# Console Application for Minesweeper In this milestone, students will create three classes: Cell, Board, and Program. Create a class that models a game cell. A game cell should have the following properties: a.Its row and column. These should initially be set to -1. b.Its visited boolean value. This should initially be set to false.
C# Console Application for Minesweeper
In this milestone, students will create three classes: Cell, Board, and Program.
Create a class that models a game cell. A game cell should have the following properties:
a.Its row and column. These should initially be set to -1.
b.Its visited boolean value. This should initially be set to false.
c.Live boolean value. This should initially be set to false. "Live" set to true will indicate that the cell is a "live bomb" cell.
d.The number of neighbors that are "live." This should initially be set to 0.
The Cell class should have a constructor, as well as getters and setters for all properties.
3.Create a class that models a game board. A game board should have the following properties:
a.Size. The board will be square, where the size includes the dimensions of both the length and width of the board.
b.Grid. The grid will be a 2-dimensional array of the type cell.
c.Difficulty. A percentage of cells that will be set to "live" status.
4.The Board class should have the following methods:
a.The constructor for the Board should have a single parameter to set the size of the Grid. In its constructor, the Grid should be initialized so that a Cell object is stored at each location.
b.setupLiveNeighbors. A method to randomly initialize the grid with live bombs. The method should utilize the Difficulty property to determine what percentage of the cells in the grid will be set to "live" status.
c.calculateLiveNeighbors. A method to calculate the live neighbors for every cell on the grid. A cell should have between 0 and 8 live neighbors. If a cell itself is "live," then you can set the neighbor count to 9.
5.Program
a.The Program class should be the console app that drives the application. This is the class that should contain a main() method. The main program should have a printBoard helper method that uses, for loops, the Console.write and Console.writeLine commands to display the contents of the Board as shown at the beginning of these instructions.
6.The main() method should:
a.Create an instance of the Board class.
b.Call the Board.setupLiveNeighbors and Board.calculateLiveNeighbors commands to initialize the grid.
c.Call the printBoard method to display the contents of the grid.
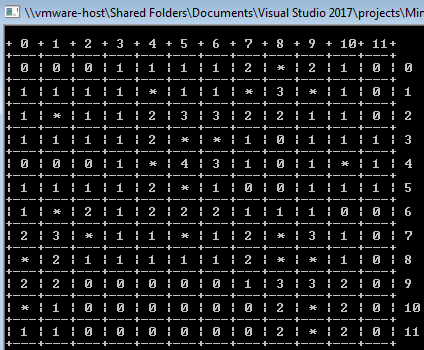

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

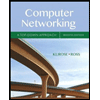
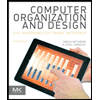
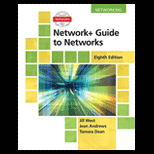
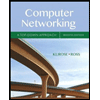
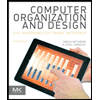
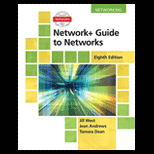
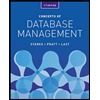
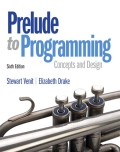
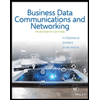