can you identify this Python Program below, where it is placed, and explain why and where it is used by following all the structures. please label where each structure is written...thank you 1.) Sequential Structures 2.) Decision Structures 3.) Repetition Structures 4.) String Methods 5.) Text File Manipulation 6.) Lists and Dictionaries 7.) Functions 8.) Program Modularization import numpy px_terms = int(input('Enter number of terms in px: ')) qx_terms = int(input('Enter number of terms in qx: ')) poly = {} for i in range(px_terms): ele = int(input('Enter coefficient {} for px: '.format(i + 1))) # if 'px' is not present already, add empty list with 'px' as key if 'px' not in poly: poly['px'] = [] # after adding empty list with 'px' as key, append input to the list # I added this line because it also add 1st coefficient to the list. poly['px'].append(ele) # else, append input to the list else: poly['px'].append(ele) for i in range(qx_terms): ele = int(input('enter coefficient {} for qx: '.format(i + 1))) # if 'qx' is not present already, add empty list with 'qx' as key if 'qx' not in poly: poly['qx'] = [] # after adding empty list with 'qx' as key, append input to the list. # I added this line because it also add 1st coefficient to the list. poly['qx'].append(ele) # else, append input to the list else: poly['qx'].append(ele) option = input('Select Addition, Subtraction, Multiplication, Division: ') if option.lower() == "addition": print(numpy.polynomial.polynomial.polyadd(poly['px'], poly['qx'])) if option.lower() == "subtraction": print(numpy.polynomial.polynomial.polysub(poly['px'], poly['qx'])) if option.lower() == "multiplication": total = 0 for i in range(px_terms): ans = poly['px'][i] * poly['qx'][i] print(f"answer{i+1} = ", ans) total += ans print(f"total = {total}") if option.lower() == "division": qu, re = numpy.polynomial.polynomial.polydiv(poly['px'], poly['qx']) print("Quotient: ", qu) print("Remainder: ", re)
can you identify this Python Program below, where it is placed, and explain why and where it is used
by following all the structures. please label where each structure is written...thank you
1.) Sequential Structures
2.) Decision Structures
3.) Repetition Structures
4.) String Methods
5.) Text File Manipulation
6.) Lists and Dictionaries
7.) Functions
8.) Program Modularization
import numpy
px_terms = int(input('Enter number of terms in px: '))
qx_terms = int(input('Enter number of terms in qx: '))
poly = {}
for i in range(px_terms):
ele = int(input('Enter coefficient {} for px: '.format(i + 1)))
# if 'px' is not present already, add empty list with 'px' as key
if 'px' not in poly:
poly['px'] = []
# after adding empty list with 'px' as key, append input to the list
# I added this line because it also add 1st coefficient to the list.
poly['px'].append(ele)
# else, append input to the list
else:
poly['px'].append(ele)
for i in range(qx_terms):
ele = int(input('enter coefficient {} for qx: '.format(i + 1)))
# if 'qx' is not present already, add empty list with 'qx' as key
if 'qx' not in poly:
poly['qx'] = []
# after adding empty list with 'qx' as key, append input to the list.
# I added this line because it also add 1st coefficient to the list.
poly['qx'].append(ele)
# else, append input to the list
else:
poly['qx'].append(ele)
option = input('Select Addition, Subtraction, Multiplication, Division: ')
if option.lower() == "addition":
print(numpy.polynomial.polynomial.polyadd(poly['px'], poly['qx']))
if option.lower() == "subtraction":
print(numpy.polynomial.polynomial.polysub(poly['px'], poly['qx']))
if option.lower() == "multiplication":
total = 0
for i in range(px_terms):
ans = poly['px'][i] * poly['qx'][i]
print(f"answer{i+1} = ", ans)
total += ans
print(f"total = {total}")
if option.lower() == "division":
qu, re = numpy.polynomial.polynomial.polydiv(poly['px'], poly['qx'])
print("Quotient: ", qu)
print("Remainder: ", re)

Step by step
Solved in 5 steps with 4 images

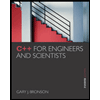
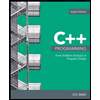
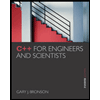
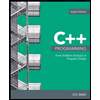