//******************************************************************************// Program name: Lab4a//// Description: //// What's on your mind about this lab? //// Author: //// Date: //// IDE Used: //// Template Date: 04/06/2021//****************************************************************************** #include #include #include using namespace std;// Write the code for class RealProperty here . . .// Write the code for class Apartment here . . .// Function prototypes// Declare a function named displayPropertyInfo that receives one reference to const parameter// representing a real property. The function has no return value.// Declare a function named displayApartmentInfo that receives one reference to const parameter// representing an apartment. The function has no return value.int main() { // Write code here . . . return 0;}//******************************************************************************//* Print the real property information.//*//* Parameter//* rp - a reference to const referencing to caller's RealProperty//* variable.//*//* Return//* void//******************************************************************************void displayPropertyInfo(const RealProperty &rp) { // Write your code here . . .
//******************************************************************************// Program name: Lab4a//// Description: //// What's on your mind about this lab? //// Author: //// Date: //// IDE Used: //// Template Date: 04/06/2021//****************************************************************************** #include #include #include using namespace std;// Write the code for class RealProperty here . . .// Write the code for class Apartment here . . .// Function prototypes// Declare a function named displayPropertyInfo that receives one reference to const parameter// representing a real property. The function has no return value.// Declare a function named displayApartmentInfo that receives one reference to const parameter// representing an apartment. The function has no return value.int main() { // Write code here . . . return 0;}//******************************************************************************//* Print the real property information.//*//* Parameter//* rp - a reference to const referencing to caller's RealProperty//* variable.//*//* Return//* void//******************************************************************************void displayPropertyInfo(const RealProperty &rp) { // Write your code here . . .
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
//******************************************************************************// Program name: Lab4a//// Description: <Write the program description here.>//// What's on your mind about this lab? <Write what you've learned here.>//// Author: <Write your name here.>//// Date: <Write the date you start writing the program in mm/dd/yyyy.>//// IDE Used: <Write the IDE you use here.>//// Template Date: 04/06/2021//******************************************************************************
#include <iostream>#include <iomanip>#include <string>using namespace std;// Write the code for class RealProperty here . . .// Write the code for class Apartment here . . .// Function prototypes// Declare a function named displayPropertyInfo that receives one reference to const parameter// representing a real property. The function has no return value.// Declare a function named displayApartmentInfo that receives one reference to const parameter// representing an apartment. The function has no return value.int main() { // Write code here . . . return 0;}//******************************************************************************//* Print the real property information.//*//* Parameter//* rp - a reference to const referencing to caller's RealProperty//* variable.//*//* Return//* void//******************************************************************************void displayPropertyInfo(const RealProperty &rp) { // Write your code here . . .
}//******************************************************************************//* Print the apartment information.//*//* Parameter//* rp - a reference to const referencing to caller's Apartment//* variable.//*//* Return//* void//******************************************************************************void displayApartmentInfo(const Apartment &apt) { // Write your code here . . .}
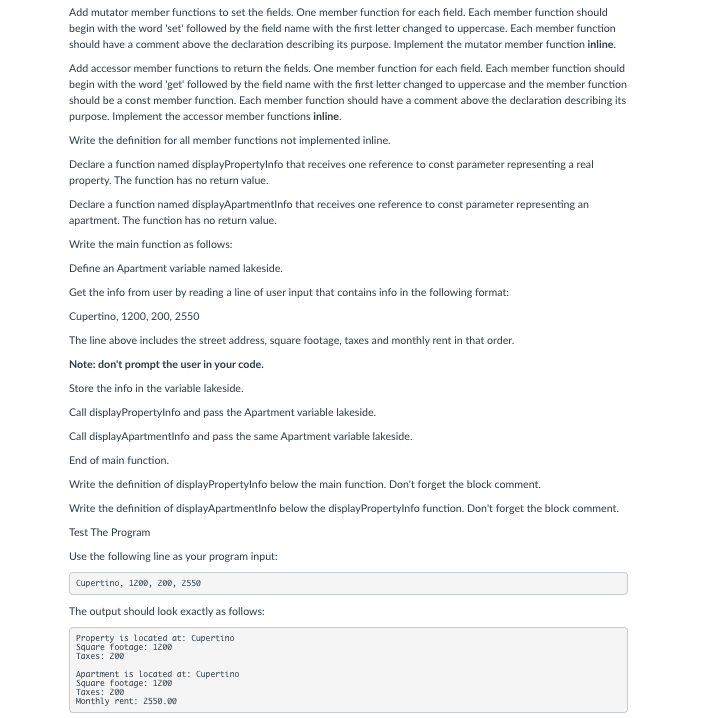
Transcribed Image Text:Add mutator member functions to set the fields. One member function for each field. Each member function should
begin with the word 'set' followed by the field name with the first letter changed to uppercase. Each member function
should have a comment above the declaration describing its purpose. Implement the mutator member function inline.
