can someone help me with this in C++ You are going to be creating part of an event planning system. You will create three classes: Time, Date, Event. Together these classes will have the functionality to describe when events take place, and the ability to compare different times. Time This class is going to represent a 24-hour cycle clock time. This class has these data members. int sec int min int hour You will write the time class to have these functions. Default Constructor The default constructor will initialize the time object to its minimum value: 0:0:0. Constructor The time constructor will take in 3 integers, hours, minutes, seconds. If the constructor receives invalid numbers, like 63 minutes or -1 hours, then it will set that value to ZERO. The accepted ranges of the member variables: seconds: 0 -> 59 minutes: 0 -> 59 hours: 0 -> 23 Getters and Setters You should create a getter and setter method for each member variable. For this assignment we provide these. toString This function will return a string describing what time the object represents. The format is hour:minute:second. Time time = Time(17, 59, 1); cout << time.toString() << endl; The above code should return: 17:59:01 timeToSeconds int timeToSeconds() const; secondsToTime This is a Private helper function and will make the +, -, < ,and > functions easier. This function should take an integer as input and return a Time object. This function is provided in the template. const Time secondsToTime(int s) const; + and - operators The time class will support the addition and subtraction of two "Time" objects. The standard rollovers apply i.e. instead of having 63 seconds its 3 seconds into the next minute. If the resulting Time is past 23 hours then you roll hours to 0. const Time operator+(const &Time other) const; const Time operator-(const &Time other) const; > and < operators The Time class will support the greater than and less than operators. 0:0:0 is the smallest time and 23:59:59 is the greatest time. bool operator<(const& Time other) const; == operator Two time objects are equal when they have the same values for their member variables. bool operator==(const& Time other) const; Date This class will represent a calendar day. For simplicity, we are excluding leap years, and all months have 31 days. Thus, a year for this program has 372 days (31 days x 12). This class has these data members. int month int day int year You will write the date class to have these functions. Default Constructor The default constructor will initialize the date object to its minimum value: 1/1/0. Constructor The Date constructor will take 3 integer parameters, day, month, year. If the constructor receives invalid parameters, such as 15 for month, it will set that value to ZERO. The accepted ranges of the member variables: day: 1 -> 31 month: 1 -> 12 year: 0 -> inf Getters and Setters You should create a getter and setter method for each member variable. For this assignment we provide these. Note: The getter methods should be constant and their parameter should be a constant reference. toString This function will return a string describing what date the object represents. The format is dd/mm/yyyy. Date date = Date(5, 2, 1999); cout << date.toString() << endl; The above code should print: 5/2/1999 dateToDays This is a Private helper function and will make the +, -, < ,and > functions easier. This function takes no input and returns the number of days from the minimum Date. So a date 3/1/1 would return 1119. This function is Not provided in the template. int dateToDays() const; daysToDate This is a Private helper function and will make the +, -, < ,and > functions easier. This function takes aan integer as input and returns a Date. This is the reverse calculation of dateToDays. This function is Not provided in the template. const Date daysToDate(int ndays) const; + and - overload (Date + int) const Date operator+(int other) const; const Date operator-(int other) const; Date date1 = Date(5, 2, 2021); date1 = date1 + 7; cout << date1.toString() << endl; The above code should print: 12/2/2021 > and < overload The Date class will support the greater than and less than operators. 1/1/0 is the smallest date and 31/12/inf is the greatest date. bool operator<(const& Date other) const; bool operator>(const& Date other) const; == overload Two date objects are equal when they have the same values for their member variables. bool operator==(const& Date other) const; Event This class has these data members. string description string location Time time Date date You will write the event class to have these functions. Constructor The event constructor will take two strings, a time, and a date as input. The first is the description, the second is the location, third is time, fourth is date. hasPassed This function will return True if the event has passed. It will take a date and a time as input. Date today = Date(10, 20, 2021); Event event = Event("Party", "Carl's House",, Time(22, 0, 0), today); cout << event.hasPassed(today, Time(22, 10, 0)) << endl;
can someone help me with this in C++
You are going to be creating part of an event planning system. You will create three classes: Time, Date, Event. Together these classes will have the functionality to describe when events take place, and the ability to compare different times.
Time
This class is going to represent a 24-hour cycle clock time.
This class has these data members.
- int sec
- int min
- int hour
You will write the time class to have these functions.
Default Constructor
The default constructor will initialize the time object to its minimum value: 0:0:0.
