Create a new project named lab7_1. You will be implementing a Tacos class. For the class attributes, let’s use to type of taco, number of tacos, and price. Note that the price per taco of any type is 99 cents. Therefore, allow the taco type and number of tacos to be set directly, but not the price! The price should be set automatically based on the number of tacos entered. So a good idea may be to call the setter for the price inside the function that sets the number of tacos. Some other requirements: A default constructor and a constructor with parameters for your taco type and number of tacos member variables. You should also include setters and getters for these private member variables. However, make sure that the setter for price is private! You don’t want anyone outside the class modifying price. This setter should be called anytime you change the number of tacos. Your constructor needs to call a set method that allows you to set the two member variables, thus takes two arguments. In turn, the set method will call the individual setters for each member variable. The number of tacos setter needs to throw an exception if a valid number for tacos is not used (only positive numbers should work). This means that back in your main, you will also have a try...catch block to catch this exception. Don’t forget to include the stdexcept library. Use a general run-time exception like shown before: if(some condition) do some stuff; else throw runtime_error("Error: No negative tacos!"); Which means the catch should be catching a runtime_error object from the main. You will also make use of the const keyword to indicate which methods are not modifying the object. Demonstrate the use of your Tacos class by creating objects of your class type, setting them to different values, and then printing out their information. Make sure to also show an example of an incorrect value being assigned to a member variable, and the program throwing an exception. Potential sample run: #include #include "Tacos.h" int main() { Tacos t1, t2, t3; t1.setTacos("carnitas", 1); t2.setTacos("asada", 3); t3.setTacos("pastor", 2); t1.print(); t2.print(); try { t2.setNumber(-4); } catch (runtime_error & e) { cout << "Error: " << e.what() << endl; } t3.print(); return 0; } 1 carnitas taco costs $0.99. 3 asada tacos costs $2.97. Error: No negative tacos! 2 pastor tacos costs $1.98.
Create a new project named lab7_1. You will be implementing a Tacos class. For the class attributes, let’s use to type of taco, number of tacos, and price. Note that the price per taco of any type is 99 cents. Therefore, allow the taco type and number of tacos to be set directly, but not the price! The price should be set automatically based on the number of tacos entered. So a good idea may be to call the setter for the price inside the function that sets the number of tacos. Some other requirements: A default constructor and a constructor with parameters for your taco type and number of tacos member variables. You should also include setters and getters for these private member variables. However, make sure that the setter for price is private! You don’t want anyone outside the class modifying price. This setter should be called anytime you change the number of tacos. Your constructor needs to call a set method that allows you to set the two member variables, thus takes two arguments. In turn, the set method will call the individual setters for each member variable. The number of tacos setter needs to throw an exception if a valid number for tacos is not used (only positive numbers should work). This means that back in your main, you will also have a try...catch block to catch this exception. Don’t forget to include the stdexcept library. Use a general run-time exception like shown before: if(some condition) do some stuff; else throw runtime_error("Error: No negative tacos!"); Which means the catch should be catching a runtime_error object from the main. You will also make use of the const keyword to indicate which methods are not modifying the object. Demonstrate the use of your Tacos class by creating objects of your class type, setting them to different values, and then printing out their information. Make sure to also show an example of an incorrect value being assigned to a member variable, and the program throwing an exception. Potential sample run: #include #include "Tacos.h" int main() { Tacos t1, t2, t3; t1.setTacos("carnitas", 1); t2.setTacos("asada", 3); t3.setTacos("pastor", 2); t1.print(); t2.print(); try { t2.setNumber(-4); } catch (runtime_error & e) { cout << "Error: " << e.what() << endl; } t3.print(); return 0; } 1 carnitas taco costs $0.99. 3 asada tacos costs $2.97. Error: No negative tacos! 2 pastor tacos costs $1.98.
Chapter12: Using Controls
Section: Chapter Questions
Problem 12E
Related questions
Question
Create a new project named lab7_1. You will be implementing a Tacos class. For the class attributes, let’s use to type of taco, number of tacos, and price. Note that the price per taco of any type is 99 cents. Therefore, allow the taco type and number of tacos to be set directly, but not the price! The price should be set automatically based on the number of tacos entered. So a good idea may be to call the setter for the price inside the function that sets the number of tacos. Some other requirements:
A default constructor and a constructor with parameters for your taco type and number of tacos member variables. You should also include setters and getters for these private member variables. However, make sure that the setter for price is private! You don’t want anyone outside the class modifying price. This setter should be called anytime you change the number of tacos.
Your constructor needs to call a set method that allows you to set the two member variables, thus takes two arguments. In turn, the set method will call the individual setters for each member variable.
The number of tacos setter needs to throw an exception if a valid number for tacos is not used (only positive numbers should work). This means that back in your main, you will also have a try...catch block to catch this exception. Don’t forget to include the stdexcept library. Use a general run-time exception like shown before:
if(some condition)
do some stuff;
else
throw runtime_error("Error: No negative tacos!");
Which means the catch should be catching a runtime_error object from the main.
You will also make use of the const keyword to indicate which methods are not modifying the object.
Demonstrate the use of your Tacos class by creating objects of your class type, setting them to different values, and then printing out their information. Make sure to also show an example of an incorrect value being assigned to a member variable, and the program throwing an exception.
Potential sample run:
#include
#include "Tacos.h"
int main()
{
Tacos t1, t2, t3;
t1.setTacos("carnitas", 1);
t2.setTacos("asada", 3);
t3.setTacos("pastor", 2);
t1.print();
t2.print();
try
{
t2.setNumber(-4);
}
catch (runtime_error & e)
{
cout << "Error: " << e.what() << endl;
}
t3.print();
return 0;
}
1 carnitas taco costs $0.99.
3 asada tacos costs $2.97.
Error: No negative tacos!
2 pastor tacos costs $1.98.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
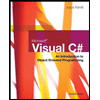
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
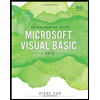
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
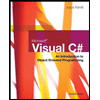
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
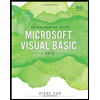
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning