Create a server class called Course and a class called Student Class Student a string called studentid a string called firstName a string called lastName the Student class will have a 3 parameter constructor with parameter for id, first name and last name of the student. it will also contain getter methods for all instance varibales and a toString method that returns the id, first name and last name Class Course a string called courseName an array of Student objects called studentArray an int called numberOfStudents A Course object: can be created using a 1 paramter constructor(the name of the course) you can add students to the course using an addStudent method which has 1 paramter consisting of a new Student object. whenever a new student is added to the course, numberOfStudents is increased you can drop a student(remove a student from the array of Students) from the course usinf a dropStudent method which has 1 parameter consisting of a String representing the id of the Student to be dropped The Course class: uses an array to store the students for the course. assume that the course enrollment is initially 5 the Course class will also contain a mthod called increaseSize, that will automatically increase the array size by creating a new larger array and copying the contents of the current array to it it will also contain a mehtod called getStudents that returns an array consisting only of those students who have been added to the course Create a method called clear, that removes all students from the course (you can do that by setting all references in the student array to "null") Class CourseDemo is given below that instantiates both class Student and class Course public class CourseDemo { public static void main(String[] args) { Course course1 = new Course("Data Structures"); Course course2 = new Course("Database Systems"); course1.addStudent(new Student("121", "Peter", "Jones")); course1.addStudent(new Student("122", "Brian", "Smith")); course1.addStudent(new Student("123", "Anne", "Kennedy")); course1.addStudent(new Student("124", "Susan", "Kennedy")); course1.addStudent(new Student("125", "Carlos", "Lopez")); course1.addStudent(new Student("126", "Michael", "Johnson")); course2.addStudent(new Student("121", "Peter", "Jones")); course2.addStudent(new Student("125", "Carlos", "Lopez")); System.out.println("Number of students in course1: " + course1.getNumberOfStudents()); Student[] students = course1.getStudents(); for (int i = 0; i < students.length; i++) System.out.println(students[i]); System.out.println(); System.out.println("Number of students in course2: " + course2.getNumberOfStudents()); course1.dropStudent("123"); System.out.println("Dropped student 123"); System.out.println("After drop: Number of students in course1: " + course1.getNumberOfStudents()); students = course1.getStudents(); for (int i = 0; i < course1.getNumberOfStudents(); i++) System.out.println(students[i] ); //(i < course1.getNumberOfStudents() - 1 ? ", " : " ")); course1.clear(); System.out.println("\nAfter clear: Number of students in course1: " + course1.getNumberOfStudents()); } }
Create a server class called Course and a class called Student
Class Student
- a string called studentid
- a string called firstName
- a string called lastName
the Student class will have a 3 parameter constructor with parameter for id, first name and last name of the student. it will also contain getter methods for all instance varibales and a toString method that returns the id, first name and last name
Class Course
- a string called courseName
- an array of Student objects called studentArray
- an int called numberOfStudents
A Course object:
- can be created using a 1 paramter constructor(the name of the course)
- you can add students to the course using an addStudent method which has 1 paramter consisting of a new Student object. whenever a new student is added to the course, numberOfStudents is increased
- you can drop a student(remove a student from the array of Students) from the course usinf a dropStudent method which has 1 parameter consisting of a String representing the id of the Student to be dropped
The Course class:
- uses an array to store the students for the course. assume that the course enrollment is initially 5
- the Course class will also contain a mthod called increaseSize, that will automatically increase the array size by creating a new larger array and copying the contents of the current array to it
- it will also contain a mehtod called getStudents that returns an array consisting only of those students who have been added to the course
- Create a method called clear, that removes all students from the course (you can do that by setting all references in the student array to "null")
Class CourseDemo is given below that instantiates both class Student and class Course
public class CourseDemo {
public static void main(String[] args) {
Course course1 = new Course("Data Structures");
Course course2 = new Course("
course1.addStudent(new Student("121", "Peter", "Jones"));
course1.addStudent(new Student("122", "Brian", "Smith"));
course1.addStudent(new Student("123", "Anne", "Kennedy"));
course1.addStudent(new Student("124", "Susan", "Kennedy"));
course1.addStudent(new Student("125", "Carlos", "Lopez"));
course1.addStudent(new Student("126", "Michael", "Johnson"));
course2.addStudent(new Student("121", "Peter", "Jones"));
course2.addStudent(new Student("125", "Carlos", "Lopez"));
System.out.println("Number of students in course1: "
+ course1.getNumberOfStudents());
Student[] students = course1.getStudents();
for (int i = 0; i < students.length; i++)
System.out.println(students[i]);
System.out.println();
System.out.println("Number of students in course2: "
+ course2.getNumberOfStudents());
course1.dropStudent("123");
System.out.println("Dropped student 123");
System.out.println("After drop: Number of students in course1: "
+ course1.getNumberOfStudents());
students = course1.getStudents();
for (int i = 0; i < course1.getNumberOfStudents(); i++)
System.out.println(students[i] );
//(i < course1.getNumberOfStudents() - 1 ? ", " : " "));
course1.clear();
System.out.println("\nAfter clear: Number of students in course1: "
+ course1.getNumberOfStudents());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

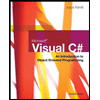
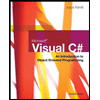