Create the class Suitcase. Suitcase has things and a maximum weight limit, which defines the greatest total allowed weight of the things contained within the Suitcase object. Add the following methods to your class: A constructor, which is given a maximum weight limit public void addThing(Thing thing), which adds the thing in the parameter to your suitcase. The method does not return any value. public String toString(), which returns a string in the form "x things (y kg)" The things are saved into an ArrayList object: ArrayList things = new ArrayList(); The class Suitcase has to make sure the thing's weight does not cause the total weight to exceed the maximum weight limit. The method addThing should not add a new thing if the total weight happens to exceed the maximum weight limit. Below, you find an example of how the class can be used: public class Main { public static void main(String[] args) { Thing book = new Thing("Happiness in three steps", 2); Thing mobile = new Thing("Nokia 3210", 1); Thing brick = new Thing("Brick", 4); Suitcase suitcase = new Suitcase(5); System.out.println(suitcase); suitcase.addThing(book); System.out.println(suitcase); suitcase.addThing(mobile); System.out.println(suitcase); suitcase.addThing(brick); System.out.println(suitcase); } } The program output should look like the following: 0 things (0 kg) 1 things (2 kg) 2 things (3 kg) 2 things (3 kg) This is class Thing public class Thing { privateStringname; privateintweight; publicThing(Stringname,intweight){ this.name=name; this.weight=weight; } publicStringgetName(){ // returns the thing's name returnthis.name; } publicintgetWeight(){ // returns the thing's weight returnthis.weight; } publicStringtoString(){ returnthis.name+" ("+this.weight+" kg)"; } }
Create the class Suitcase. Suitcase has things and a maximum weight limit, which defines the greatest total allowed weight of the things contained within the Suitcase object.
Add the following methods to your class:
- A constructor, which is given a maximum weight limit
- public void addThing(Thing thing), which adds the thing in the parameter to your suitcase. The method does not return any value.
- public String toString(), which returns a string in the form "x things (y kg)"
The things are saved into an ArrayList object:
ArrayList<Thing> things = new ArrayList<Thing>();
The class Suitcase has to make sure the thing's weight does not cause the total weight to exceed the maximum weight limit. The method addThing should not add a new thing if the total weight happens to exceed the maximum weight limit.
Below, you find an example of how the class can be used:
public class Main {
public static void main(String[] args) {
Thing book = new Thing("Happiness in three steps", 2);
Thing mobile = new Thing("Nokia 3210", 1);
Thing brick = new Thing("Brick", 4);
Suitcase suitcase = new Suitcase(5);
System.out.println(suitcase);
suitcase.addThing(book);
System.out.println(suitcase);
suitcase.addThing(mobile);
System.out.println(suitcase);
suitcase.addThing(brick);
System.out.println(suitcase);
}
}
The
0 things (0 kg)
1 things (2 kg)
2 things (3 kg)
2 things (3 kg)
This is class Thing
public class Thing {
privateStringname;
privateintweight;
publicThing(Stringname,intweight){
this.name=name;
this.weight=weight;
}
publicStringgetName(){
// returns the thing's name
returnthis.name;
}
publicintgetWeight(){
// returns the thing's weight
returnthis.weight;
}
publicStringtoString(){
returnthis.name+" ("+this.weight+" kg)";
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

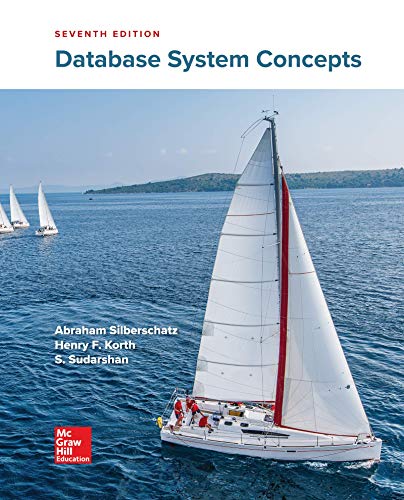
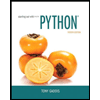
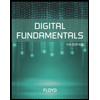
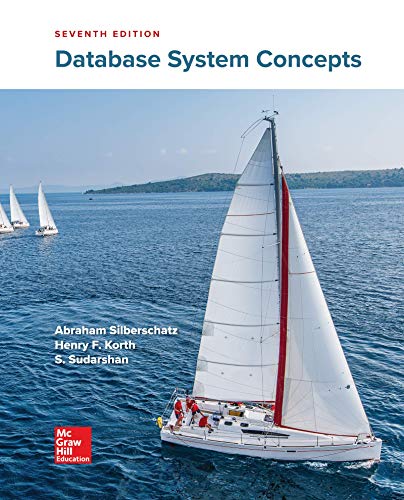
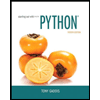
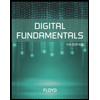
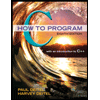
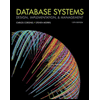
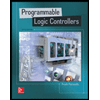