Create a program for Smalltown Regional Airport Flights that accepts either an integer flight number or string airport code from the options in Figure 8-33. Pass the user’s entry to one of two overloaded GetFlightInfo() methods, and then display a returned string with all the flight details. For example, if 201 was input, the output would be: Flight #201 AUS Austin Scheduled at: 0710 (note that there should be two spaces between 'Austin' and 'Scheduled'). The method version that accepts an integer looks up the airport code, name, and time of flight; the version that accepts a string description looks up the flight number, airport name, and time. The methods return a message if the flight is not found. For example, if 100 was input, the output should be Flight #100 was not found. If no flights were scheduled for the airport code entered, for example MCO, the message displayed should be Flight to MCO was not found. I am having these errors 1- Flights.GetFlightInfo(string, int[], string[], string[], string[])': not all code paths return a value Compilation failed: 1 error(s)". What does that mean and how will I fix it? 2- How to write a code to return a string? 3- What an overloaded method mean, and how to write it?
Microsoft Visual C# 7th edition
Create a program for Smalltown Regional Airport Flights that accepts either an integer flight number or string airport code from the options in Figure 8-33.
Pass the user’s entry to one of two overloaded GetFlightInfo() methods, and then display a returned string with all the flight details. For example, if 201 was input, the output would be: Flight #201 AUS Austin Scheduled at: 0710 (note that there should be two spaces between 'Austin' and 'Scheduled').
The method version that accepts an integer looks up the airport code, name, and time of flight; the version that accepts a string description looks up the flight number, airport name, and time.
The methods return a message if the flight is not found. For example, if 100 was input, the output should be Flight #100 was not found.
If no flights were scheduled for the airport code entered, for example MCO, the message displayed should be Flight to MCO was not found.
I am having these errors
1- Flights.GetFlightInfo(string, int[], string[], string[], string[])': not all code paths return a value
Compilation failed: 1 error(s)". What does that mean and how will I fix it?
2- How to write a code to return a string?
3- What an overloaded method mean, and how to write it? thank you.
Here is my code

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

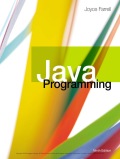
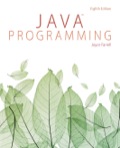
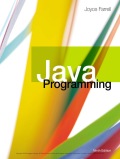
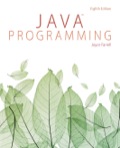