Can you please help with this Use the provided template below BASE_YEAR = 1903 #*************************************************************** # Function: main # # Description: The main function of the program # # Parameters: None # # Returns: Nothing # #************************************************************** def main(): # Local dictionary variables year_dict = {} count_dict = {} developerInfo() # Open the file for reading input_file = open('Program11.txt', 'r') # End of the main function #********************************************* # # Function: # # Description: # # Parameters: # # Returns: # #***************************************** def showResults(year_dict, count_dict): # Receive user input year = int(input('Enter a year in the range 1903-2018: ')) # Print results if year == 1904 or year == 1994: print("The world series wasn't played in the year", year) elif year < 1903 or year > 2018: print('The data for the year', year, \ 'is not included in our database.') else: winner = year_dict[year] wins = count_dict[winner] print('The team that won the world series in ', \ year, ' is the ', winner, '.', sep='') print('They have won the world series', wins, 'times.') # End of showResults #**************************************** # # Function: developerInfo # # Description: Prints Programmer's information # # Parameters: None # # Returns: Nothing # #*************************************** def developerInfo(): print('Name: ') print('Course: Programming Fundamentals I') print('Program: Eleven') print() # End of the developerInfo function # Call the main function. main() __________________________________________________________________ Here are the program details: The file, Program11.txt, contains a chronological list of the World Series’ winning teams from 1903 through 2018. The first line in the file is the name of the team that won in 1903, and the last line is the name of the team that won in 2018. (Note that the World Series was not played in 1904 or 1994. There are no entries in the file indicating this.) Write a program that reads this file and creates a dictionary in which the keys are the names of the teams and each key’s associated value is the number of times the team has won the World Series. It should also create a dictionary in which the keys are the years and each key’s associated value is the name of the team that won that year. The program should prompt the user for a year in the range of 1903 through 2018. It should then display the name of the team that won the World Series that year and the number of times that team has won the World Series. Allow the user to run the program as many times as possible until a sentinel value of zero (0) has been entered for the year. IMPORTANT: No input, processing, or output should happen in the main function. All work should be delegated to other functions. The program should have at least 4 functions (main and developerInfo included). Include the recommended minimum documentation for each function. Run your program at least five times with 1903, 1994, 2009, 2016, and 2018 as the user input for the year. This is the input info Boston Americans New York Giants Chicago White Sox Chicago Cubs Chicago Cubs Pittsburg Pirates Philadelphia Athletics Philadelphia Athletics Boston Red Sox Philadelphia Athletics Boston Braves Boston Red Sox Boston Red Sox Chicago White Sox Boston Red Sox Cincinnati Reds Cleveland Indians New York Giants New York Giants New York Yankees Washington Senators Pittsburgh Pirates St. Louis Cardinals New York Yankees New York Yankees Philadelphia Athletics Philadelphia Athletics St. Louis Cardinals New York Yankees New York Giants St. Louis Cardinals Detroit Tigers New York Yankees New York Yankees New York Yankees New York Yankees Cincinnati Reds New York Yankees St. Louis Cardinals New York Yankees St. Louis Cardinals Detroit Tigers St. Louis Cardinals New York Yankees Cleveland Indians New York Yankees New York Yankees New York Yankees New York Yankees New York Yankees New York Giants Brooklyn Dodgers New York Yankees Milwaukee Braves New York Yankees Los Angeles Dodgers Pittsburgh Pirates New York Yankees New York Yankees Los Angeles Dodgers St. Louis Cardinals Los Angeles Dodgers Baltimore Orioles St. Louis Cardinals Detroit Tigers New York Mets Baltimore Orioles Pittsburgh Pirates Oakland Athletics Oakland Athletics Oakland Athletics Cincinnati Reds Cincinnati Reds New York Yankees New York Yankees Pittsburgh Pirates Philadelphia Phillies Los Angeles Dodgers St. Louis Cardinals Baltimore Orioles Detroit Tigers Kansas City Royals New York Mets Minnesota Twins Los Angeles Dodgers Oakland Athletics Cincinnati Reds Minnesota Twins Toronto Blue Jays Toronto Blue Jays Atlanta Braves New York Yankees Florida Marlins New York Yankees New York Yankees New York Yankees Arizona Diamondbacks Anaheim Angels Florida Marlins Boston Red Sox Chicago White Sox St. Louis Cardinals Boston Red Sox Philadelphia Phillies New York Yankees San Francisco Giants St. Louis Cardinals San Francisco Giants Boston Red Sox San Francisco Giants Kansas City Royals Chicago Cubs Houston Astros Boston Red Sox
Can you please help with this
Use the provided template below
BASE_YEAR = 1903
#***************************************************************
# Function: main
#
# Description: The main function of the program
#
# Parameters: None
#
# Returns: Nothing
#
#**************************************************************
def main():
# Local dictionary variables
year_dict = {}
count_dict = {}
developerInfo()
# Open the file for reading
input_file = open('Program11.txt', 'r')
# End of the main function
#*********************************************
#
# Function:
#
# Description:
#
# Parameters:
#
# Returns:
#
#*****************************************
def showResults(year_dict, count_dict):
# Receive user input
year = int(input('Enter a year in the range 1903-2018: '))
# Print results
if year == 1904 or year == 1994:
print("The world series wasn't played in the year", year)
elif year < 1903 or year > 2018:
print('The data for the year', year, \
'is not included in our
else:
winner = year_dict[year]
wins = count_dict[winner]
print('The team that won the world series in ', \
year, ' is the ', winner, '.', sep='')
print('They have won the world series', wins, 'times.')
