can I get help writing this in c++ It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date. The characteristics of stocks and bonds in a portfolio are shown below: Stocks: Bonds: Purchase date (Date) Purchase date (Date) Purchase price (double) Purchase price (double) Quantity purchased (int) Quantity purchased (int) Ticker symbol (string) Issuer (string) Par value (int) Face value (int) Stock type (i.e. Common or Preferred) (enum) Stated interest rate (double) Dividends per share (double) Maturity date (Date) Several of the data members above require the use of dates. Strings will not be acceptable substitutes for date fields. C++ does not have a built in data type for Date. Therefore, you may use this Date class in your program: #pragma once #include #include #include class Date{ friend std::ostream& operator<<(std::ostream&, Date); public: Date(int d=0, int m=0, int yyyy=0) { setDate(d, m, yyyy); } ~Date() {} void setDate(int d, int m, int yyyy){ day = d; month = m; year = yyyy; } private: int day; int month; int year; }; std::ostream& operator<<(std::ostream& output, Date d){ output << d.month << "/" << d.day << "/" << d.year; return output; } Security class (base class) The class should contain data members that are common to both Stocks and Bonds. Write appropriate member functions to store and retrieve information in the member variables above. Be sure to include a constructor and destructor. Stock class and Bond class. (derived classes) Each class should be derived from the Security class. Each should have the member variables shown above that are unique to each class. Each class should contain a function called calcIncome that calculates the amount a client receives as dividend or interest income for each security purchase. Note that the calcIncome algorithm has been simplified for this assignment. In real life, interest is paid on most bonds semi-annually, and dividends are declared by a company once per year. In our example, we are assuming that dividends are known at the time of the stock purchase and are not subject to change. We are also assuming an annual (as opposed to semi-annual) payment of interest on bonds. To calculate a stock puchase’s dividend income, use the following formula: income = dividends per share * number of shares. To calculate a bond purchase’s annual income, use this formula: income = number of bonds in purchase * the face value of the bonds * the stated interest rate. In each derived class, the << operator should be overloaded to output, neatly formatted, all of the information associated with a stock or bond, including the calculated income. The < operator should also be overloaded in each derived class to enable sorting of the vectors using the sort function. This function is part of the library. Write member functions to store and retrieve information in the appropriate member variables. Be sure to include a constructor and destructor in each derived class. Design a Portfolio class The portfolio has a name data member. The class contains a vector of Stock objects and a vector of Bond objects. There is no limit to the number of Stock and Bond puchases that can be added to a portfolio. The Portfolio class should support operations to purchase stocks for the portfolio, purchase bonds for the portfolio, or list all of the items in the portfolio (both stocks and bonds). Main() You should write a main() program that creates a portfolio and presents a menu to the user that looks like this: Pictures attached “S” should allow the user to record the purchase of some stocks and add the purchase to the Stocks list. Likewise, “B” should allow the user to record the purchase of some bonds and add the purchase to the Bonds list. “L” should list all of the securities in the portfolio, first displaying all of the Stocks, sorted by ticker symbol, followed by all of the bonds, sorted by issuer. The user should be able to add stocks, add bonds, and list repeatedly until he or she selects “Q” to quit.
can I get help writing this in c++
It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date.
The characteristics of stocks and bonds in a portfolio are shown below:
Stocks: Bonds:
Purchase date (Date) Purchase date (Date)
Purchase price (double) Purchase price (double)
Quantity purchased (int) Quantity purchased (int)
Ticker symbol (string) Issuer (string)
Par value (int) Face value (int)
Stock type (i.e. Common or Preferred) (enum) Stated interest rate (double)
Dividends per share (double) Maturity date (Date)
Several of the data members above require the use of dates. Strings will not be acceptable substitutes for date fields.
C++ does not have a built in data type for Date. Therefore, you may use this Date class in your program:
#pragma once
#include <iostream>
#include <cstdlib>
#include <cctype>
class Date{
friend std::ostream& operator<<(std::ostream&, Date);
public:
Date(int d=0, int m=0, int yyyy=0) {
setDate(d, m, yyyy);
}
~Date() {}
void setDate(int d, int m, int yyyy){
day = d;
month = m;
year = yyyy;
}
private:
int day;
int month;
int year;
};
std::ostream& operator<<(std::ostream& output, Date d){
output << d.month << "/" << d.day << "/" << d.year;
return output;
}
Security class (base class)
The class should contain data members that are common to both Stocks and Bonds.
