check the Coin class and cointester program below. Write a new method .flipCoins(), that flips two (2) coins: the current coin and the coin passed as a parameter. The method returns the integer addition of the flipSides of both coins. Example, as if used in the CointTester program class: Coin bobCoin = new Coin(); // create Coin object [using Coin() constructor] Coin aliceCoin = new Coin(6); // create Coin object with 6 sides int combinedFlip = 0; // combined flip value of two coins combinedFlip = bobCoin.flipCoins(aliceCoin); // flip both coins, returned combined System.out.println ("Flip of two coins: " + combinedFlip); Coin class:
check the Coin class and cointester program below.
Write a new method .flipCoins(), that flips two (2) coins: the current coin and the coin passed as a parameter. The method returns the integer addition of the flipSides of both coins.
Example, as if used in the CointTester program class:
Coin bobCoin = new Coin(); // create Coin object [using Coin() constructor]
Coin aliceCoin = new Coin(6); // create Coin object with 6 sides
int combinedFlip = 0; // combined flip value of two coins
combinedFlip = bobCoin.flipCoins(aliceCoin); // flip both coins, returned combined
System.out.println ("Flip of two coins: " + combinedFlip);
Coin class:
import java.util.Random;
blic class Coin
{
private int numSides = 2;
private int flipSide = 0;
private static int coinCounter = 0;
private int uniqueID;
/** default constructor for Coin class
*/
public Coin ()
{
coinCounter++;
uniqueID = coinCounter;
}
/** constructor that sets the sides for Coin class
* @param numSides the number of sides of the coin
*/
public Coin (int numSides)
{
this.numSides = numSides;
coinCounter++;
uniqueID = coinCounter;
}
/** return the flipside (number)
* @return numeric value of flipside
*/
public int get()
{
return flipSide;
}
/** return the "name" of the face; Multi # for coins with more than 2 sides
* @return "name" of face (Heads, Tails, Multi #)
*/
public String getFace()
{
String face = "";
if ( numSides > 2 )
{
face = "Multi "+flipSide;
}
else if ( flipSide == 0 )
{
face = "Heads";
}
else if ( flipSide == 1 )
{
face = "Tails";
}
return (face);
}
/** set the side of the coin, numeric, within the range of numSides
* @param side force flipside to side, but only if within range 0..(numsides-1)
*/
public void set (int side)
{
//if ( side == 0 || side == 1)
if ( 0 <= side && side < numSides )
{
flipSide = side; // set the side of the coin
}
}
/** randomly flip the coin within the range of numsides
*/
public void flip()
{
Random rand = new Random();
flipSide = rand.nextInt(numSides);
}
/** compare two coins for flipSide
* @param that the other coin object
* @return true if flipsides are equal; false otherwise
*/
public boolean equals (Coin that)
{
boolean equal = false;
equal = (this.flipSide == that.flipSide);
return (equal);
}
/** returns a formatted string describing the coin, number of sides and the face
* @return formatted string with coin description
*/
public String toString()
{
String ret = String.format ("ID: %d, # of sides: %d, flip side: %s", uniqueID, numSides, getFace() );
return (ret);
}
}/
Tester :
import java.util.*;
public class CoinTester
{
public static void main (String[] args)
{
Coin bobCoin = new Coin();
Coin aliceCoin = new Coin(6);
Coin ashCoin = new Coin(1000);
/* testing idea of two Coin references pointing to the same Coin object.