# End of showResults
#****************************************
#
# Function: developerInfo
#
# Description: Prints Programmer's information
#
# Parameters: None
#
# Returns: Nothing
#
#***************************************
def developerInfo():
print('Name: <Put your full name here>')
print('Course:
print('Program: Eleven')
print()
# End of the developerInfo function
# Call the main function.
main()
__________________________________________________________________
Here are the program details:
The file, Program11.txt, contains a chronological list of the World Series’ winning teams from 1903 through 2018. The first line in the file is the name of the team that won in 1903, and the last line is the name of the team that won in 2018. (Note that the World Series was not played in 1904 or 1994. There are no entries in the file indicating this.)
Write a program that reads this file and creates a dictionary in which the keys are the names of the teams and each key’s associated value is the number of times the team has won the World Series.
It should also create a dictionary in which the keys are the years and each key’s associated value is the name of the team that won that year.
The program should prompt the user for a year in the range of 1903 through 2018.
It should then display the name of the team that won the World Series that year and the number of times that team has won the World Series.
Allow the user to run the program as many times as possible until a sentinel value of zero (0) has been entered for the year.
IMPORTANT: No input, processing, or output should happen in the main function. All work should be delegated to other functions. The program should have at least 4 functions (main and developerInfo included).
Include the recommended minimum documentation for each function.
Run your program at least five times with 1903, 1994, 2009, 2016, and 2018 as the user input for the year.
This is the input info
Boston Americans
New York Giants
Chicago White Sox
Chicago Cubs
Chicago Cubs
Pittsburg Pirates
Philadelphia Athletics
Philadelphia Athletics
Boston Red Sox
Philadelphia Athletics
Boston Braves
Boston Red Sox
Boston Red Sox
Chicago White Sox
Boston Red Sox
Cincinnati Reds
Cleveland Indians
New York Giants
New York Giants
New York Yankees
Washington Senators
Pittsburgh Pirates
St. Louis Cardinals
New York Yankees
New York Yankees
Philadelphia Athletics
Philadelphia Athletics
St. Louis Cardinals
New York Yankees
New York Giants
St. Louis Cardinals
Detroit Tigers
New York Yankees
New York Yankees
New York Yankees
New York Yankees
Cincinnati Reds
New York Yankees
St. Louis Cardinals
New York Yankees
St. Louis Cardinals
Detroit Tigers
St. Louis Cardinals
New York Yankees
Cleveland Indians
New York Yankees
New York Yankees
New York Yankees
New York Yankees
New York Yankees
New York Giants
Brooklyn Dodgers
New York Yankees
Milwaukee Braves
New York Yankees
Los Angeles Dodgers
Pittsburgh Pirates
New York Yankees
New York Yankees
Los Angeles Dodgers
St. Louis Cardinals
Los Angeles Dodgers
Baltimore Orioles
St. Louis Cardinals
Detroit Tigers
New York Mets
Baltimore Orioles
Pittsburgh Pirates
Oakland Athletics
Oakland Athletics
Oakland Athletics
Cincinnati Reds
Cincinnati Reds
New York Yankees
New York Yankees
Pittsburgh Pirates
Philadelphia Phillies
Los Angeles Dodgers
St. Louis Cardinals
Baltimore Orioles
Detroit Tigers
Kansas City Royals
New York Mets
Minnesota Twins
Los Angeles Dodgers
Oakland Athletics
Cincinnati Reds
Minnesota Twins
Toronto Blue Jays
Toronto Blue Jays
Atlanta Braves
New York Yankees
Florida Marlins
New York Yankees
New York Yankees
New York Yankees
Arizona Diamondbacks
Anaheim Angels
Florida Marlins
Boston Red Sox
Chicago White Sox
St. Louis Cardinals
Boston Red Sox
Philadelphia Phillies
New York Yankees
San Francisco Giants
St. Louis Cardinals
San Francisco Giants
Boston Red Sox
San Francisco Giants
Kansas City Royals
Chicago Cubs
Houston Astros
Boston Red Sox

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

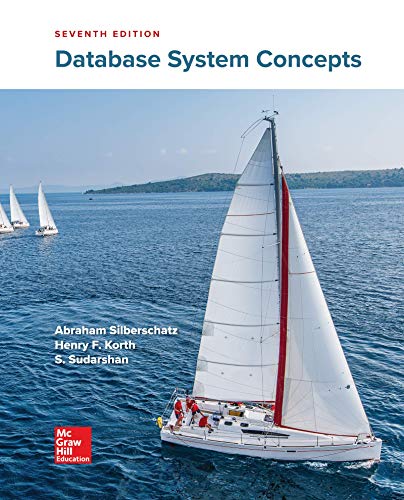
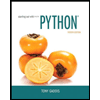
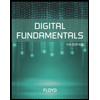
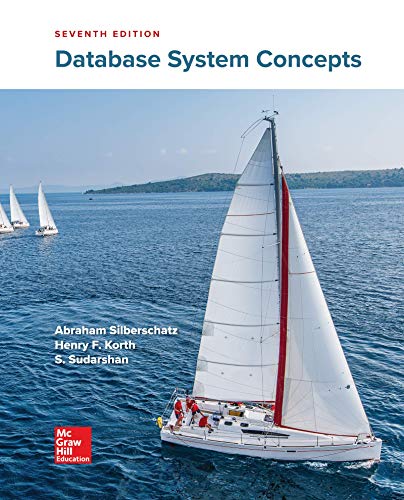
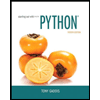
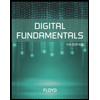
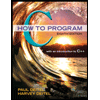
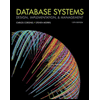
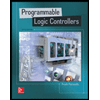