Write appropriate member functions to store and retrieve information in the member variables above. Be sure to include a constructor and destructor.
Stock class and Bond class. (derived classes)
Each class should be derived from the Security class. Each should have the member variables shown above that are unique to each class.
Each class should contain a function called calcIncome that calculates the amount a client receives as dividend or interest income for each security purchase. Note that the calcIncome
To calculate a stock puchase’s dividend income, use the following formula: income = dividends per share * number of shares. To calculate a bond purchase’s annual income, use this formula: income = number of bonds in purchase * the face value of the bonds * the stated interest rate.
In each derived class, the << operator should be overloaded to output, neatly formatted, all of the information associated with a stock or bond, including the calculated income.
The < operator should also be overloaded in each derived class to enable sorting of the
Write member functions to store and retrieve information in the appropriate member variables. Be sure to include a constructor and destructor in each derived class.
Design a Portfolio class
The portfolio has a name data member.
The class contains a vector of Stock objects and a vector of Bond objects.
There is no limit to the number of Stock and Bond puchases that can be added to a portfolio.
The Portfolio class should support operations to purchase stocks for the portfolio, purchase bonds for the portfolio, or list all of the items in the portfolio (both stocks and bonds).
Main()
You should write a main() program that creates a portfolio and presents a menu to the user that looks like this: Pictures attached
“S” should allow the user to record the purchase of some stocks and add the purchase to the Stocks list. Likewise, “B” should allow the user to record the purchase of some bonds and add the purchase to the Bonds list. “L” should list all of the securities in the portfolio, first displaying all of the Stocks, sorted by ticker symbol, followed by all of the bonds, sorted by issuer. The user should be able to add stocks, add bonds, and list repeatedly until he or she selects “Q” to quit.
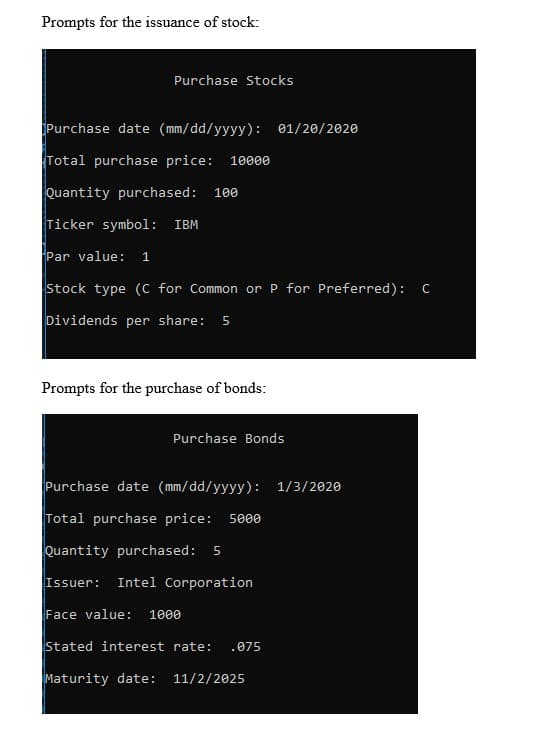
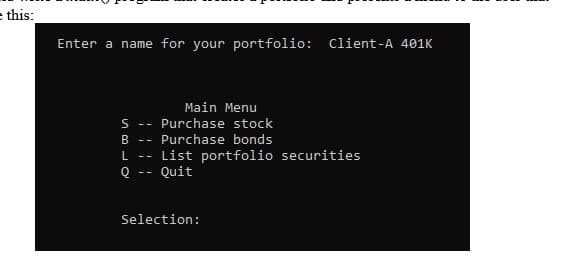

Trending now
This is a popular solution!
Step by step
Solved in 6 steps

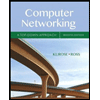
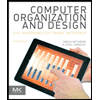
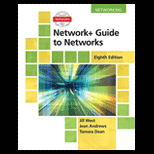
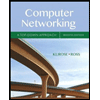
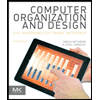
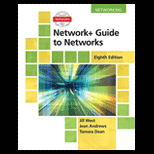
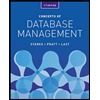
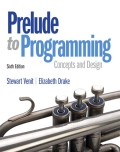
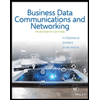