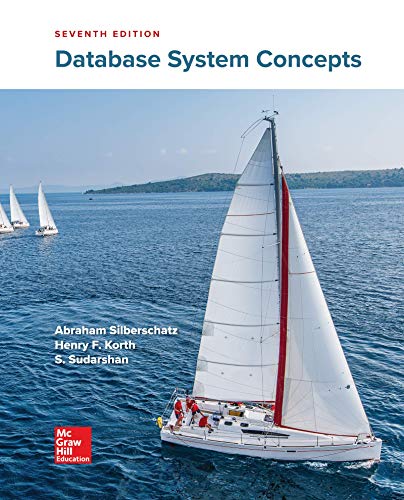
Concept explainers
3. Debugging Lists
File: debugging.py
Similar to the previous practical's debugging exercises:
- Copy the code from debugging.py
- debugging.py -
"""CP1401 - Practical 8 - Debugging."""
# Debug
program 1 - friends' names
names = ["Abby", "Jerome", "Olivia", "Monique"]
print("My friends' names: ")
for i in range(1, len(names)):
print(f"{i}. {names[i]}")
last_number = len(names)
print(f"The last name (number {last_number}) is {names[last_number]}")# Problem(s) for program 1:
# ?# Fixed code for program 1:
# Debug program 2 - title-casing country names
countries = ("australia", "new zeaLAND", "India")
for i in range(len(countries)):
countries[i] = countries[i].title()
print(countries) # country names should be in title-case now# Problem(s) for program 2:
# ?# Fixed code for program 2:
- Run the code and see what problems you can find.
- Complete the template in this file, adding comments to explain the problems (bugs) you find.
- Remember: debugging is about finding bugs, not just generally improving code. We're not interested in formatting and style here, just functionality.
- Then, comment-out the broken code and include fixed code in the provided section.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

- PYTHON ONLY AND PLEASE COMMENT CODE 1. A) write a For loop that iterates through a list of products (any number of products) that are sold in a store and prints them. [examples: fan, fridge, freezer, washer, etc.]. b) copy the code from a) and use the same loop to add more products(any)to the list. You must use list methods to add. c) Copy the code from c), and search for a specific product (any), if the product is found the loop is terminated. Make sure to use else in the for loop. d) Create an empty dictionary, then add 4 entries (the key is a word, the value is word’s frequency in a book). [example: {‘street’: 23}]. e) Create a for loop to access the entries from d) and print the keys and values. f) Copy the code from e) and search for a specific key in the dictionary and prints its value. The program prints a message “ the {key} is not found”, if the key does not exist.arrow_forward*Coding language is Python Write a program that opens the productsales.txt file and reads the sales into a list. The program should output the following information, in this order: The total of all sales in the list The average of all sales in the list The lowest sale in the list The highest sale in the list NOTE: Your program should include at least one user-defined function, in addition to the main function. 4147 1594 2235 8433 10000 129 5555 7030 9764 7465 1111 4444 8954 2243 2895 1436 4978 5486 1436 9846 4789 8456 2497 2280 6375arrow_forwardUsing only two lines, write the C++ code to print out the contents of the ideas array using a for loop in the space provided. std::string ideas[3]{ "Jalapeno Mustard", "Jalapeno IceCrea m", "Jalapeno Cake" }; The output of the code will be the following three strings: Jalapeno Mustard Jalapeno IceCream Jalapeno Cake Edit View Inscrt Format Tools Table 12pt~ Paragraph B IU A Tarrow_forward
- : Animating a Plot Problem: The MATLAB code with the initial conditions and one dimensional motion equation are given in the MProject2_Assignment1_base.m file. Modify the code to explore how to animate the plot, use functions to create plot functionarrow_forwardQuestion-4 Iteration, List and Strings For the given birthday list use a for loop to construct two lists of • months • year Note: birthdays are in the form of MM/DD/YYYYarrow_forwardTopic: Array covered in Chapter 7 Do not use any topic not covered in lecture. Write a C++ program to store and process an integer array. The program creates an array (numbers) of integers that can store up to 10 numbers. The program then asks the user to enter up to a maximum of 10 numbers, or enter 999 if there are less than 10 numbers. The program should store the numbers in the array. Then the program goes through the array to display all even (divisible by 2) numbers in the array that are entered by the user. Then the program goes through the array to display all odd (not divisible by 2) numbers in the array that are entered by the user. At the end, the program displays the total sum of all numbers that are entered by the user.arrow_forward
