* A class to represent a triangle defined by the x- and y-coorindates of its * three corner points */ public class Triangle { // ADD YOUR INSTANCE VARIABLES HERE /** * Constructs a triangle with the given corner points * @param x1 x-value of corner point 1 @param y1 y-value of corner point 1 * @param x2 x-value of corner point 2 * @param y2 y-value of corner point 2 * @param x3 x-value of corner point 3 * @param y3 y-value of corner point 3 */ public Triangle(double x1, double y1, double x2, double y2, double x3, double y3) { // FILL IN /** * Returns the length of side 1: (x1, y1) to (x2, y2) @return the length of side 1 public double getSide1length() { return -1.0; // FIX ME } /** * Returns the length of side 2: (x1, y1) to (x3, y3) * @return the length of side 2 */ public double getside2Length() { return -1.0; // FIX ME /** * Returns the length of side 3: (x2, y2) to (x3, y3) * @return the length of side 3 */ public double getSide3Length() { return -1.0; // FIX ME } /** * Returns the angle at corner 1 (x1, y1) * @return the angle at corner 1 */ public double getAngle1() { return -1.0; // FIX ME /** * Returns the angle at corner 2 (x2, y2) * @return the angle at corner 2 */ public double getAngle2() { return -1.0; // FIX ME /** * Returns the angle at corner 3 (x3, y3) * @return the angle at corner 3 */ public double getAngle3() { return -1.0; // FIX ME } /** * Returns the perimeter of the triangle * @return the perimeter of the triangle */ public double getPerimeter() { return -1.0; // FIX ME /** * Returns the area of the triangle * @return the area of the triangle public double getArea() { return -1.0; // FIX ME }
* A class to represent a triangle defined by the x- and y-coorindates of its * three corner points */ public class Triangle { // ADD YOUR INSTANCE VARIABLES HERE /** * Constructs a triangle with the given corner points * @param x1 x-value of corner point 1 @param y1 y-value of corner point 1 * @param x2 x-value of corner point 2 * @param y2 y-value of corner point 2 * @param x3 x-value of corner point 3 * @param y3 y-value of corner point 3 */ public Triangle(double x1, double y1, double x2, double y2, double x3, double y3) { // FILL IN /** * Returns the length of side 1: (x1, y1) to (x2, y2) @return the length of side 1 public double getSide1length() { return -1.0; // FIX ME } /** * Returns the length of side 2: (x1, y1) to (x3, y3) * @return the length of side 2 */ public double getside2Length() { return -1.0; // FIX ME /** * Returns the length of side 3: (x2, y2) to (x3, y3) * @return the length of side 3 */ public double getSide3Length() { return -1.0; // FIX ME } /** * Returns the angle at corner 1 (x1, y1) * @return the angle at corner 1 */ public double getAngle1() { return -1.0; // FIX ME /** * Returns the angle at corner 2 (x2, y2) * @return the angle at corner 2 */ public double getAngle2() { return -1.0; // FIX ME /** * Returns the angle at corner 3 (x3, y3) * @return the angle at corner 3 */ public double getAngle3() { return -1.0; // FIX ME } /** * Returns the perimeter of the triangle * @return the perimeter of the triangle */ public double getPerimeter() { return -1.0; // FIX ME /** * Returns the area of the triangle * @return the area of the triangle public double getArea() { return -1.0; // FIX ME }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 10PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Java. Please use the template, thank you!