Add accessor member functions to return the fields. One member function for each field. Each member function should
begin with the word 'get' followed by the field name with the first letter changed to uppercase and the member function
should be a const member function. Each member function should have a comment above the declaration describing its
purpose. Implement the accessor member functions inline.
Write the definition for all member functions not implemented inline.
Declare a function named displayPropertylnfo that receives one reference to const parameter representing a real
property. The function has no return value.
Declare a function named displayApartmentlnfo that receives one reference to const parameter representing an
apartment. The function has no return value.
Write the main function as follows:
Define an Apartment variable named lakeside.
Get the info from user by reading a line of user input that contains info in the following format:
Cupertino, 1200, 200, 2550
The line above includes the street address, square footage, taxes and monthly rent in that order.
Note: don't prompt the user in your code.
Store the info in the variable lakeside.
Call displayPropertylnfo and pass the Apartment variable lakeside.
Call displayApartmentlnfo and pass the same Apartment variable lakeside.
End of main function.
Write the definition of displayPropertylnfo below the main function. Don't forget the block comment.
Write the definition of displayApartmentlnfo below the displayPropertylnfo function. Don't forget the block comment.
Test The Program
Use the following line as your program input:
Cupertino, 1200, 200, 2550
The output should look exactly as follows:
Property is located at: Cupertino
Square footage: 1200
Taxes: 200
Apartment is located at: Cupertino
Square footage: 1200
Taxes: 200
Monthly rent: 2550.00
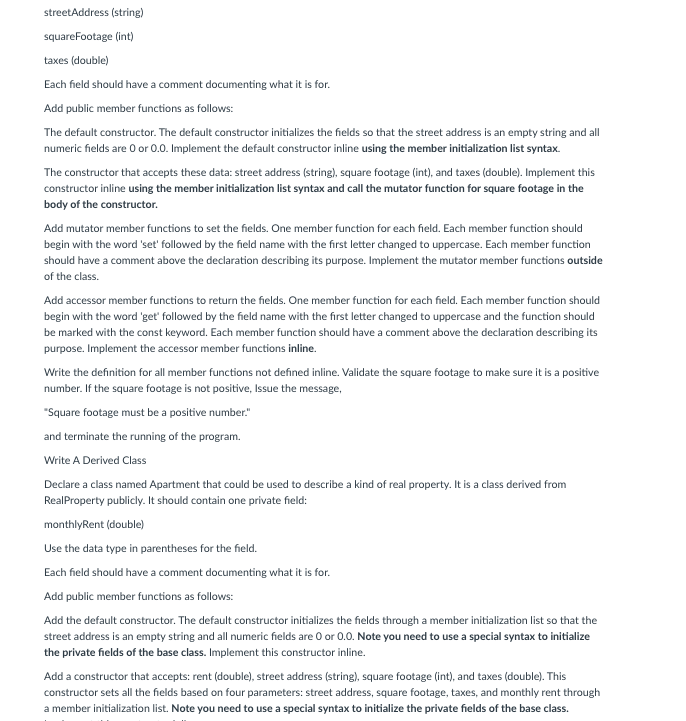
Transcribed Image Text:streetAddress (string)
squareFootage (int)
taxes (double)
Each field should have a comment documenting what it is for.
