method isFull() //Drain the battery by 25.5 mAh //Print the current capacity of battery1 after draining //=========================battery2============================ //Create a Battery object called battery2 using the overloaded constructor and set the current capacity and //max capacity to 2000 mAh. System.out.println("\n=====Battery2====="); //Print the max capacity and the current capacity of battery2 //Drain the battery by 2200 mAh //Print…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
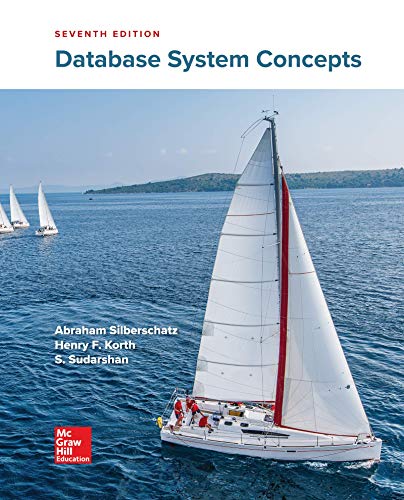
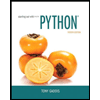
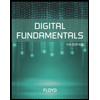
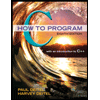
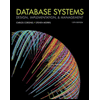
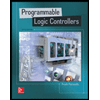