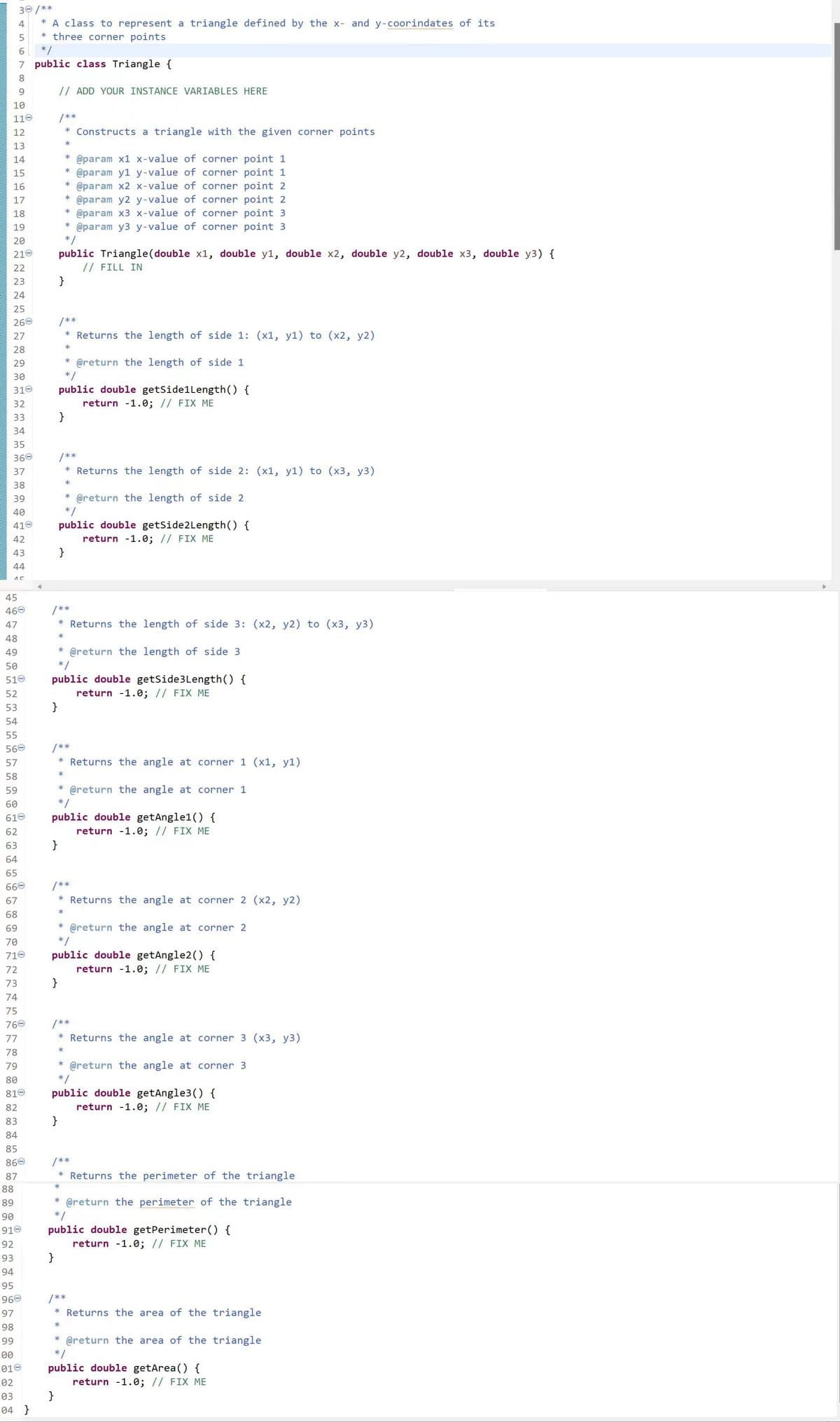
Transcribed Image Text:30 /**
* A class to represent a triangle defined by the x- and y-coorindates of its
* three corner points
4
7 public class Triangle {
8
9.
// ADD YOUR INSTANCE VARIABLES HERE
10
110
/**
12
* Constructs a triangle with the given corner points
*
13
* @param x1 x-value of corner point 1
* @param y1 y-value of corner point 1
* @param x2 x-value of corner point 2
* @param y2 y-value of corner point 2
* @param x3 x-value of corner point 3
* @param y3 y-value of corner point 3
*/
14
15
16
17
18
19
20
public Triangle(double x1, double y1, double x2, double y2, double x3, double y3) {
// FILL IN
}
210
22
23
24
25
260
/**
27
* Returns the length of side 1: (x1, y1) to (x2, y2)
*
28
@return the length of side 1
*/
*
29
30
public double getSide1Length() {
return -1.0; // FIX ME
}
310
32
33
34
35
**
360
37
* Returns the length of side 2: (x1, y1) to (x3, y3)
*
38
* @return the length of side 2
*/
39
40
public double getSide2Length() {
return -1.0; // FIX ME
410
42
43
}
44
45
460
/**
47
* Returns the length of side 3: (x2, y2) to (x3, y3)
48
@return the length of side 3
*/
public double getSide3Length() {
return -1.0; // FIX ME
}
49
50
510
52
53
54
55
560
/**
57
* Returns the angle at corner 1 (x1, y1)
58
* @return the angle at corner 1
*/
59
60
public double getAngle1() {
return -1.0; // FIX ME
}
610
62
63
64
65
/**
* Returns the angle at corner 2 (x2, y2)
660
67
68
*
@return the angle at corner 2
*/
69
70
public double getAngle2() {
return -1.0; // FIX ME
}
710
72
73
74
75
760
/**
77
* Returns the angle at corner 3 (x3, y3)
78
*
@return the angle at corner 3
* /
