Employee's first name: Kim Employee's last name: Smith Number of shifts: 25 Number of transactions: 75 Transaction dollar value: 40000.00 our output should be: Employee Name: Kim Smith Employee Bonus: $50.0
Employee's first name: Kim Employee's last name: Smith Number of shifts: 25 Number of transactions: 75 Transaction dollar value: 40000.00 our output should be: Employee Name: Kim Smith Employee Bonus: $50.0
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter2: Basic Elements Of C++
Section: Chapter Questions
Problem 5PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
// EmployeeBonus.cpp - This program calculates an employee's yearly bonus.
#include <iostream>
#include <string>
using namespace std;
int main()
{
// Declare and initialize variables here
string employeeFirstName;
string employeeLastName;
double numTransactions;
double numShifts;
double dollarValue;
double score;
double bonus;
const double BONUS_1 = 50.00;
const double BONUS_2 = 75.00;
const double BONUS_3 = 100.00;
const double BONUS_4 = 200.00;
// This is the work done in the housekeeping() function
cout << "Enter employee's first name: Kim";
cin >> employeeFirstName;
cout << "Enter employee's last name: Smith";
cin >> employeeLastName;
cout << "Enter number of shifts: 25";
cin >> numShifts;
cout << "Enter number of transactions: 75";
cin >> numTransactions;
cout << "Enter dollar value of transactions: 40000.00";
cin >> dollarValue;
// This is the work done in the detailLoop()function
// Write your code here
if
// This is the work done in the endOfJob() function
// Output.
