Create a car rental system where customers rent cars for a given period of time. You are given the following classes: Car– a class that has the following properties: car type: Mrcedes, Opel, Fiat, ....etc.. car license end date. car number. Necessary constructor and getters/setters. Small car class– inherits from the car and has the following constants: 4 passengers, Motor 1200, air-conditioner. Medium car class– inherits from the car and has the following constants: 4 passengers, Motor 1400, air-conditioner, Speed Control. Large car class- inherits from car and has the following constants: 6 passengers, Motor 1600, air-conditioner, speed control, Wi-Fi. Customer class: holds first name, last name and id number as member variables (of course with necessary constructor, getters and setters) . Rent class (representing the time period when the car is rent): holds startDate and endDate, rent price (of course with necessary constructor, getters and setters). In addition to the previous classes, Implement the following interface: rentInterface – holds a method displayRent(). The Rent Class implements this interface. Override the__toString() method for each class (of all previous classes) to print information about the object from the class in which it is instantiated. For example, suppose you have an object called someCar instantiated from small car class with values: "Opel", "20/9/2021", 12548754 (these values inherited from the car class), and the other contact values in the small car class. The toString() method in this case prints (for example): "The car type is Opel, the license end date is:20/9/2021 and the car number is:12548754. This car has 4 passengers size, Motor power of 1200 hp and equipped with an air-conditioner". This statement will be returned from the method toString(). Write a class rentingManager with a static method rentCar($car, $rent) that handles car renting and prints a message whether the operation was successful or not. This method takes two objects (car and rent) and adds them to an array of rents. This array contains the rents for each car. Of course, there must be no conflict in the dates of rents. Now, after writing the code of the previous classes and interface, create an array of different cars. Perform the following operations: Create an array of cars, in which the array contains different objects of the three types of cars (create the objects of the array and fill the objects with suitable data). Display the array by small cars and medium ones with a rent price less than or equal to 250.00 Display the array by all cars with an air conditioner. Return the car numbers of all cars which have a wi-fi service.
Create a car rental system where customers rent cars for a given period of time.
You are given the following classes:
-
Car– a class that has the following properties:
-
car type: Mrcedes, Opel, Fiat, ....etc..
-
car license end date.
-
car number.
-
Necessary constructor and getters/setters.
-
-
Small car class– inherits from the car and has the following constants: 4 passengers, Motor 1200, air-conditioner.
-
Medium car class– inherits from the car and has the following constants: 4 passengers, Motor 1400, air-conditioner, Speed Control.
-
Large car class- inherits from car and has the following constants: 6 passengers, Motor 1600, air-conditioner, speed control, Wi-Fi.
-
Customer class: holds first name, last name and id number as member variables (of course with necessary constructor, getters and setters) .
-
Rent class (representing the time period when the car is rent): holds startDate and endDate, rent price (of course with necessary constructor, getters and setters).
In addition to the previous classes, Implement the following interface:
-
rentInterface – holds a method displayRent(). The Rent Class implements this interface.
-
Override the__toString() method for each class (of all previous classes) to print information about the object from the class in which it is instantiated. For example, suppose you have an object called someCar instantiated from small car class with values: "Opel", "20/9/2021", 12548754 (these values inherited from the car class), and the other contact values in the small car class. The toString() method in this case prints (for example): "The car type is Opel, the license end date is:20/9/2021 and the car number is:12548754. This car has 4 passengers size, Motor power of 1200 hp and equipped with an air-conditioner". This statement will be returned from the method toString().
- Write a class rentingManager with a static method rentCar($car, $rent) that handles car renting and prints a message whether the operation was successful or not. This method takes two objects (car and rent) and adds them to an array of rents. This array contains the rents for each car. Of course, there must be no conflict in the dates of rents.
Now, after writing the code of the previous classes and interface, create an array of different cars. Perform the following operations:
-
Create an array of cars, in which the array contains different objects of the three types of cars (create the objects of the array and fill the objects with suitable data).
-
Display the array by small cars and medium ones with a rent price less than or equal to 250.00
-
Display the array by all cars with an air conditioner.
-
Return the car numbers of all cars which have a wi-fi service.

Step by step
Solved in 2 steps

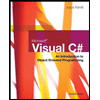
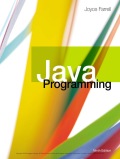
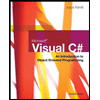
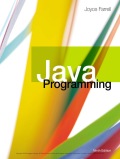