79
80
public double getAngle3() {
return -1.0; // FIX ME
}
810
82
83
84
85
860
/**
87
* Returns the perimeter of the triangle
88
@return the perimeter of the triangle
*/
89
90
public double getPerimeter() {
return -1.0; // FIX ME
}
910
92
93
94
95
960
/**
97
Returns the area of the triangle
*
98
*
@return the area of the triangle
*/
99
L00
L010
public double getArea() {
return -1.0; // FIX ME
}
L02
L03
L04 }
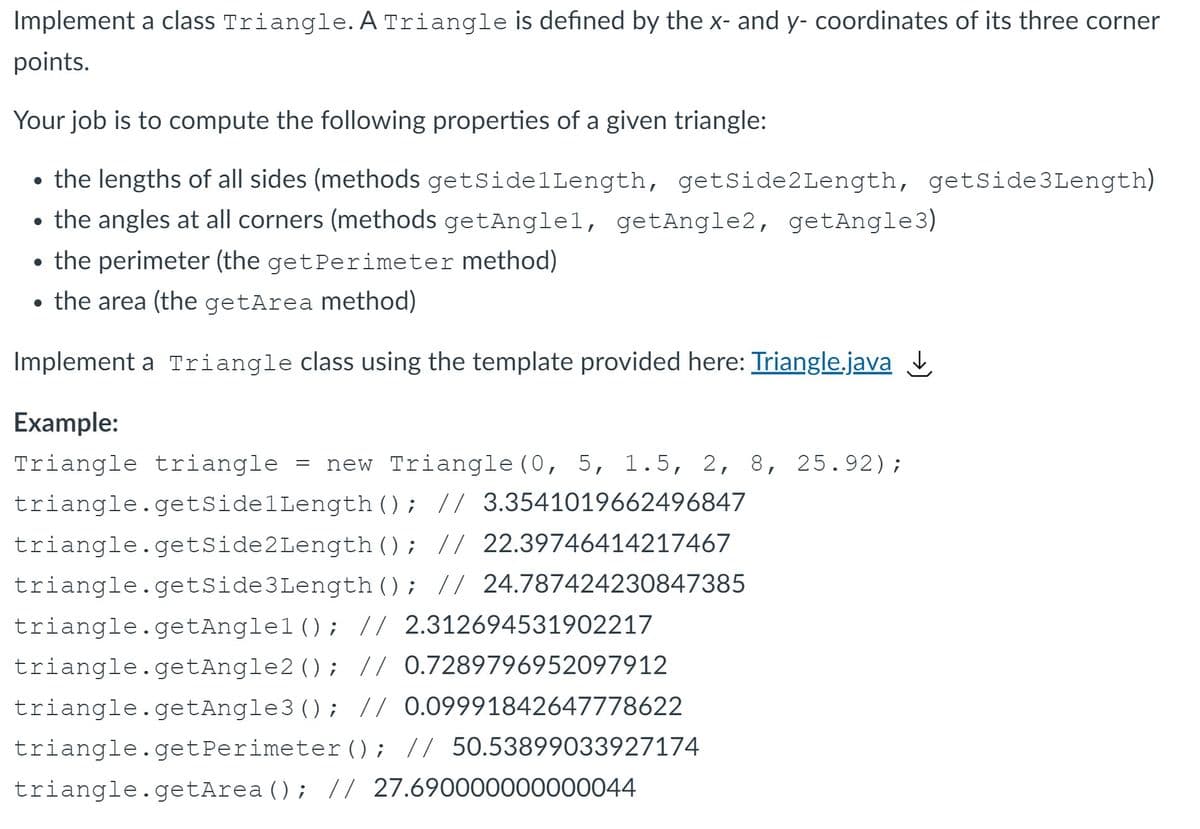
Transcribed Image Text:Implement a class Triangle. A Triangle is defined by the x- and y- coordinates of its three corner
points.
Your job is to compute the following properties of a given triangle:
the lengths of all sides (methods getSidelLength, getSide2Length, getSide3Length)
• the angles at all corners (methods getAnglel, getAngle2, getAngle3)
• the perimeter (the getPerimeter method)
• the area (the getArea method)
Implement a Triangle class using the template provided here: Triangle.java
Example:
Triangle triangle = new Triangle (0, 5, 1.5, 2, 8, 25.92);
triangle.getSidelLength(); // 3.3541019662496847
triangle.getSide2Length (); // 22.39746414217467
triangle.getSide3Length (); // 24.787424230847385
triangle.getAnglel (); // 2.312694531902217
triangle.getAngle2 (); // 0.7289796952097912
triangle.getAngle3 (); // 0.09991842647778622
triangle.getPerimeter(); // 50.53899033927174
triangle.getArea (); // 27.690000000000044
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
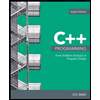
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
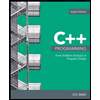
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning