Change this cpp code to C language #include using namespace std; /* Link list node */ struct Node { int data; struct Node* next; Node(int data) { this->data = data; next = NULL; } }; /*This Function takes head of Linked list as parameter */ /* and reverses the linked list by using an iterative approach*/ Node* reverse_iter(Node* head) { // Initializing the current,next and previous pointers Node* current = head; Node *prev = NULL, *next = NULL; while (current != NULL) { // Storing the next pointer next = current->next; // Reverse the current node's pointer current->next = prev; // Moving the pointers one position ahead. prev = current; current = next; } head = prev; return head; } /*This Function takes head of Linked list as parameter */ /* and reverses the linked list by using an recursive approach*/ Node* reverse_rec(Node* head) { //If there is no element or only one element in the list then simply return head. if (head == NULL || head->next == NULL) return head; /* Calling the same function recursively which reverses the rest list and put the first element at the end */ Node* rest = reverse_rec(head->next); head->next->next = head; head->next = NULL; /* This fixes the head pointer in every recursive call*/ return rest; } /* This Function prints the linked list */ void print(Node* head) { struct Node* temp = head; while (temp != NULL) { cout << temp->data << " "; temp = temp->next; } } /* This Function inserts the data into Linked list */ Node* push(Node* head, int data) { Node* temp = new Node(data); temp->next = head; head = temp; return head; } int main() { Node* head = NULL, *newhead, *updatedhead; /* Start with the empty list */ newhead = push(head, 10); newhead = push(newhead, 20); newhead = push(newhead, 30); newhead = push(newhead, 40); newhead = push(newhead, 50); newhead = push(newhead, 60); cout << "Given linked list\n"; print(newhead); updatedhead = reverse_iter(newhead); cout << "\n\nReversed Linked list using Iterative Approach \n"; print(updatedhead); head = NULL; /* Start with the empty list */ newhead = push(head, 10); newhead = push(newhead, 20); newhead = push(newhead, 30); newhead = push(newhead, 40); newhead = push(newhead, 50); newhead = push(newhead, 60); updatedhead = reverse_rec(newhead); cout << "\n\nReversed Linked list using Recursive Approach \n"; print(updatedhead); return 0; }
Change this cpp code to C language
#include <iostream>
using namespace std;
/* Link list node */
struct Node {
int data;
struct Node* next;
Node(int data)
{
this->data = data;
next = NULL;
}
};
/*This Function takes head of Linked list as parameter */
/* and reverses the linked list by using an iterative approach*/
Node* reverse_iter(Node* head)
{
// Initializing the current,next and previous pointers
Node* current = head;
Node *prev = NULL, *next = NULL;
while (current != NULL) {
// Storing the next pointer
next = current->next;
// Reverse the current node's pointer
current->next = prev;
// Moving the pointers one position ahead.
prev = current;
current = next;
}
head = prev;
return head;
}
/*This Function takes head of Linked list as parameter */
/* and reverses the linked list by using an recursive approach*/
Node* reverse_rec(Node* head)
{
//If there is no element or only one element in the list then simply return head.
if (head == NULL || head->next == NULL)
return head;
/* Calling the same function recursively which reverses the rest list and put the first element at the end */
Node* rest = reverse_rec(head->next);
head->next->next = head;
head->next = NULL;
/* This fixes the head pointer in every recursive call*/
return rest;
}
/* This Function prints the linked list */
void print(Node* head)
{
struct Node* temp = head;
while (temp != NULL) {
cout << temp->data << " ";
temp = temp->next;
}
}
/* This Function inserts the data into Linked list */
Node* push(Node* head, int data)
{
Node* temp = new Node(data);
temp->next = head;
head = temp;
return head;
}
int main()
{
Node* head = NULL, *newhead, *updatedhead;
/* Start with the empty list */
newhead = push(head, 10);
newhead = push(newhead, 20);
newhead = push(newhead, 30);
newhead = push(newhead, 40);
newhead = push(newhead, 50);
newhead = push(newhead, 60);
cout << "Given linked list\n";
print(newhead);
updatedhead = reverse_iter(newhead);
cout << "\n\nReversed Linked list using Iterative Approach \n";
print(updatedhead);
head = NULL;
/* Start with the empty list */
newhead = push(head, 10);
newhead = push(newhead, 20);
newhead = push(newhead, 30);
newhead = push(newhead, 40);
newhead = push(newhead, 50);
newhead = push(newhead, 60);
updatedhead = reverse_rec(newhead);
cout << "\n\nReversed Linked list using Recursive Approach \n";
print(updatedhead);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

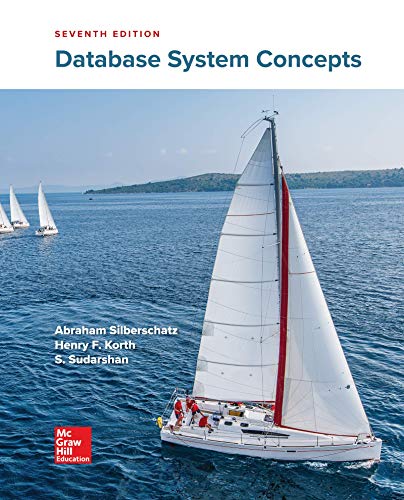
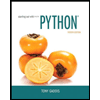
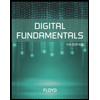
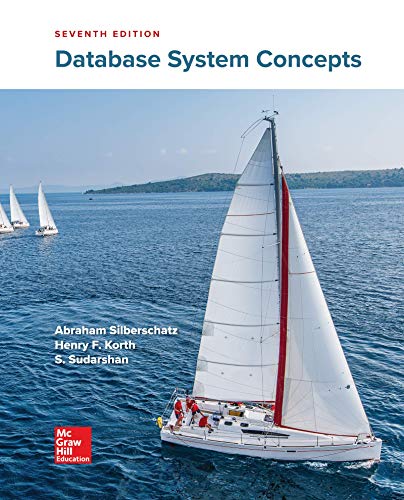
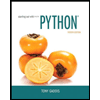
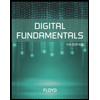
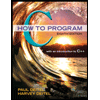
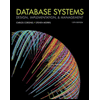
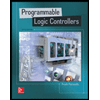