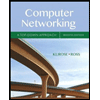
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
In C program. Implement list_removeFront( list_t* list ) function.
/*
* dataStructure.h
*
* Provides a data structure made of a doubly-headed singly-linked list (DHSL)
* of unsorted elements (integers) in which duplicated elements are allowed.
*
* A DHSL list is comprised of a single header and zero or more elements.
* The header contains pointers to the first and last elements in the list,
* or NULL if the list is empty. The first element contains a pointer to
* the next element, and so on. The last element in the list has its
* "next" pointer set to NULL.
*
* The interface of this data structure includes several utility
* functions that operate on this data structure.
*
* Precondition: Functions that operate on such data structure
* require a valid pointer to it as their first argument.
*
* Do not change this dataStructure.h file.
*
*/
/*** Data structure ***/
/* List element (node): our DHSL list is a chain of these nodes. */
typedef struct element {
int val;
structelement*next;
} element_t;
/* List header: keeps track of the first and last list elements
as well as the number of elements stored in our data structure. */
typedef struct list {
element_t* head;
element_t* tail;
unsignedint elementCount;
} list_t;
/*** Data structure interface ***/
/* A type for returning status codes */
typedef enum {
OK,
ERROR,
NULL_PARAM
} result_t;
//dataStructure.c
/* Description: Removes the front (first) element (node) in the data
* structure pointed to by "list" and returns OK.
* If no elements (no nodes) can be removed, leaves the
* data structure unmodified and returns ERROR.
* Returns NULL_PARAM if "list" is NULL.
* Time efficiency: O(1)
*/
result_t list_removeFront( list_t* list ) {
result_t result = OK;
// Stubbing this function
// This stub is to be removed when you have successfully implemented this function, i.e.,
// ***Remove this call to printf!***
printf( "Calling list_removeFront(...): remove first element at the front of the list.\n" );
return result;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- def reverse_list (1st: List [Any], start: int, end: int) -> None: """Reverse the order of the items in list , between the indexes and (including the item at the index). If start or end are out of the list bounds, then raise an IndexError. The function must be implemented **recursively**. >>> lst ['2', '2', 'v', 'e'] >>>reverse_list (lst, 0, 3) >>> lst ['e', 'v', 'i', '2'] >>> lst [] >>>reverse_list (lst, 0, 0) >>> lst [0] >>> Ist = [] >>> reverse_list (lst, 0, 1) Traceback (most recent call last): IndexError #1 #1arrow_forwardC++ Create a generic function add_bookends(ls, e) that adds a copy of element e to the front and back of list ls.arrow_forwardConsider the following function access_element_by_index that returns the iterator to the element at the index i in the list l. typedef std::list<int> int_list; int_list::iterator access_element_by_index(size_t I, int_list &) { assert( ? ); … } Assume the first element of the list is at the index 0, the second at the index 1, and so on. If the function is required to return an iterator that can be dereferenced, determine the precondition of the function, and write an assertion to validate it. (You don’t need to implement the functions).arrow_forward
- Given an IntNode struct and the operating functions for a linked list, complete the following functions to extend the functionality of the linked list(this is not graded).arrow_forwardPascal triangle! write a function(in OCaml) val nextpascalrow : int list -> int list = that given a list of integers that correspond to a row of the pascal triangle, it calculates the next row of the pascal triangle. # nextpascalrow [1; 6; 15; 20; 15; 6; 1];; - : int list = [1; 7; 21; 35; 35; 21; 7; 1]arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
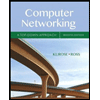
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
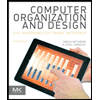
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
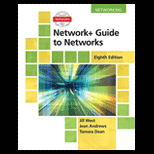
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
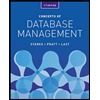
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
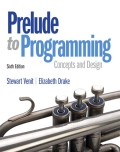
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
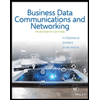
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY