Consider the following definition of class Person: 1 public class Person 2 { 3 public final String name; 4 5 public Person(String name) 6 { 7 if (name == null) this.name = ""; //No null names... 8 else this.name = name; 9 } 10 } and the definition of class PersonListUtils implemented by a student... 1 public class PersonListUtils 2 { 3 //Replaces the first occurrence of a Person in the list with the given oldPersonName with a new Person having the newPersonName. 4 //Returns true if the substitution is successful, false otherwise 5 public static boolean substitute(ArrayList list, String oldPersonName, String newPersonName) 6 { 7 for (Person person: list) { 8 if (person.name == oldPersonName) { 9 person = new Person(newPersonName); 10 return true; 11 } 12 } 13 return false; 14 } 15 16 //Removes from the list the first occurrence of a Person with the given nameToRemove. 17 //Returns true if the deletion is successful, false otherwise 18 public static boolean remove(ArrayList list, String nameToRemove) 19 { 20 for (Person element: list) { 21 if (element.name == nameToRemove) { 22 return list.remove(element); 23 } 24 } 25 return false; 26 } 27 } Identify, characterize (i.e, determine if it is functional, compile-time, or run-time) and explain any errors you find in the definition of the class PersonListUtils (ignore the static keyword).
Consider the following definition of class Person:
1 public class Person
2 {
3 public final String name;
4
5 public Person(String name)
6 {
7 if (name == null) this.name = "<NoName>"; //No null names...
8 else this.name = name;
9 }
10 }
and the definition of class PersonListUtils implemented by a student...
1 public class PersonListUtils
2 {
3 //Replaces the first occurrence of a Person in the list with the given
oldPersonName with a new Person having the newPersonName.
4 //Returns true if the substitution is successful, false otherwise
5 public static boolean substitute(ArrayList<Person> list, String
oldPersonName, String newPersonName)
6 {
7 for (Person person: list) {
8 if (person.name == oldPersonName) {
9 person = new Person(newPersonName);
10 return true;
11 }
12 }
13 return false;
14 }
15
16 //Removes from the list the first occurrence of a Person with the given
nameToRemove.
17 //Returns true if the deletion is successful, false otherwise
18 public static boolean remove(ArrayList<Person> list, String nameToRemove)
19 {
20 for (Person element: list) {
21 if (element.name == nameToRemove) {
22 return list.remove(element);
23 }
24 }
25 return false;
26 }
27 }
Identify, characterize (i.e, determine if it is functional, compile-time, or run-time) and explain any errors you find
in the definition of the class PersonListUtils (ignore the static keyword).

Step by step
Solved in 2 steps

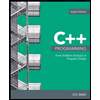
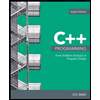