- Complete the Funnyville High School registration program where user is prompted for her full name and the program generates email id and temporary password. Sample run: generate_EmailID: This function takes two arguments: the user’s first name and last name and creates and returns the email id as a string by using these rules: The email id is all lower case. email id is of the form “last.first@fhs.edu”. e.g. For "John Doe" it will be "doe.john@fhs.edu". See sample runs above. generate_Password: This function takes two arguments: the user’s first name and last name and generates and returns a temporary password as a string by using these rules. Assume that user's first and last names have at least 2 letters. The temporary password starts with the first 2 letters of the first name, made lower case. followed by a number which is the sum of the lengths of the first and last name (For example this number will be 7 for "John Doe" since length of "John" is 4 and length of "Doe" is…arrow_forwardLAB ASSIGNMENTS IMPORTANT: you should complete the PDP thinking process for each program. Turn in items: 1) book_list.py: you can adapt your Chapter 4 book_list.py Lab program. The program summarizes costs of a book list. It uses all of our standard mipo_ex features. In this version of the program you must use pylnputPlus functions to perform all the input validation and for the main() loop decision. Adjust your program to allow book prices to include $ and cents. Restrict individual book prices to $100 maxium. • Clearly document, with comments, your use of the pyip functions. This program summarizes a book liat. Enter the number of books that you need: Please enter a whole number: three Enter a number greater than 0: 3 Enter the name of book #1: The Mueller Report Enter cost of The Mueller Report, to the nearest dollar: Please enter a whole number: 18 Enter the name of book #2: Educated: A Memoir Enter cost of Educated: A Memoir, to the nearest dollar: Please enter a whole number: 24…arrow_forwardPYTHON!!! The credit plan at TidBit Computer Store specifies a 10% down payment and an annual interest rate of 12%. Monthly payments are 5% of the listed purchase price, minus the down payment. Write a program that takes the purchase price as input. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items: The month number (beginning with 1) The current total balance owed The interest owed for that month The amount of principal owed for that month The payment for that month The balance remaining after payment The amount of interest for a month is equal to balance × rate / 12. The amount of principal for a month is equal to the monthly payment minus the interest owed. An example of the program input and output is shown below: Enter the puchase price: 200 (SEE IMAGE FOR PURCHASE PRICE CHART) Results you should get: Input: 200 Output: Purchase price: 200…arrow_forward
- C++ Coding: Arrays Implement a two-dimensional character array. Use a nested loop to store a 12x6 flag. 5 x 2 stars as * Alternate = and – to represent stripe colors Use a nested loop to output the character array to the console.arrow_forwardcan somone help me with this in c languagearrow_forwardC Programming Write function checkHorizontal to count how many discs of the opposing player would be flipped, it should do the following a. Return type integer b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. char playerCharc. If the square to the left or right is a space, stop checking d. If the square to the left or right is the same character as the player’s character, save that it was a flank, stop checking e. If the square to the left or right is not the same character as the player’s character, count the disc f. If the counted discs is greater than zero AND the player found their own character, return the counted discs, otherwise return ZERO Write function checkVertical to count how many discs of the opposing player would be flipped, it should do the following a. Return type integer b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. char playerCharc. If the square above or below is a space, stop checking d. If the square above or below is…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
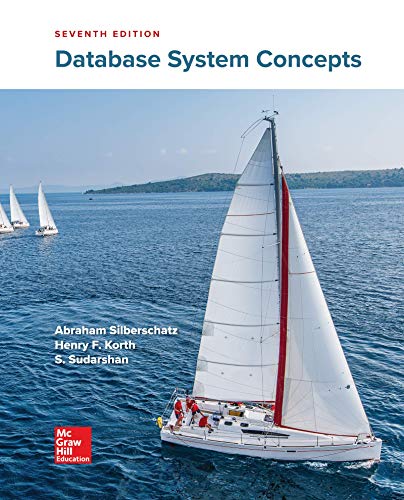
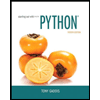
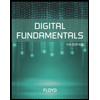
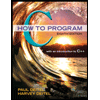
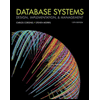
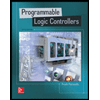