Add public member functions as follows:
The default constructor. The default constructor initializes the fields so that the street address is an empty string and all
numeric fields are O or 0.0. Implement the default constructor inline using the member initialization list syntax.
The constructor that accepts these data: street address (string), square footage (int), and taxes (double). Implement this
constructor inline using the member initialization list syntax and call the mutator function for square footage in the
body of the constructor.
Add mutator member functions to set the fields. One member function for each field. Each member function should
begin with the word 'set' followed by the field name with the first letter changed to uppercase. Each member function
should have a comment above the declaration describing its purpose. Implement the mutator member functions outside
of the class.
Add accessor member functions to return the fields. One member function for each field. Each member function should
begin with the word 'get followed by the field name with the first letter changed to uppercase and the function should
be marked with the const keyword. Each member function should have a comment above the declaration describing its
purpose. Implement the accessor member functions inline.
Write the definition for all member functions not defined inline. Validate the square footage to make sure it is a positive
number. If the square footage is not positive, Issue the message,
"Square footage must be a positive number."
and terminate the running of the program.
Write A Derived Class
Declare a class named Apartment that could be used to describe a kind of real property. It is a class derived from
RealProperty publicly. It should contain one private field:
monthlyRent (double)
Use the data type in parentheses for the field.
Each field should have a comment documenting what it is for.
Add public member functions as follows:
Add the default constructor. The default constructor initializes the fields through a member initialization list so that the
street address is an empty string and all numeric fields are O or 0.0. Note you need to use a special syntax to initialize
the private fields of the base class. Implement this constructor inline.
Add a constructor that accepts: rent (double), street address (string), square footage (int), and taxes (double). This
constructor sets all the fields based on four parameters: street address, square footage, taxes, and monthly rent through
a member initialization list. Note you need to use a special syntax to initialize the private fields of the base class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
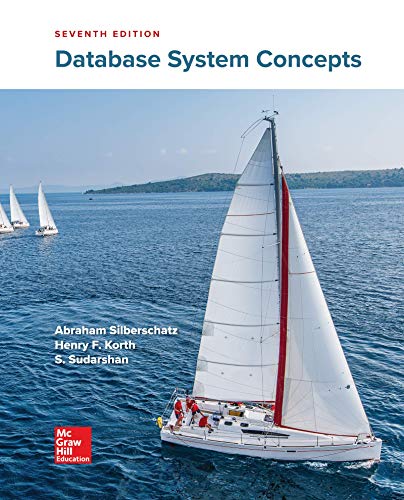
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
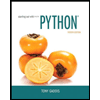
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
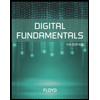
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
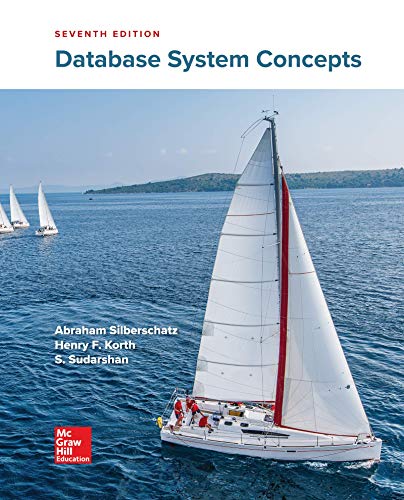
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
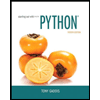
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
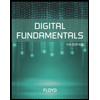
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
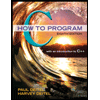
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
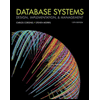
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
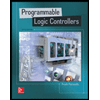
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education