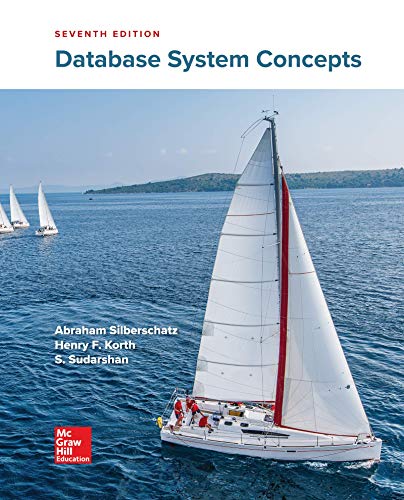
Python question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks.
Write a function diceprob() that takes a possible result r of a roll of pair of dice (i.e. an
integer between 2 and 12) and simulates repeated rolls of a pair of dice until 100 rolls of r
have been obtained. Your function should print how many rolls it took to obtain 100 rolls
of r.
>>> diceprob(2)
It took 4007 rolls to get 100 rolls of 2
>>> diceprob(3)
It took 1762 rolls to get 100 rolls of 3
>>> diceprob(4)
It took 1058 rolls to get 100 rolls of 4
>>> diceprob(5)
It took 1075 rolls to get 100 rolls of 5
>>> diceprob(6)
It took 760 rolls to get 100 rolls of 6
>>> diceprob(7)
It took 560 rolls to get 100 rolls of 7

Step by stepSolved in 3 steps with 1 images

why isn't the code getting the same results that are in the question above
why isn't the code getting the same results that are in the question above
- design the program to hold 30 employees (therefore 30 member array of struct). The data file may have more than 30 employee records however. In that case your program must read only 30 records and give a message that number of records exceeded the program capacity. If data file is empty, then program must print the message to inform user that data file is empty. Modularize your program with functions as much as you can. Your program output must be of the following format. check this Job Type Management Gross Salary $2205.00 Name ID# Jack Smith 1234 number for аccuracy For employees, for which the data are bad, the program must print the message to the affect that Gross Salary could not be calculated due to bad data. The types of bad data may be: Employee code in lower case letter Number of years of service exceeding 50 years Educational and job classification code not in the correct range. The output must be printed to a file. The output file must have processed data printed in the…arrow_forwardComplete the following Codearrow_forwardExercise 6A Write a function Estimate SuperEllipse (m,n) which samples n points randomly in the square, tests whether each lies inside the superellipse, and returns an estimate of the superellipse's area. You can use the command random.uniform(-1, 1) to generate random numbers between -1 and 1. (For technical reasons to assist in marking, please leave the first line of your function as random.seed (1234).)arrow_forward
- use c code to develop the condition and function for the following items and then redevelop the code by adding the function as shown below, the original code has been given below. Hint: a)the function need to be made and develop and than add to the code 1. print Reverse 2. void reverseIt 3. void search Array 4.void search array 5. void array copy and void arrayDiff) b) the answer must be include and output and c code void printReverse(int array[], size); //prints the array reverselyvoid reverseIt(int array[], size); //reverses an arrayvoid searchArray(int array[], int size, int num);// returns the first index of the array whose value is num.//returns -1 if the number was not found in the array// optional for lab 6:void searchnArray(int array[], int size, int num, int n);// returns the nth index of the array whose value is num.//returns -1 if the number was not found in the arrayvoid arrayCopy (int arDest[], int arSource[], int commonSize);// copies cells from arSourc to arDestvoid…arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments through the code, and test the given examples below. Thanks. Write a function called qsolver() that solves a quadratic equation: ax2+bx+c=0. Yourfunction will take three input arguments, a, b, and c, first compute the discriminant d =b2 - 4*a*c. If d < 0, the equation has no real roots. If d == 0 then the equation has one realroot equal to -b / (2*a). If d > 0, the equation has two roots: (-b - math.sqrt(d)) / (2*a) and(-b+math.sqrt(d))/(2*a). Your program should solve the equation given by coefficients a,b, and c, and then return string "No roots", or value ____ , or tuple (____, ____).>>> qsolver(1,2,1)-1.0>>> qsolver(1,0,-1)(1.0, -1.0)>>> qsolver(1,0,1)'No roots'arrow_forwardFill in the Blank Word GameThis exercise involves creating a fill in the blank word game. Try prompting the user to enter a noun,verb, and an adjective. Use the provided responses to fill in the blanks and then display the story.First, write a short story. Remove a noun, verb, and an adjective.Create a function that asks for input from the user.Create another function that will fill the blanks in the story you’ve just created.Ensure that each function contains a docstring.After the noun, verb, and adjective have been collected from the user, display the story that has beencreated using their input. use python to ode.arrow_forward