ashCoin = bobCoin; // "assign" bobCoin to ashCoin
System.out.println ("bobCoin : " + bobCoin); // automatically call .toString()
System.out.println ("ashCoin : " + ashCoin);
*/
System.out.println ("bobCoin : " + bobCoin);
System.out.println ("aliceCoin : " + aliceCoin);
System.out.println ("ashCoin : " + ashCoin);
System.out.println ();
coinSetSide( bobCoin, 1 );
System.out.println ("bobCoin : " + bobCoin);
coinSetSide( ashCoin, 15 );
System.out.println ("ashCoin : " + ashCoin);
System.out.println ("bobCoin is : " + bobCoin );
System.out.println ("aliceCoin is : " + aliceCoin );
// bobCoin
bobCoin.flip();
System.out.println ("B Side of coin after flip: " + bobCoin.get() );
System.out.println ("B Face of coin after flip: " + bobCoin.getFace() );
// aliceCoin
aliceCoin.flip();
System.out.println ("A Side of coin after flip: " + aliceCoin.get() );
System.out.println ("A Face of coin after flip: " + aliceCoin.getFace() );
System.out.println();
// check for equality?
if ( bobCoin.equals(aliceCoin) )
//if ( aliceCoin.equals(bobCoin) )
{
System.out.println ("Coins are equal.");
}
else
{
System.out.println ("Coins are NOT equal.");
}
System.out.println ();
System.out.println ("Test to show that Java RNG is \"fair\" ");
int countHeads = 0;
int countTails = 0;
//int countOther = 0;
int numberOfFlips = 1000;
for (int i=1; i<=numberOfFlips; i++)
{
bobCoin.flip();
// Technique A (with if_else): determine which side of the coin is appearing
if ( bobCoin.getFace().equals("Heads") )
{
countHeads++; // add 1 to count of heads
}
else if ( bobCoin.getFace().equals("Tails") ) appearing
{
countTails++;
}
/* // Technique B (with switch): determine which side of the coin is appearing
switch ( bobCoin.getFace() )
{
case "Heads":
countHeads++;
break;
case "Tails":
countTails++;
break;
}
*/
}
System.out.println ("For a total of " + numberOfFlips + " flips");
System.out.println ("Count of Heads: " + countHeads);
System.out.println ("Count of Tails: " + countTails);
System.out.printf ("%% of Heads: %.3f \n", (1.0*countHeads/numberOfFlips)*100 );
System.out.printf ("%% of Tails: %.3f \n", (1.0*countTails/numberOfFlips)*100 );
/** set the side of a coin (with a method) */
public static void coinSetSide (Coin theCoin, int side)
{
System.out.println ("Coin: " + theCoin + ", to side: " + side);
theCoin.set(side);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

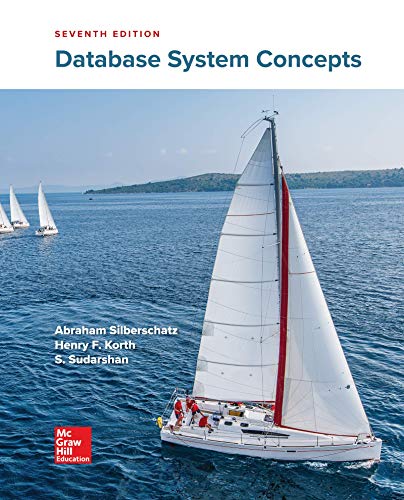
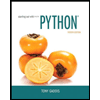
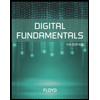
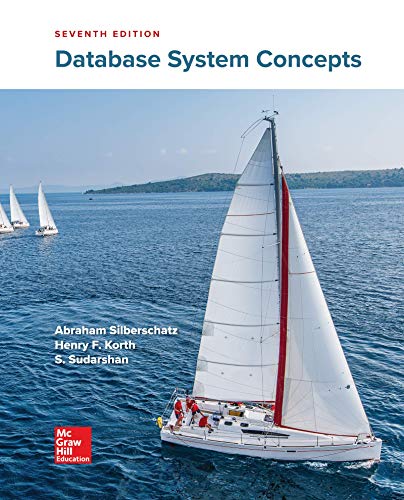
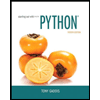
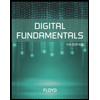
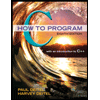
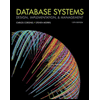
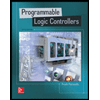