cout << "Employee Name: Kim Smith" << employeeFirstName << " " << employeeLastName << endl;
cout << "Employee Bonus: $50.00" << bonus << endl;
return 0;
}
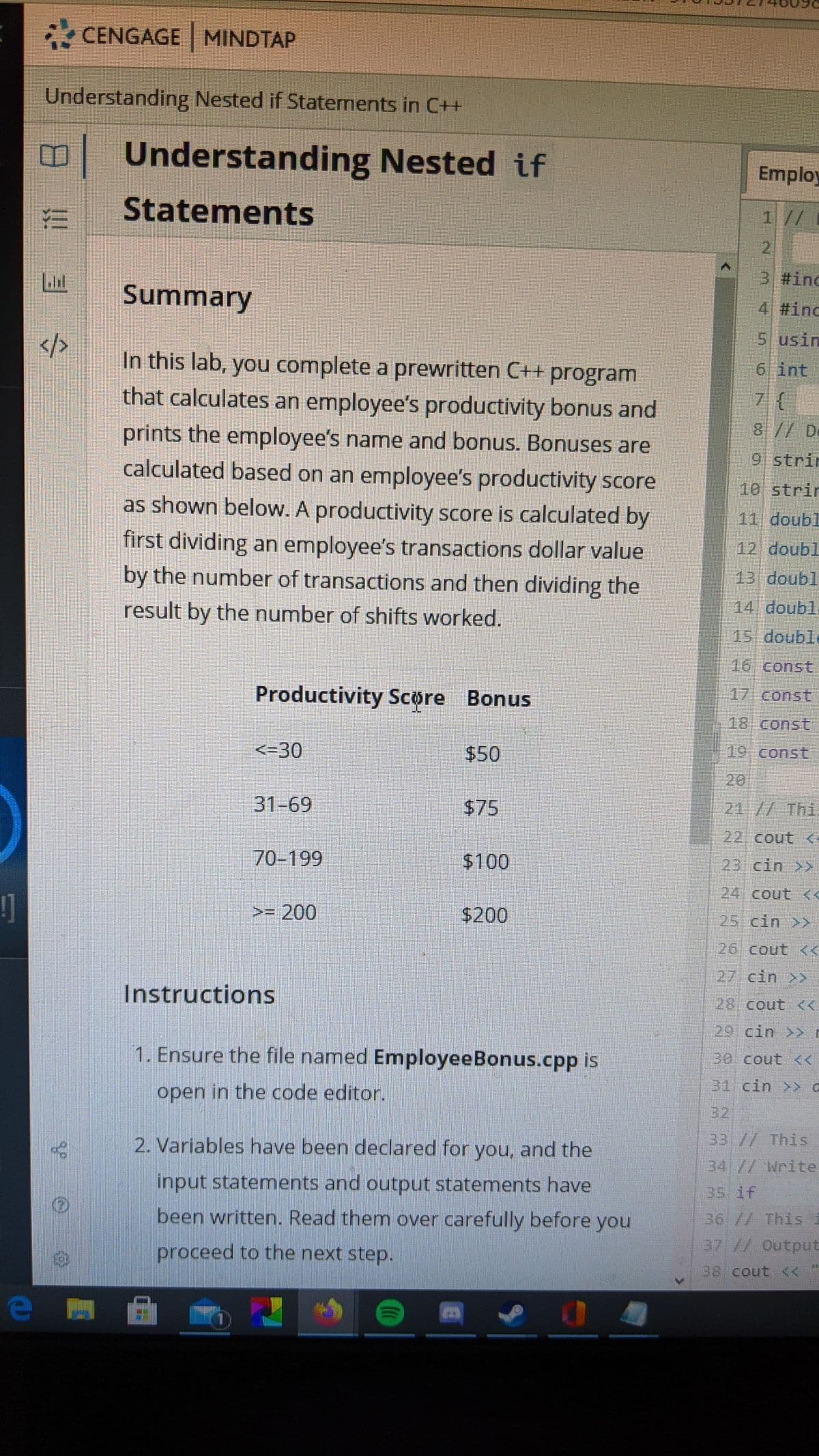
Transcribed Image Text:* CENGAGE MINDTAP
Understanding Nested if Statements in C++
Understanding Nested if
Employ
Statements
1 //
2.
3 #inc
Summary
4 #inc
5 usin
In this lab, you complete a prewritten C++ program
that calculates an employee's productivity bonus and
6 int
8// De
prints the employee's name and bonus. Bonuses are
calculated based on an employee's productivity score
9 strir
10 strir
as shown below. A productivity score is calculated by
11 doubl
first dividing an employee's transactions dollar value
by the number of transactions and then dividing the
12 doubl
13 doubl
result by the number of shifts worked.
14 doubl
15 double
16 const
Productivity Scøre Bonus
17 const
18. const
<-30
$50
19 const
20
31-69
$75
21 // Thi
22 cout <
70-199
$100
23 cin >
24 cout <<
1
>= 200
$200
25 cin >
26 cout <
27 cin >>
Instructions
28 cout <<
29 cin >> r
1. Ensure the file named EmployeeBonus.cpp is
30 cout <
31 cin >> a
open in the code editor.
32
2. Variables have been declared for you, and the
33 // This
34 // Write
input statements and output statements have
35 if
been written. Read them over carefully before you
36 // Thisi
proceed to the next step.
37 // Output
38 cout <
7.
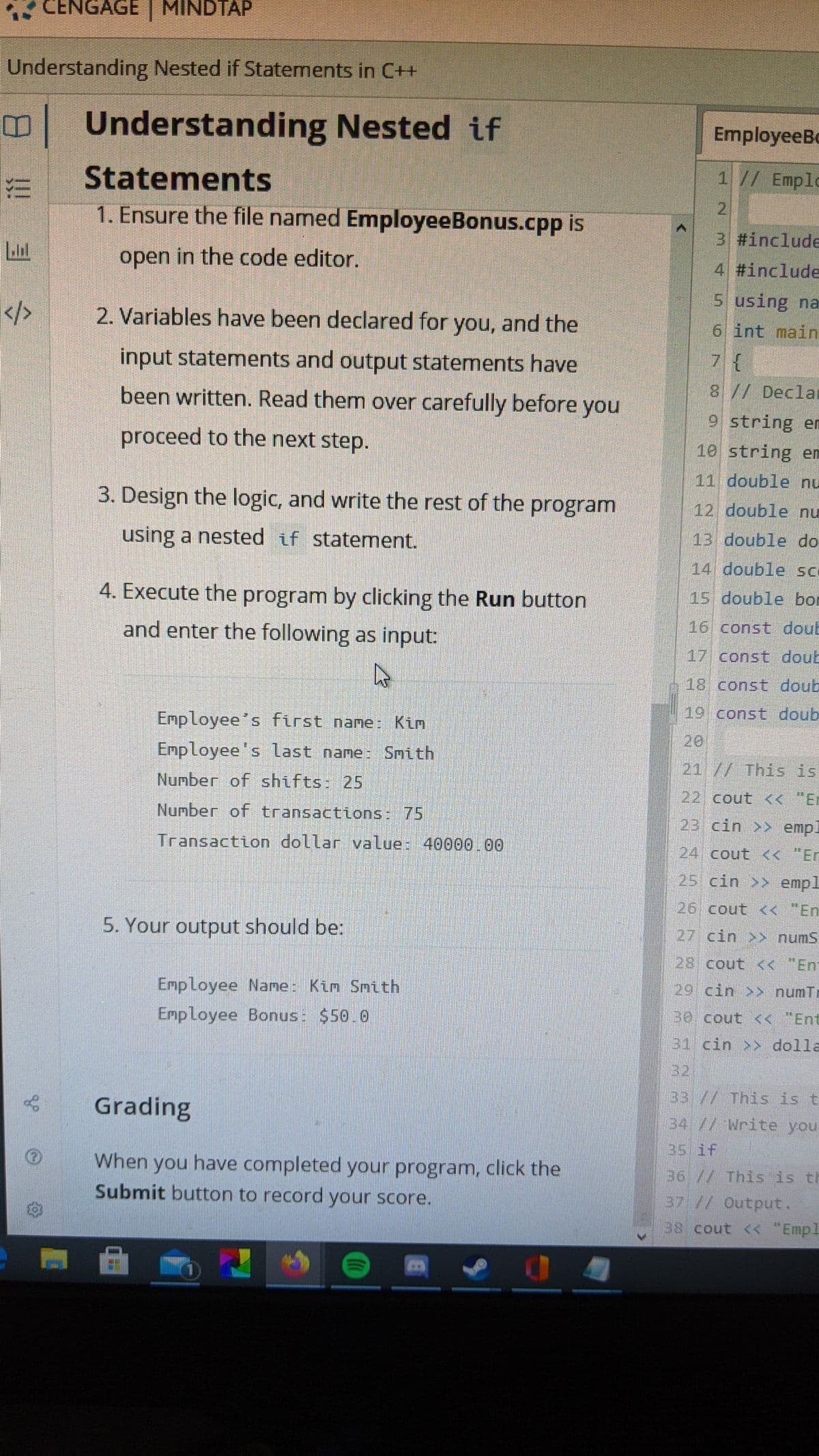
Transcribed Image Text:CENGAGE MINDTAP
Understanding Nested if Statements in C++
Understanding Nested if
EmployeeBo
1 // Emple
21
Statements
