For this assignment you need to write a parallel program in C++ using OpenMP for vector addition. Assume A, B, C are three vectors of equal length. The program will add the corresponding elements of vectors A and B and will store the sum in the corresponding elements in vector C (in other words C[i] = A[i] + B[i]). Every thread should execute approximately equal number of loop iterations. The only OpenMP directive you are allowed to use is: #pragma omp parallel num_threads(no of threads) The program should take n and the number of threads to use as command line arguments: ./parallel_vector_addition Where n is the length of the vectors and threads is the number of threads to be created. Pseudocode for Assignment mystart = myid*n/p; // starting index for the individual thread myend = mystart+n/p; // ending index for the individual thread for (i = mystart; i < myend; i++) // each thread computes local sum do vector addition // and later all local sums combined.
For this assignment you need to write a parallel
As an input vector A, initialize its size to 10,000 and elements from 1 to 10,000.
So, A[0] = 1, A[1] = 2, A[2] = 3, … , A[9999] = 10000.
Input vector B will be initialized to the same size with opposite inputs.
So, B[0] = 10000, B[1] = 9999, B[2] = 9998, … , B[9999] = 1
Using above input vectors A and B, create output Vector C which will be computed as
C[ i ] = A[ i ] + B[ i ];
You should check whether your output vector value is 10001 in every C[ i ].
First, start with 2 threads (each thread adding 5,000 vectors), and then do with 4,and and 8
threads. Remember sometimes your vector size can not be divided equally by number of threads.
You need to slightly modify pseudo code to handle the situation accordingly. (Hint: If you have p
threads, first (p - 1) threads should have equal number of input size and the last thread will take
care of whatever the remainder portion.) Check the running time from each experiment and
compare the result. Report your findings from this project in a separate paragraph.
Your output should show team of treads do evenly distributed work, but big vector size might
cause an issue in output. You can create mini version of original vector in much smaller size of
100 (A[0] = 1, A[1] = 2, A[2] = 3, … , A[99] = 100) and run with 6 threads once and take a snap
shop of your output. And run with original size with 2, 4, and 8 threads to compare running times.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

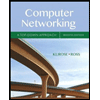
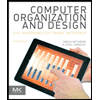
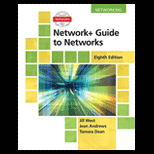
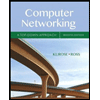
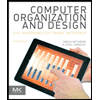
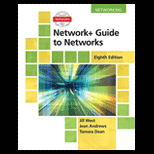
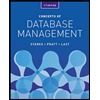
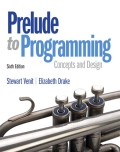
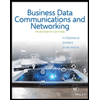