Constructor
The time constructor will take in 3 integers, hours, minutes, seconds. If the constructor receives invalid numbers, like 63 minutes or -1 hours, then it will set that value to ZERO.
The accepted ranges of the member variables:
seconds: 0 -> 59 minutes: 0 -> 59 hours: 0 -> 23
Getters and Setters
You should create a getter and setter method for each member variable. For this assignment we provide these.
toString
This function will return a string describing what time the object represents. The format is hour:minute:second.
Time time = Time(17, 59, 1); cout << time.toString() << endl;
The above code should return:
17:59:01
timeToSeconds
int timeToSeconds() const;
secondsToTime
This is a Private helper function and will make the +, -, < ,and > functions easier. This function should take an integer as input and return a Time object. This function is provided in the template.
const Time secondsToTime(int s) const;
+ and - operators
The time class will support the addition and subtraction of two "Time" objects. The standard rollovers apply i.e. instead of having 63 seconds its 3 seconds into the next minute. If the resulting Time is past 23 hours then you roll hours to 0.
const Time operator+(const &Time other) const; const Time operator-(const &Time other) const;
> and < operators
The Time class will support the greater than and less than operators. 0:0:0 is the smallest time and 23:59:59 is the greatest time.
bool operator<(const& Time other) const;
== operator
Two time objects are equal when they have the same values for their member variables.
bool operator==(const& Time other) const;
Date
This class will represent a calendar day. For simplicity, we are excluding leap years, and all months have 31 days. Thus, a year for this program has 372 days (31 days x 12).
This class has these data members.
- int month
- int day
- int year
You will write the date class to have these functions.
Default Constructor
The default constructor will initialize the date object to its minimum value: 1/1/0.
Constructor
The Date constructor will take 3 integer parameters, day, month, year. If the constructor receives invalid parameters, such as 15 for month, it will set that value to ZERO.
The accepted ranges of the member variables:
day: 1 -> 31 month: 1 -> 12 year: 0 -> inf
Getters and Setters
You should create a getter and setter method for each member variable. For this assignment we provide these. Note: The getter methods should be constant and their parameter should be a constant reference.
toString
This function will return a string describing what date the object represents. The format is dd/mm/yyyy.
Date date = Date(5, 2, 1999); cout << date.toString() << endl;
The above code should print:
5/2/1999
dateToDays
This is a Private helper function and will make the +, -, < ,and > functions easier. This function takes no input and returns the number of days from the minimum Date. So a date 3/1/1 would return 1119. This function is Not provided in the template.
int dateToDays() const;
daysToDate
This is a Private helper function and will make the +, -, < ,and > functions easier. This function takes aan integer as input and returns a Date. This is the reverse calculation of dateToDays. This function is Not provided in the template.
const Date daysToDate(int ndays) const;
+ and - overload (Date + int)
const Date operator+(int other) const; const Date operator-(int other) const; Date date1 = Date(5, 2, 2021); date1 = date1 + 7; cout << date1.toString() << endl;
The above code should print:
12/2/2021
> and < overload
The Date class will support the greater than and less than operators. 1/1/0 is the smallest date and 31/12/inf is the greatest date.
bool operator<(const& Date other) const; bool operator>(const& Date other) const;
== overload
Two date objects are equal when they have the same values for their member variables.
bool operator==(const& Date other) const;
Event
This class has these data members.
- string description
- string location
- Time time
- Date date
You will write the event class to have these functions.
Constructor
The event constructor will take two strings, a time, and a date as input. The first is the description, the second is the location, third is time, fourth is date.
hasPassed
This function will return True if the event has passed. It will take a date and a time as input.
Date today = Date(10, 20, 2021); Event event = Event("Party", "Carl's House",, Time(22, 0, 0), today); cout << event.hasPassed(today, Time(22, 10, 0)) << endl;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

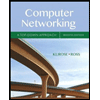
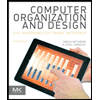
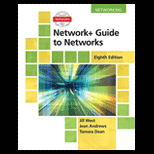
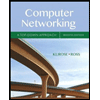
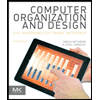
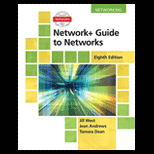
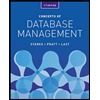
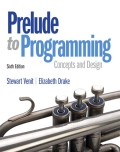
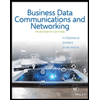