- *DONOT USE CHATGPT, please put comments so i can understand. It’s a C language program with algorithm.arrow_forwardplaese code in python You are tasked with creating a square grid of values in which each value only appears once in each column and once in each row (think like a Sudoku). Write a function called grid that inputs a list and outputs a square grid of values of that list in which each value only appears once in each column and once in each row. Specifically, one in which each row is the one above it shifted by one item. Your answer should be in the form of a list of lists, where each sub-list is a row of the square. For example: grid([1, 2, 3, 4]) -> [[1, 2, 3, 4], [4, 1, 2, 3], [3, 4, 1, 2], [2, 3, 4, 1]]arrow_forwardCreate a function named fnTuition that calculates the tuition for a student. This function accepts one parameter, the student ID, and it calls the fnStudentUnits function that you created in task 2. The tuition value for the student calculated according to the following pseudocode: if (student does not exist) or (student units = 0) tuition = 0 else if (student units >= 9) tuition = (full time cost) + (student units) * (per unit cost) else tuition = (part time cost) + (student units) * (per unit cost) Retrieve values of FullTimeCost, PartTimeCost, and PerUnitCost from table Tuition. If there is no student with the ID passed to the function, the function should return -1. Code two tests: 1) a student who has < 9 student units, and 2) for a student who has >= 9 student units. For each test, display StudentID and the result returned by the function. Also, run supportive SELECT query or queries that prove the results to be correct.arrow_forward
- The food pantry provides its volunteers with keys to unlock doors and a Food Pantry vest to let customers know they work there. There are a limited amount of keys and vests, so volunteers need to check them out of the office.Complete the program by creating a CheckoutEquipment function that takes in the number of keys and vests currently available at the office. If there are enough keys and vests, the function should reduce the number of keys and vests by 1 and return true. Otherwise, the number of keys and vests will stay the same, and the function will return false. Take note that the number of keys and vests will be defined in the main function and the CheckoutEquipment function should modify the values of those variables declared in main. #include <iostream> int main() { int keys = 10; int vests = 10; bool checked_out = CheckoutEquipment(keys, vests); if (checked_out) { std::cout << "Successfully checked out.\n"; } else { std::cout << "Not…arrow_forwardCreate a function that determines whether each seat can "see" the front-stage. A number can "see" the front-stage if it is strictly greater than the number before it. Everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 5, 4, 4], [6, 6, 7, 6, 5, 5]] # Starting from the left, the 6 > 5 > 2 > 1, so all numbe # 6 > 5 > 4 > 2 - so all numbers can see, etc. Not everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 10, 4, 4], [6, 6, 7, 6, 5, 5]] # The 10 is directly in front of the 6 and blocking its varrow_forwardPlease complete the exercise as instructed and no not use global variables. Thank you!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
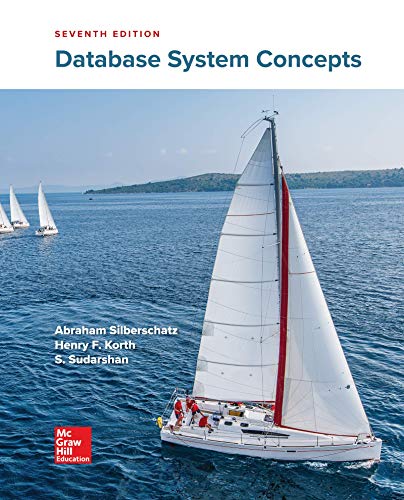
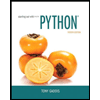
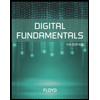
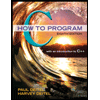
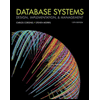
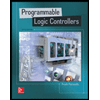