1. Ensure the file named EmployeeBonus.cpp is
3 #include
open in the code editor.
4 #include
5 using na
2. Variables have been declared for you, and the
6 int main
input statements and output statements have
7{
8// Declal
been written. Read them over carefully before you
9 string en
proceed to the next step.
10 string em
11 double nu
3. Design the logic, and write the rest of the program
12 double nu
13 double do
using a nested if statement.
14 double sc
4. Execute the program by clicking the Run button
15 double bor
16 const doub
and enter the following as input:
17 const doub
18 const doub
19 const doub
EmpLoyee's first name: Kim
20
Employee's last name: Smith
21//This is
Number of shifts: 25
22 cout < "Er
Number of transactions: 75
23 cin >> emp.
Transaction dollar value: 40000.00
24 cout << "Er
25 cin >> empl
26 cout << "En
5. Your output should be:
27 cin >> numS
28 cout << "En
Employee Name: Kim Snth
29 cin >> numTr
30 cout << "Ent
Employee Bonus: $50.0
31 cin >> dolla
32
33// This is t
Grading
34 // Write you
35 if
When you have completed your program, click the
Submit button to record your score.
36 // This is th
37 // Output.
38 cout < "Empl
!!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
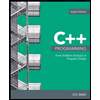
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
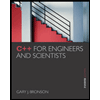
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
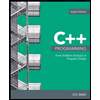
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
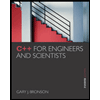
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr